PHP Program to Convert Fahrenheit to Celsius
Last Updated :
27 Feb, 2024
Converting temperature from Fahrenheit to Celsius is a common task in many applications. The formula to convert Fahrenheit to Celsius is:-
Celsius = (Fahrenheit - 32) * 5/9
In this article, we will explore how to implement this conversion in PHP using different approaches such as inline calculations, functions, and using HTML.
Convert Fahrenheit to Celsius using Inline Calculation
If you only need to perform the conversion once or in a specific context, you can do the calculation inline without defining a separate function.
Example: Illustration of Calculating Fahrenheit to Celsius in PHP using inline calculations.
PHP
<?php
$fahrenheit = 98.6;
$celsius = ( $fahrenheit - 32) * 5 / 9;
echo "{$fahrenheit}°F is = {$celsius}°C" ;
?>
|
Output
98.6°F is = 37°C
Time Complexity:Â O(1)
Auxiliary Space:Â O(1)
Convert Fahrenheit to Celsius using a Function
One way to perform the conversion is by defining a function that takes the Fahrenheit temperature as an input and returns the Celsius temperature.
Example: Illustration of Calculating Fahrenheit to Celsius in PHP using a function.
PHP
<?php
function fahToCel( $fahrenheit ) {
$celsius = ( $fahrenheit - 32) * 5 / 9;
return $celsius ;
}
$fahrenheit = 98.6;
$celsius = fahToCel( $fahrenheit );
echo "{$fahrenheit}°F is {$celsius}°C" ;
?>
|
Time Complexity:Â O(1)
Auxiliary Space:Â O(1)
In a more interactive scenario, you might want to convert a temperature entered by the user. This can be done using an HTML form to get the Fahrenheit temperature as input and PHP to process this input.
Example: Illustration of Calculating Fahrenheit to Celsius in PHP through User Input using HTML.
PHP
<!DOCTYPE html>
<html>
<head>
<title>Fahrenheit to Celsius Conversion</title>
</head>
<body>
<form action= "" method= "post" >
<label for = "fahrenheit" >Fahrenheit:</label>
<input type= "text" id= "fahrenheit" name= "fahrenheit" >
<input type= "submit" value= "Convert" >
</form>
<?php
if (isset( $_POST [ 'fahrenheit' ])) {
$fahrenheit = $_POST [ 'fahrenheit' ];
$celsius = ( $fahrenheit - 32) * 5 / 9;
echo "<p>{$fahrenheit}°F is {$celsius}°C</p>" ;
}
?>
</body>
</html>
|
Output:
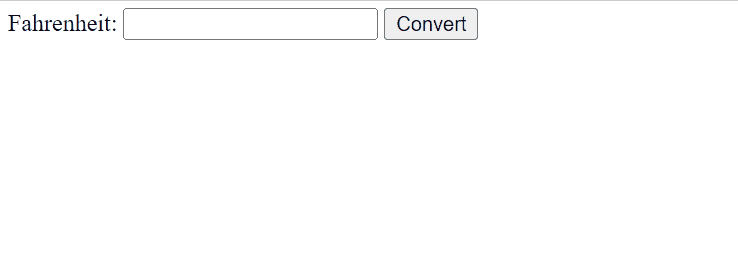
Time Complexity:Â O(1)
Auxiliary Space:Â O(1)
Share your thoughts in the comments
Please Login to comment...