Run One Python Script From Another in Python
Last Updated :
08 Feb, 2024
In Python, we can run one file from another using the import statement for integrating functions or modules, exec() function for dynamic code execution, subprocess module for running a script as a separate process, or os.system() function for executing a command to run another Python file within the same process. In this article, we will explore all the specified approaches to make one Python file run another.
Run One Python Script From Another in Python
Below are some of the ways by which we can make one Python file run another in Python:
Make One Python File Run Another Using import Module
In this example, file_2.py imports functions from file_1.py using the import statement. By calling the imported function in file_2.py (file_1.printing()), the code executes the printing() function defined in file_1.py, allowing for the integration and execution of code from one file within another.
Python3
def printing():
print ( "Hello world!" )
|
Python3
import file_1
file_1.printing()
|
Output:
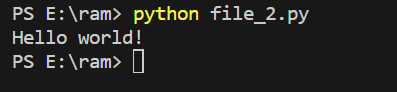
Run One Python Script From Another Using exec() Function
In this example, file_2.py opens and reads the file_1.py, by ‘with‘ statement using the ‘open‘ function it opens file_1.py, and the ‘read‘ function reads the file_1.py. The code read from file_1.py will be executed by using the exec() function within file_2.py.
Python3
Python3
with open ( "file_1.py" ) as file :
exec ( file .read())
|
Output:
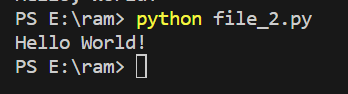
Python Run One Script From Another Using subprocess Module
In this example, file_2.py imports the subprocess module using the import statement. By using run() function Python script in “file_1.py” will be executed as a separate process within file_2.py.
Python3
print ( "This is file_1 content." )
|
Python3
import subprocess
subprocess.run([ "python" , "file_1.py" ])
|
Output:
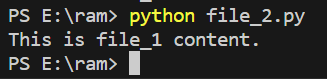
Run One Python Script From Another Using os.system() Function
In this example, file_2.py imports the os module using the import statement. Then using os.system() to execute the command “python file_1.py” and file_1.py will run within the same process but in a separate system shell.
Python3
print ( "This is file_1 content." )
|
Python3
import os
os.system( "python file_1.py" )
|
Output:
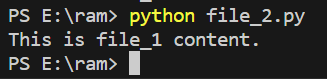
Conclusion
In conclusion, understanding how to make one Python file run another is a useful skill for writing organized and modular code. Using the import statement and grasping the concept of modules allows for improved code reusability. Incorporating functions and classes enhances the structure and readability of your code. Remembering to organize files and follow naming conventions is essential for a collaborative development process. In essence, mastering these techniques helps you create well-structured and scalable Python applications.
Share your thoughts in the comments
Please Login to comment...