Python PostgreSQL – Update Table
Last Updated :
07 Dec, 2021
In this article, we will see how to Update data in PostgreSQL using python and Psycopg2. The update command is used to modify the existing record in the table. By default whole records of the specific attribute are modified, but to modify some particular row, we need to use the where clause along with the update clause.
Syntax for Update Clause
UPDATE table_name SET column1=value1,column2=value2,…
Table for demonstration:

Example 1: Update the columns of the table using Python – pscopg2
Here we are going to see how to update the columns of the table. The table after modification looks like the table shown below. As we can see for every tuple state value is changed to Kashmir.
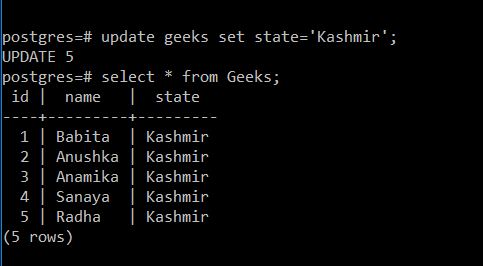
Python3
import psycopg2
conn = psycopg2.connect(
database = "postgres" ,
user = 'postgres' ,
password = 'password' ,
host = 'localhost' ,
port = '5432'
)
cursor = conn.cursor()
sql =
cursor.execute(sql)
print ( 'table updated..' )
print ( 'table after updation...' )
sql2 =
cursor.execute(sql2);
print (cursor.fetchall())
conn.commit()
conn.close()
|
Output
table updated..
table after updation...
[(1,'Babita','kashmir'),(2,'Anushka','Kashmir'),(3,'Anamika','Kashmir'),
(4,'Sanaya','Kashmir'),(5,'Radha','Kashmir')]
Example 2: Update the columns using the where clause
Here we will use the where clause along with the update table.
Syntax: UPDATE Geeks set state=’Delhi’ where id=2;
We can see state of the row having id 2 is changed from Hyderabad to Delhi.
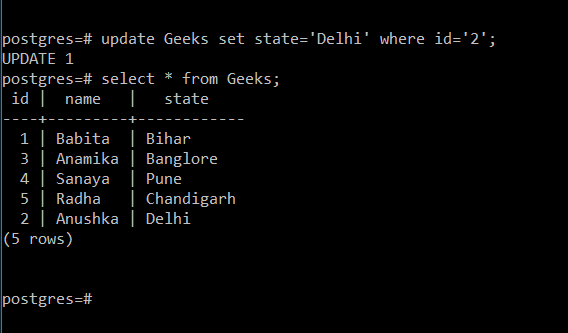
Python3
import psycopg2
conn = psycopg2.connect(
database = "postgres" ,
user = 'postgres' ,
password = 'password' ,
host = 'localhost' ,
port = '5432'
)
cursor = conn.cursor()
sql =
cursor.execute(sql)
print ( "Table updated.." )
print ( 'Table after updation...' )
sql2 = 'select * from Geeks;'
cursor.execute(sql2);
print (cursor.fetchall());
conn.commit()
conn.close()
|
Output:
Table updated..
Table after updation...
[(1, 'Babita', 'Bihar'), (3, 'Anamika', 'Banglore'),
(4, 'Sanaya', 'Pune'), (5, 'Radha', 'Chandigarh'),
(2, 'Anushka', 'Delhi')]
Share your thoughts in the comments
Please Login to comment...