Remove & Add new HTML Tags using JavaScript
Last Updated :
12 Apr, 2024
In web development, dynamically add or remove HTML tags using JavaScript. This can be useful for creating interactive user interfaces and updating content based on user actions.
Below are the approaches to remove and add new HTML tags using JavaScript:
Using createElement and removeChild
In this approach, we are using the createElement() method to dynamically add a new HTML tag and the removeChild method to remove it. This approach provides more control over the newly created tag and allows for efficient addition and removal of the tag from the DOM.
Example: The example demonstrates adding and removing new HTML Tags with JavaScript using createElement and removeChild.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<style>
h1 {
color: green;
}
#container {
margin-top: 20px;
text-align: center;
}
#container button {
margin: 2px;
padding: 5px 10px;
background-color: #3498db;
color: white;
border: none;
cursor: pointer;
}
#container button:hover {
background-color: #2980b9;
}
.new-div {
margin-top: 10px;
padding: 10px;
margin-left: 43%;
width: 100px;
background-color: green;
border-radius: 5px;
color: white;
}
</style>
</head>
<body>
<div id="container">
<h1>GeeksForGeeks</h1>
<button onclick="addDiv()">
Add Div
</button>
<button onclick="removeDiv()">
Remove Div
</button>
</div>
<script>
function addDiv() {
let container =
document.getElementById('container');
// Create a new div
let newDiv =
document.createElement('div');
newDiv.className = 'new-div';
newDiv.textContent = 'New Div Tag';
// Add the new div to the container
container.appendChild(newDiv);
}
function removeDiv() {
let container =
document.getElementById('container');
// Remove the last added div
let divs = container.querySelectorAll('.new-div');
if (divs.length > 0) {
let lastDiv = divs[divs.length - 1];
container.removeChild(lastDiv);
}
}
</script>
</body>
</html>
Output:
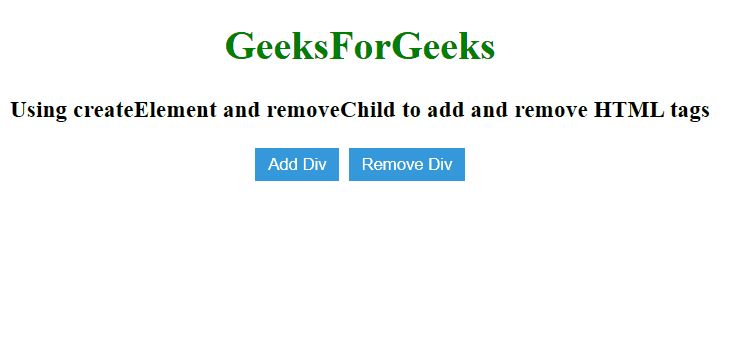
Using innerHTML
In this approach, we are using the innerHTML property to add and remove HTML Tags. Here we directly modify the innerHTML property of an element to add or remove HTML content. to add a new tag we will set the innerHTML property of a parent element to a string containing the HTML markup of the tag we want to add. And to remove we will set the innerHTML property to Null.
Example: This example uses the innerHTML property to remove and add new HTML Tags with JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<style>
h1 {
color: green;
}
body {
text-align: center;
height: 100vh;
margin: 0;
}
#container {
margin-left: 40%;
width: 20%;
text-align: center;
margin-bottom: 20px;
}
p {
margin: 5px 0;
padding: 10px;
background-color: lightblue;
border: 1px solid blue;
}
button {
padding: 5px 10px;
margin: 0 10px;
background-color: #f0f0f0;
border: 1px solid #ccc;
border-radius: 3px;
cursor: pointer;
}
button:hover {
background-color: #e0e0e0;
}
</style>
</head>
<body>
<h1>GeeksForGeeks</h1>
<h3>Using innerHTML to add
and remove HTML tags
</h3>
<button onclick="addParagraph()">
Add Paragraph
</button>
<button onclick="removeParagraphs()">
Remove Paragraphs
</button>
<div id="container">
</div>
<script>
function addParagraph() {
let container = document.getElementById('container');
container.innerHTML +=
'<p>New paragraph</p>';
}
function removeParagraphs() {
let container = document.getElementById('container');
container.innerHTML = '';
}
</script>
</body>
</html>
Output:
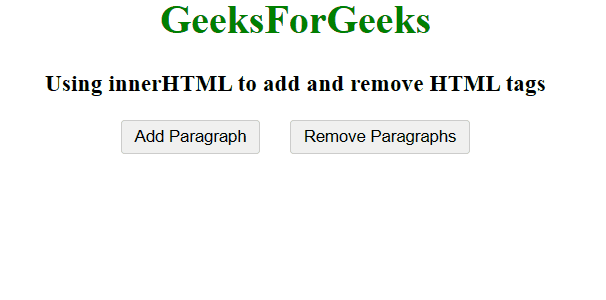
Share your thoughts in the comments
Please Login to comment...