Scroll to Top Using window.scrollY Property
Last Updated :
20 Jun, 2023
A Scroll to top button is used to Scroll to the top of the page whenever we are at the end or middle of the page and we want to reach the top of the page directly. In this article, we are going to make a Scroll To Top Button.
Before starting we need Font Awesome icon for the Up arrow. To get this we will use the font awesome CDN link. The link is given below you can copy and paste it into the head tag inside the HTML file.
CDN Link:
https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.0/css/all.min.css
Functionalities we want:
- Initially, the scroll button should hide.
- Whenever we scroll the current page, in the bottom right corner a button should appear.
- After clicking on the button, the page automatically scrolls to the top of the page.
Here is the preview of the feature we are going to make.
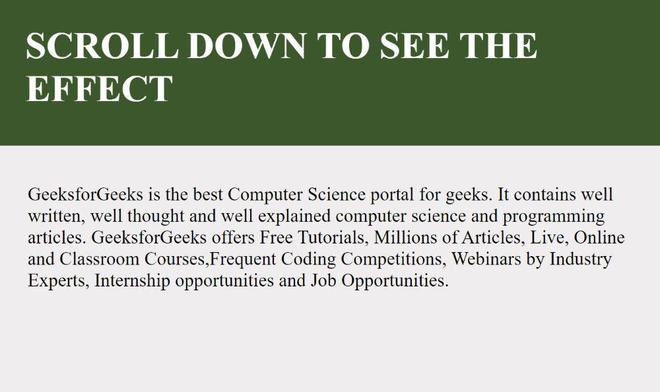
Preview Image
Project Structure
- index.html
- style.css
- script.js
Example: Add all the below code to the respective files you will see the output in the browser.
- HTML Code: We have used a container div and inside that we have used random text and used the ArrowUp icon from the fontawesome CDN link.
- CSS Code: Used normal styling to style the text and attached the ArrowUp icon to the bottom right.
- JavaScript Code: Initially, the ArrowUp will hide and whenever the user scrolls the screen the icon will be visible, and the scrollToTop() function will trigger whenever the icon will be clicked.
Javascript
window.onscroll = function () {
scrollFunction();
};
function scrollFunction() {
if (document.body.scrollTop > 20 || document.documentElement.scrollTop > 20) {
document.getElementById( "scrollToTopBtn" ).style.display = "block" ;
} else {
document.getElementById( "scrollToTopBtn" ).style.display = "none" ;
}
}
function scrollToTop() {
const scrollDuration = 500;
const scrollStep = -window.scrollY / (scrollDuration / 15);
const scrollInterval = setInterval( function () {
if (window.scrollY !== 0) {
window.scrollBy(0, scrollStep);
} else {
clearInterval(scrollInterval);
}
}, 15);
}
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< link rel = "stylesheet" href = "style.css" >
< link rel = "stylesheet"
href =
< title >Document</ title >
</ head >
< body >
< div class = "container" >
< button id = "scrollToTopBtn" onclick = "scrollToTop()" >
< i class = "fa-solid fa-arrow-up fa-beat fa-lg" style = "color: #ffffff;" >
</ i >
</ button >
< div class = "content-div" >
< div class = "heading" >
Scroll Down to see the effect
</ div >
< div class = "description" >
GeeksforGeeks is the best Computer Science portal for geeks.
It contains well written, well thought and well explained
computer science and programming articles.
GeeksforGeeks offers Free Tutorials, Millions of Articles,
Live, Online and Classroom Courses,Frequent Coding Competitions,
Webinars by Industry Experts, Internship opportunities and
Job Opportunities.
</ div >
</ div >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
* {
margin : 0 ;
padding : 0 ;
box-sizing: border-box;
}
body {
background-color : rgb ( 239 , 238 , 238 );
}
.heading {
background-color : rgb ( 51 , 88 , 38 );
color : white ;
padding : 30px ;
font-size : 2 rem;
font-weight : 900 ;
text-transform : uppercase ;
}
#scrollToTopBtn {
position : fixed ;
width : 2.5 rem;
height : 2.5 rem;
bottom : 2 rem;
right : 2 rem;
z-index : 10 ;
font-size : 1 rem;
background-color : rgb ( 255 , 0 , 0 );
border : none ;
cursor : pointer ;
border-radius: 50% ;
opacity: 0.8 ;
transition: opacity 0.3 s, transform 0.3 s;
}
#scrollToTopBtn:hover {
opacity: 1 ;
transform: scale( 1.1 );
}
.description {
height : 2500px ;
margin : 2 rem;
}
|
Output: Click here to see live code output
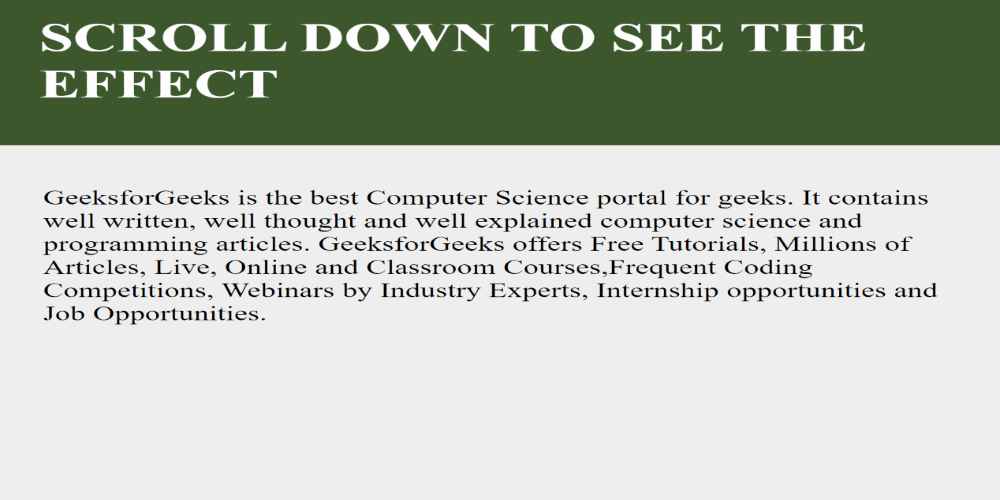
Scroll To Top Button using onscroll Method
Share your thoughts in the comments
Please Login to comment...