Python PRAW – Getting the list of trophies a redditor has
Last Updated :
26 Jun, 2020
In Reddit, a redditor is the term given to a user. A redditor can earn certain trophies. Here we will see how to fetch all the trophies a redditor has. We will be using the trophies()
method of the Redditor
class to fetch all the trophies a redditor has.
trophies()
Syntax : Redditor.trophies()
Parameter : None
Returns : List of objects of class Trophy
Example 1 : Consider the following redditor :
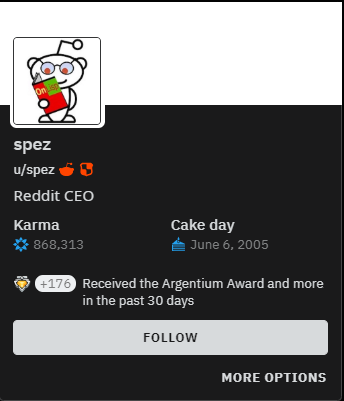
The user name of the redditor is : spez.
import praw
client_id = ""
client_secret = ""
username = ""
password = ""
user_agent = ""
reddit = praw.Reddit(client_id = client_id,
client_secret = client_secret,
username = username,
password = password,
user_agent = user_agent)
redditor_name = "spez"
redditor = reddit.redditor(redditor_name)
trophies = redditor.trophies()
for trophy in trophies:
print (trophy)
|
Output :
15-Year Club
Inciteful Comment
Inciteful Link
RPAN Viewer
Not Forgotten
Sequence | Editor
Spared
Alpha Tester
Inciteful Comment
Inciteful Link
redditgifts Exchanges
Inciteful Comment
Beta Team
Inciteful Link
Inciteful Comment
Inciteful Link
Inciteful Link
Inciteful Link
Inciteful Link
Best Comment
Best Comment
Reddit Premium
reddit mold
Rally Monkey
Verified Email
ComboCommenter
Example 2 : Consider the following redditor :
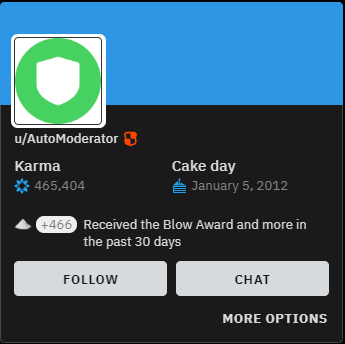
The user name of the redditor is : AutoModerator
import praw
client_id = ""
client_secret = ""
username = ""
password = ""
user_agent = ""
reddit = praw.Reddit(client_id = client_id,
client_secret = client_secret,
username = username,
password = password,
user_agent = user_agent)
redditor_name = "AutoModerator"
redditor = reddit.redditor(redditor_name)
trophies = redditor.trophies()
for trophy in trophies:
print (trophy)
|
Output :
Eight-Year Club
Undead | Zombie
Inciteful Comment
Inciteful Comment
Well-rounded
Inciteful Comment
Well-rounded
Well-rounded
Reddit Premium
Share your thoughts in the comments
Please Login to comment...