Python – Call function from another function
Last Updated :
04 Jul, 2023
Prerequisite: Functions in Python
In Python, any written function can be called by another function. Note that this could be the most elegant way of breaking a problem into chunks of small problems. In this article, we will learn how can we call a defined function from another function with the help of multiple examples.
What is Calling a Function and a Called Function?
The Function which calls another Function is called Calling Function and the function which is called by another Function is called a Called Function.
How does Function Execution Work in Python?
A stack data structure is used during the execution of the function calls. Whenever a function is invoked then the calling function is pushed into the stack and called function is executed. When the called function completes its execution and returns then the calling function is popped from the stack and executed. Calling Function execution will be completed only when Called Function’s execution is completed.
In the below figure. The function call is made from the Main function to Function1. Now the state of the Main function is stored in Stack, and execution of the Main function is continued when Function 1 returns. When Function1 calls Function2, the state of the Function1 is stored in the stack, and execution of Function 1 will be continued when Function 2 returns.
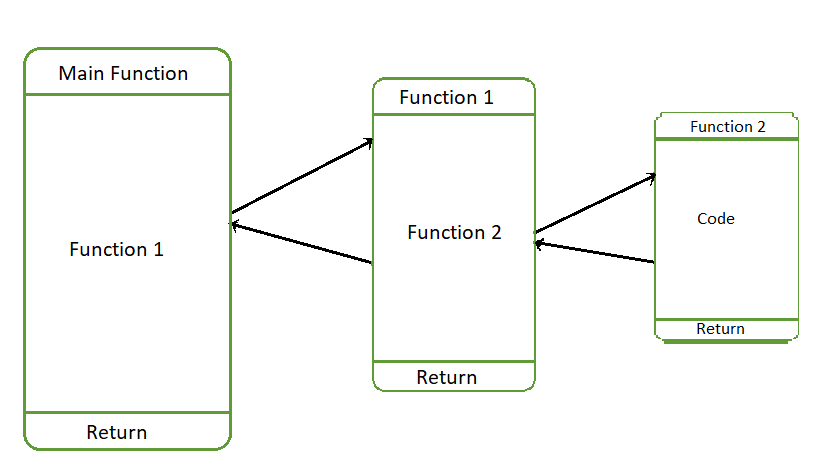
Working of Functions when one Function calls another Function
Examples of a Function Call from Another Function
Let us see a few different examples, that explain a function call from another function in Python.
Example 1:
In this example, the SumOfSquares() function calls the Square() which returns the square of the number.
Python3
def Square(X):
return (X * X)
def SumofSquares(Array, n):
Sum = 0
for i in range (n):
SquaredValue = Square(Array[i])
Sum + = SquaredValue
return Sum
Array = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 ]
n = len (Array)
Total = SumofSquares(Array, n)
print ( "Sum of the Square of List of Numbers:" , Total)
|
Output :
Sum of the Square of List of Numbers: 385
Example 2:
In this example, we will call a function from another function within the same class. The class method Function1() calls the method Function2() from the Main class.
Python3
class Main:
def __init__( self ):
self .String1 = "Hello"
self .String2 = "World"
def Function1( self ):
self .Function2()
print ( "Function1:" , self .String2)
return
def Function2( self ):
print ( "Function2:" , self .String1)
return
Object = Main()
Object .Function1()
|
Output :
Function2: Hello
Function1: World
Example 3:
In this example, we will call the parent class function from the child class function. The child class inherits the attributes from the parent class.
Python3
class Parent:
def __init__( self ):
self .String1 = "Hello"
self .String2 = "World"
def Function2( self ):
print ( "Function2:" , self .String1)
return
class Child(Parent):
def Function1( self ):
self .Function2()
print ( "Function1:" , self .String2)
return
Object1 = Parent()
Object2 = Child()
Object2.Function1()
|
Output :
Function2: Hello
Function1: World
Share your thoughts in the comments
Please Login to comment...