Print words of a string in reverse order
Last Updated :
21 Jul, 2022
Let there be a string say “I AM A GEEK”. So, the output should be “GEEK A AM I” . This can done in many ways. One of the solutions is given in Reverse words in a string .
Examples:
Input : I AM A GEEK
Output : GEEK A AM I
Input : GfG IS THE BEST
Output : BEST THE IS GfG
This can be done in more simpler way by using the property of the “%s format specifier” .
Property: %s will get all the values until it gets NULL i.e. ‘\0’.
Example: char String[] = “I AM A GEEK” is stored as shown in the image below :

Approach: Traverse the string from the last character, and move towards the first character. While traversing, if a space character is encountered, put a NULL in that position and print the remaining string just after the NULL character. Repeat this until the loop is over and when the loop ends, print the string, the %s will make the printing of characters until it encounters the first NULL character.
Let us see the approach with the help of diagrams:
step 1: Traverse from the last character until it encounters a space character .
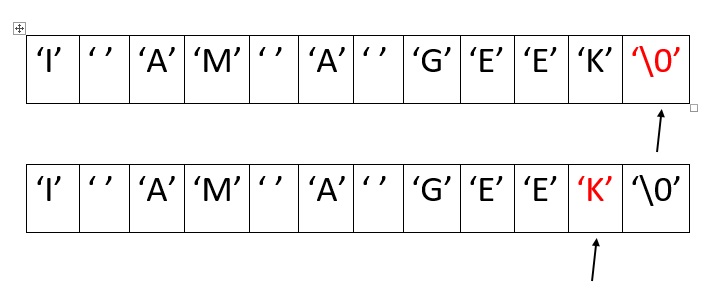
Step 2: Put a NULL character at the position of space character and print the string after it.
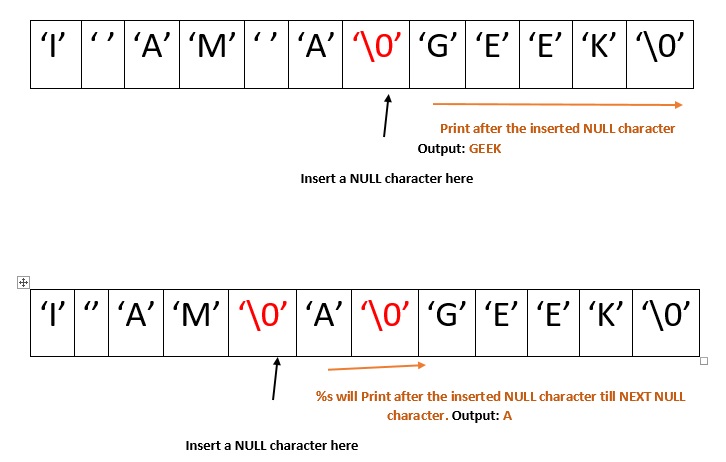
Step 3: At the end, the loop ends when it reaches the first character, so print the remaining characters, it will be printed the first NULL character, hence the first word will be printed.
