Python | Pandas Series.str.extract()
Last Updated :
27 Mar, 2019
Series.str
can be used to access the values of the series as strings and apply several methods to it. Pandas Series.str.extract()
function is used to extract capture groups in the regex pat as columns in a DataFrame. For each subject string in the Series, extract groups from the first match of regular expression pat.
Syntax: Series.str.extract(pat, flags=0, expand=True)
Parameter :
pat : Regular expression pattern with capturing groups.
flags : int, default 0 (no flags)
expand : If True, return DataFrame with one column per capture group.
Returns : DataFrame or Series or Index
Example #1: Use Series.str.extract()
function to extract groups from the string in the underlying data of the given series object.
import pandas as pd
import re
sr = pd.Series([ 'New_York' , 'Lisbon' , 'Tokyo' , 'Paris' , 'Munich' ])
idx = [ 'City 1' , 'City 2' , 'City 3' , 'City 4' , 'City 5' ]
sr.index = idx
print (sr)
|
Output :
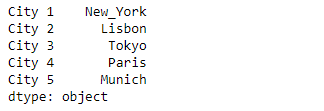
Now we will use Series.str.extract()
function to extract groups from the strings in the given series object.
result = sr. str .extract(pat = '([aeiou].)' )
print (result)
|
Output :
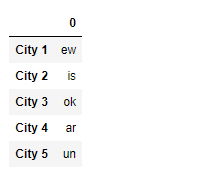
As we can see in the output, the Series.str.extract()
function has returned a dataframe containing a column of the extracted group.
Example #2 : Use Series.str.extract()
function to extract groups from the string in the underlying data of the given series object.
import pandas as pd
import re
sr = pd.Series([ 'Mike' , 'Alessa' , 'Nick' , 'Kim' , 'Britney' ])
idx = [ 'Name 1' , 'Name 2' , 'Name 3' , 'Name 4' , 'Name 5' ]
sr.index = idx
print (sr)
|
Output :
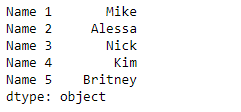
Now we will use Series.str.extract()
function to extract groups from the strings in the given series object.
result = sr. str .extract(pat = '([A-Z]i.)' )
print (result)
|
Output :
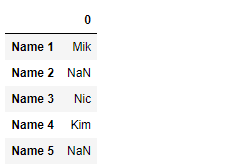
As we can see in the output, the Series.str.extract()
function has returned a dataframe containing a column of the extracted group.
Share your thoughts in the comments
Please Login to comment...