Program to find the quantity after mixture replacement
Last Updated :
23 Jul, 2022
Given a container that has X liters of milk. Y liters of milk is drawn out and replaced with Y liters of water. This operation is done Z times. The task is to find out the quantity of milk left in the container.
Examples:
Input: X = 10 liters, Y = 2 liters, Z = 2 times
Output: 6.4 liters
Input: X = 25 liters, Y = 6 liters, Z = 3 times
Output: 10.97 liters
Formula:-
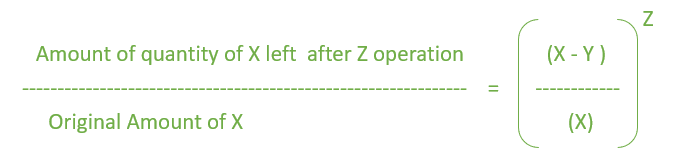
Below is the required implementation:
C++
#include <bits/stdc++.h>
using namespace std;
float Mixture( int X, int Y, int Z)
{
float result = 0.0, result1 = 0.0;
result1 = ((X - Y) / ( float )X);
result = pow (result1, Z);
result = result * X;
return result;
}
int main()
{
int X = 10, Y = 2, Z = 2;
cout << Mixture(X, Y, Z) << " litres" ;
return 0;
}
|
Java
import java.io.*;
class GFG
{
static double Mixture( int X, int Y, int Z)
{
double result1 = 0.0 , result = 0.0 ;
result1 = ((X - Y) / ( float )X);
result = Math.pow(result1, Z);
result = result * X;
return result;
}
public static void main(String[] args)
{
int X = 10 , Y = 2 , Z = 2 ;
System.out.println(( float )Mixture(X, Y, Z) +
" litres" );
}
}
|
Python 3
def Mixture(X, Y, Z):
result = 0.0
result1 = 0.0
result1 = ((X - Y) / X)
result = pow (result1, Z)
result = result * X
return result
if __name__ = = "__main__" :
X = 10
Y = 2
Z = 2
print ( "{:.1f}" . format (Mixture(X, Y, Z)) +
" litres" )
|
C#
using System;
class GFG
{
static double Mixture( int X,
int Y, int Z)
{
double result1 = 0.0, result = 0.0;
result1 = ((X - Y) / ( float )X);
result = Math.Pow(result1, Z);
result = result * X;
return result;
}
public static void Main()
{
int X = 10, Y = 2, Z = 2;
Console.WriteLine(( float )Mixture(X, Y, Z) +
" litres" );
}
}
|
PHP
<?php
function Mixture( $X , $Y , $Z )
{
$result = 0.0;
$result1 = 0.0;
$result1 = (( $X - $Y ) / $X );
$result = pow( $result1 , $Z );
$result = $result * $X ;
return $result ;
}
$X = 10;
$Y = 2;
$Z = 2;
echo Mixture( $X , $Y , $Z ), " litres" ;
?>
|
Javascript
<script>
function Mixture(X, Y, Z)
{
var result = 0.0, result1 = 0.0;
result1 = ((X - Y) / X);
result = Math.pow(result1, Z);
result = result * X;
return result;
}
var X = 10, Y = 2, Z = 2;
document.write( Mixture(X, Y, Z).toFixed(1) + " litres" );
</script>
|
Time Complexity: O(log(n))
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...