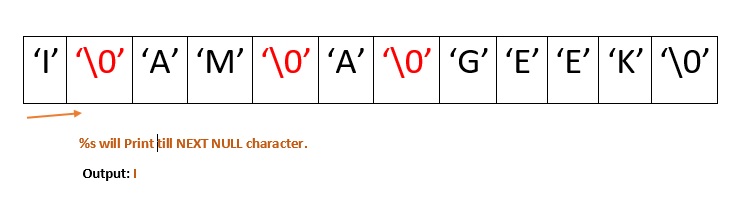
Implementation:
C++
#include <iostream>
using namespace std;
string wordReverse(string str)
{
int i = str.length() - 1;
int start, end = i + 1;
string result = "" ;
while (i >= 0) {
if (str[i] == ' ' ) {
start = i + 1;
while (start != end)
result += str[start++];
result += ' ' ;
end = i;
}
i--;
}
start = 0;
while (start != end)
result += str[start++];
return result;
}
int main()
{
string str = "I AM A GEEK" ;
cout << wordReverse(str);
return 0;
}
|
C
#include <stdio.h>
#include <string.h>
void printReverse( char str[])
{
int length = strlen (str);
int i;
for (i = length - 1; i >= 0; i--) {
if (str[i] == ' ' ) {
str[i] = '\0' ;
printf ( "%s " , &(str[i]) + 1);
}
}
printf ( "%s" , str);
}
int main()
{
char str[] = "I AM A GEEK" ;
printReverse(str);
return 0;
}
|
Java
import java.io.*;
import java.lang.*;
import java.util.*;
class GFG {
static String wordReverse(String str)
{
int i = str.length() - 1 ;
int start, end = i + 1 ;
String result = "" ;
while (i >= 0 ) {
if (str.charAt(i) == ' ' ) {
start = i + 1 ;
while (start != end)
result += str.charAt(start++);
result += ' ' ;
end = i;
}
i--;
}
start = 0 ;
while (start != end)
result += str.charAt(start++);
return result;
}
public static void main(String[] args)
{
String str = "I AM A GEEK" ;
System.out.print(wordReverse(str));
}
}
|
Python3
def wordReverse( str ):
i = len ( str ) - 1
start = end = i + 1
result = ''
while i > = 0 :
if str [i] = = ' ' :
start = i + 1
while start ! = end:
result + = str [start]
start + = 1
result + = ' '
end = i
i - = 1
start = 0
while start ! = end:
result + = str [start]
start + = 1
return result
str = 'I AM A GEEK'
print (wordReverse( str ))
|
C#
using System;
class GFG {
static String wordReverse(String str)
{
int i = str.Length - 1;
int start, end = i + 1;
String result = "" ;
while (i >= 0) {
if (str[i] == ' ' ) {
start = i + 1;
while (start != end)
result += str[start++];
result += ' ' ;
end = i;
}
i--;
}
start = 0;
while (start != end)
result += str[start++];
return result;
}
public static void Main()
{
String str = "I AM A GEEK" ;
Console.Write(wordReverse(str));
}
}
|
PHP
<?php
function wordReverse( $str )
{
$i = strlen ( $str ) - 1;
$end = $i + 1;
$result = "" ;
while ( $i >= 0)
{
if ( $str [ $i ] == ' ' )
{
$start = $i + 1;
while ( $start != $end )
$result = $result . $str [ $start ++];
$result = $result . ' ' ;
$end = $i ;
}
$i --;
}
$start = 0;
while ( $start != $end )
$result = $result . $str [ $start ++];
return $result ;
}
$str = "I AM A GEEK" ;
echo wordReverse( $str );
?>
|
Javascript
<script>
function wordReverse(str)
{
var i = str.length - 1;
var start, end = i + 1;
var result = "" ;
while (i >= 0)
{
if (str[i] == ' ' )
{
start = i + 1;
while (start != end)
result += str[start++];
result += ' ' ;
end = i;
}
i--;
}
start = 0;
while (start != end)
result += str[start++];
return result;
}
var str = "I AM A GEEK" ;
document.write(wordReverse(str));
</script>
|
Time Complexity: O(len(str))
Auxiliary Space: O(len(str))
Without using any extra space:
Go through the string and mirror each word in the string, then, at the end, mirror the whole string.





Implementation: The following C++ code can handle multiple contiguous spaces.
