How to Change Text Inside all HTML Tags using JavaScript ?
Last Updated :
27 Sep, 2023
In this article, we will learn how to change the text content inside all HTML tags using JavaScript. This skill is valuable when you need to dynamically modify the text displayed on a web page. which can be useful for various scenarios like updating content, internationalization, or creating dynamic user interfaces.
Changing the text inside HTML tags using JavaScript involves accessing the DOM (Document Object Model) & manipulating the text nodes of HTML elements. JavaScript provides multiple approaches to achieve this and allows you to update the text content of the specific elements or all elements on a page. Possible approaches to achieve this task are:
Approaches to Change Text Inside All HTML Tags:
- The
innerHTML
property is used to access or modify the HTML content within the element.
- To change the text inside all HTML tags using this approach you would traverse through all the elements on the page & set the
innerHTML
property for each element of the desired text.
Syntax
// Changing text using innerHTML property
const elements = document.querySelectorAll("*");
for (const element of elements) {
element.innerHTML = "Your new text here";
}
Example: This example describes the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Change Text using innerHTML</ title >
</ head >
< body >
< h1 style = "background-color: lightblue" >
Hello Geeks!.
</ h1 >
< p >
This is a simple paragraph.
</ p >
< div >
< p >
Another paragraph inside a div.
</ p >
</ div >
< button id = "changeContentButton" >
Change Content
</ button >
< script >
const elements =
document.querySelectorAll("*");
const buttonText =
"Welcome to GeeksforGeeks......";
// Function to change content
function changeContent() {
for (const element of elements) {
element.innerHTML = buttonText;
}
}
// Add a click event listener to the button
const changeButton =
document.getElementById("changeContentButton");
changeButton.addEventListener(
"click", changeContent);
</ script >
</ body >
</ html >
|
Output:
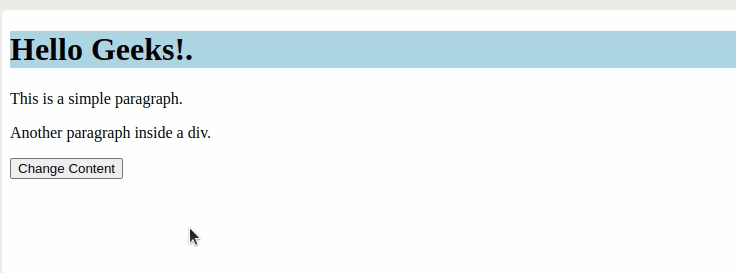
Approach 2: Using TextNode
- In this approach, you directly target the text nodes of each element & modify their text content.
- It involves iterating through all elements and their child nodes identifying the text nodes & updating their data.
Syntax
// Changing text using text nodes
function changeText(node) {
if (node.nodeType === Node.TEXT_NODE) {
node.data = "Your new text here";
} else if (node.nodeType === Node.ELEMENT_NODE) {
for (const childNode of node.childNodes) {
changeText(childNode);
}
}
}
// Call the function with the root node of the document
changeText(document.body);
Example: This example shows the use of the aboveexplained approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Change Text using the TextNode.
</ title >
</ head >
< body >
< h1 >hello Geeks.</ h1 >
< p >This is a simple paragraph.</ p >
< div >
< p >Paragraph inside a div.</ p >
</ div >
< button id = "changeContentButton" >
Change Content
</ button >
< script >
function changeText(node) {
if (node.nodeType === Node.TEXT_NODE) {
node.data =
".....Welcome to GeeksforGeeks.....";
} else if (
node.nodeType === Node.ELEMENT_NODE
) {
for (const childNode of node.childNodes) {
changeText(childNode);
}
}
}
// Function to handle button click
function handleButtonClick() {
changeText(document.body);
}
// Add a click event listener to the button
const changeButton =
document.getElementById("changeContentButton");
changeButton.addEventListener(
"click", handleButtonClick);
</ script >
</ body >
</ html >
|
Output:
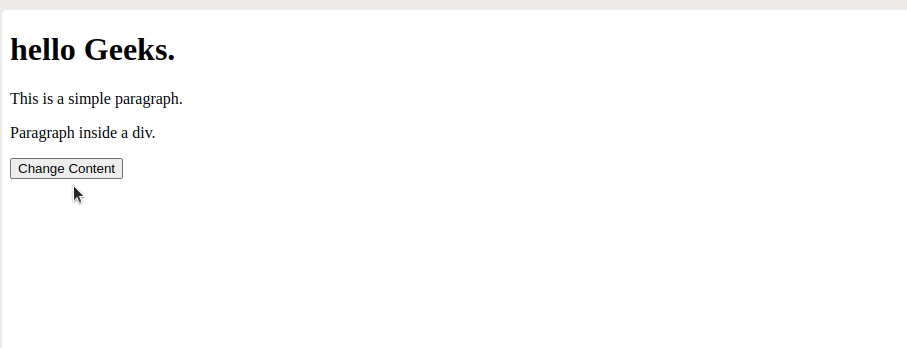
Share your thoughts in the comments
Please Login to comment...