How to Read a File From the Classpath in Java?
Last Updated :
02 Feb, 2024
Reading a file from the classpath concept in Java is important for Java Developers to understand. It is important because it plays a crucial role in the context of Resource Loading within applications. In Java, we can read a file from the classpath by using the Input Stream class. By loading the class which has the file inside it we can read the content of the file.
In this article, we will explore how to read a file from the classpath in Java.
Implementation to read a file from the classpath in Java
Project Structure:
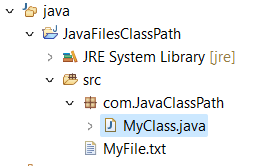
- In the above Project Structure, we have created Java Project JavaFilesClassPath inside my workingSet Java.
- Created a Package com.JavaClassPath consists of the class MyClass.java.
- Also Created a file MyFile.txt text file in the same project.
Content inside Text File:
Below we can see the content inside the text file.
MyFile.txt
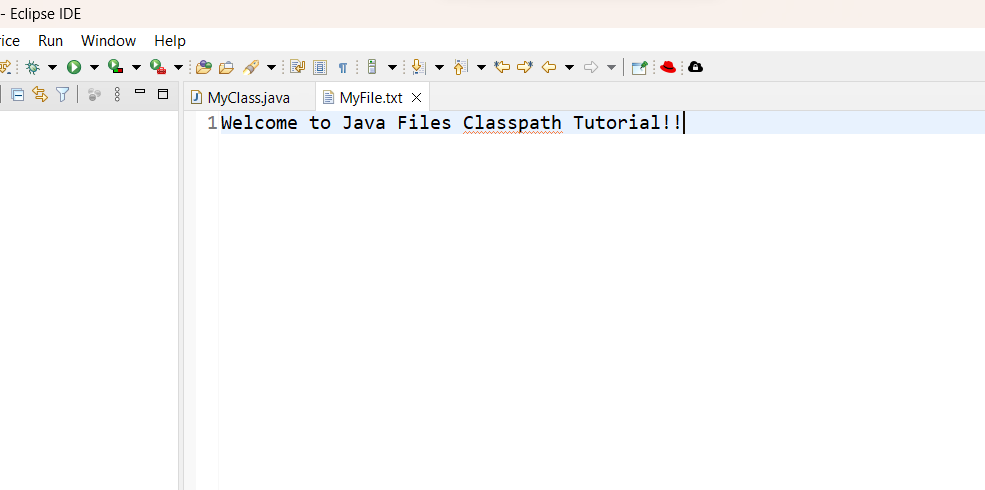
Java Program to Read a File From the Classpath
Let’s take an example of reading a file and printing its content in the console.
Java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class MyClass {
public static void main(String[] args) {
String filePath = "MyFile.txt" ;
InputStream inputStream = MyClass. class .getClassLoader().getResourceAsStream(filePath);
if (inputStream != null ) {
try (BufferedReader reader = new BufferedReader( new InputStreamReader(inputStream))) {
String line;
while ((line = reader.readLine()) != null ) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
} else {
System.out.println( "OOPS! File not found: " + filePath);
}
}
}
|
Output:
Below is the output in the console.
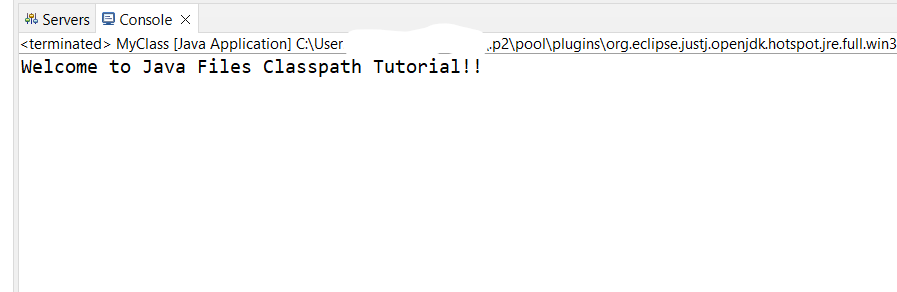
Explanation of the above Program:
- In the above code, first we have stored the file name in a string.
- The getClassLoader().getResourceAsStream(filePath) method is implemented to obtain an InputStream from the file specified by filePath. This method utilizes the class loader associated with the MyClass class.
- The buffer Reader is instantiated to read the characters efficiently from the obtained InputStream.
- The while loop is used for reading and printing the content into the console.
- Error Handling is made to trace if IO Exceptions are found.
- In the end, if the InputStream is null, “OOPS! File not Found “ is printed in the console.
Note: Import BufferedReader, IOException, InputStream, InputStreamReader Classes.
Share your thoughts in the comments
Please Login to comment...