C++
#include <algorithm>
#include <iostream>
#include <string>
using namespace std;
string reverse_words(string s)
{
int left = 0, i = 0, n = s.length();
while (s[i] == ' ' ){
i++;
}
left = i;
while (i < n)
{
if (i + 1 == n || s[i] == ' ' )
{
int j = i - 1;
if (i + 1 == n)
j++;
reverse(s.begin()+left, s.begin()+j+1);
left = i + 1;
}
if (left < n && s[left] == ' ' && i > left)
left = i;
i++;
}
reverse(s.begin(), s.end());
return s;
}
int main()
{
string str = "I AM A GEEK" ;
str = reverse_words(str);
cout << str;
return 0;
}
|
Java
import java.io.*;
class GFG {
static String reverse_words(String s)
{
int left = 0 , i = 0 , n = s.length();
while (s.charAt(i) == ' ' ){
i++;
}
left = i;
while (i < n)
{
if (i + 1 == n || s.charAt(i) == ' ' )
{
int j = i - 1 ;
if (i + 1 == n)
j++;
while (left<n && j<n && left < j){
char ch[] = s.toCharArray();
char temp = ch[left];
ch[left] = ch[j];
ch[j] = temp;
s = String.valueOf(ch);
left++;
j--;
}
left = i + 1 ;
}
if (left < n && s.charAt(left) == ' ' && i > left)
left = i;
i++;
}
char ch[] = s.toCharArray();
int len = s.length();
for (i= 0 ; i < (len/ 2 ); i++)
{
char temp = ch[i];
ch[i] = ch[len - i - 1 ];
ch[len - i- 1 ] = temp;
}
s = String.valueOf(ch);
return s;
}
public static void main(String args[])
{
String str = "I AM A GEEK" ;
str = reverse_words(str);
System.out.println(str);
}
}
|
Python3
def reverse_words(s):
left, i, n = 0 , 0 , len (s)
while (s[i] = = ' ' ):
i + = 1
left = i
while (i < n):
if (i + 1 = = n or s[i] = = ' ' ):
j = i - 1
if (i + 1 = = n):
j + = 1
while (left < j):
s = s[ 0 :left] + s[j] + s[left + 1 :j] + s[left] + s[j + 1 :]
left + = 1
j - = 1
left = i + 1
if (i > left and s[left] = = ' ' ):
left = i
i + = 1
s = s[:: - 1 ]
return s
Str = "I AM A GEEK"
Str = reverse_words( Str )
print ( Str )
|
C#
using System;
class GFG {
static String reverse_words(String s)
{
var left = 0;
var i = 0;
var n = s.Length;
while (s[i] == ' ' ) {
i++;
}
left = i;
while (i < n) {
if (i + 1 == n || s[i] == ' ' ) {
var j = i - 1;
if (i + 1 == n) {
j++;
}
while (left < n && j < n && left < j) {
char [] ch = s.ToCharArray();
var temp = ch[left];
ch[left] = ch[j];
ch[j] = temp;
s = new string (ch);
left++;
j--;
}
left = i + 1;
}
if (left < n && s[left] == ' ' && i > left) {
left = i;
}
i++;
}
int len = s.Length;
char [] chh = s.ToCharArray();
for (i = 0; i < (len / 2); i++) {
char temp = chh[i];
chh[i] = chh[len - i - 1];
chh[len - i - 1] = temp;
}
s = new string (chh);
return s;
}
public static void Main(String[] args)
{
string str = "I AM A GEEK" ;
str = reverse_words(str);
Console.WriteLine(str);
}
}
|
Javascript
<script>
function reverse_words(s)
{
let left = 0, i = 0, n = s.length;
while (s[i] == ' ' )
i++;
left = i;
while (i < n)
{
if (i + 1 == n || s[i] == ' ' )
{
let j = i - 1;
if (i + 1 == n)
j++;
let temp;
let a = s.split( "" );
while (left < j){
temp = a[left];
a[left] = a[j];
a[j] = temp;
left++;
j--;
}
s = a.join( "" );
left = i + 1;
}
if (s[left] == ' ' && i > left)
left = i;
i++;
}
s = s.split( '' ).reverse().join( '' );
return s;
}
let str = "I AM A GEEK" ;
str = reverse_words(str);
document.write(str);
</script>
|
Time Complexity: O(len(str))
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...