PHP | $_FILES Array (HTTP File Upload variables)
Last Updated :
06 Jun, 2022
How does the PHP file handle know some basic information like file-name, file-size, type of the file and a few attributes about the file which has been selected to be uploaded? Let’s have a look at what is playing behind the scene. $_FILES is a two-dimensional associative global array of items which are being uploaded via the HTTP POST method and holds the attributes of files such as:
Attribute |
Description |
[name] |
Name of file which is uploading |
[size] |
Size of the file |
[type] |
Type of the file (like .pdf, .zip, .jpeg…..etc) |
[tmp_name] |
A temporary address where the file is located before processing the upload request |
[error] |
Types of error occurred when the file is uploading |
Now see How does the array look like??
$_FILES[input-field-name][name]
$_FILES[input-field-name][tmp_name]
$_FILES[input-field-name][size]
$_FILES[input-field-name][type]
$_FILES[input-field-name][error]
Approach: Make sure you have XAMPP or WAMP installed on your machine. In this article, we will be using the XAAMP server.
Let us go through examples, of how this PHP array works in the first example.
Example 1 :
HTML
<? php
echo "<pre>";
print_r($_FILES);
echo "</ pre >";
?>
< form action = "" method = "post" enctype = "multipart/form-data" >
< input type = "file" name = "file" >
< input type = "submit" value = "Upload Image" >
</ form >
|
In the above script, before uploading the file
Once when we select the file and upload then the function print_r will display the information of the PHP superglobal associative array $_FILES.
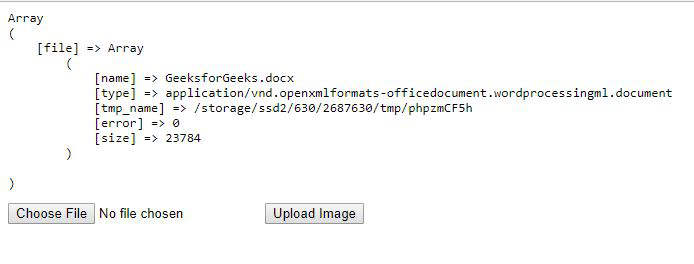
output array
Example 2: Add the HTML code followed by PHP script in different files. Let’s make an HTML form for uploading the file index.html
HTML
<!DOCTYPE html>
< html >
< head >
< title >GeeksForGeeks</ title >
< style type = "text/css" >
div {
background: #4CB974;
text-align: center;
font-size: 20px;
padding: 30px;
color: #fff;
font-family: sans-serif;
}
form {
border: 1px solid #1f1f1f;
padding: 20px;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
</ style >
</ head >
< body >
< form action = "file-upload-manager.php" method = "post" enctype = "multipart/form-data" >
< h2 >Upload File</ h2 >
< input type = "file" name = "photo" id = "fileSelect" >< br >< br >
< input type = "submit" name = "submit" value = "Upload" >< br >< br >
< div > This Video is made for GFG</ div >
</ form >
</ body >
</ html >
|
Now, time to write a PHP script which is able to handle the file uploading system. file-upload-manager.php
PHP
<?php
if ( $_SERVER [ "REQUEST_METHOD" ] == "POST" ) {
if (isset( $_FILES [ "photo" ]) && $_FILES [ "photo" ][ "error" ] == 0) {
$file_name = $_FILES [ "photo" ][ "name" ];
$file_type = $_FILES [ "photo" ][ "type" ];
$file_size = $_FILES [ "photo" ][ "size" ];
$file_tmp_name = $_FILES [ "photo" ][ "tmp_name" ];
$file_error = $_FILES [ "photo" ][ "error" ];
echo "<div style='text-align: center; background: #4CB974;
padding: 30px 0 10px 0; font-size: 20px; color: #fff'>
File Name: " . $file_name . " </div>";
echo "<div style='text-align: center; background: #4CB974;
padding: 10px; font-size: 20px; color: #fff'>
File Type: " . $file_type . " </div>";
echo "<div style='text-align: center; background: #4CB974;
padding: 10px; font-size: 20px; color: #fff'>
File Size: " . $file_size . " </div>";
echo "<div style='text-align: center; background: #4CB974;
padding: 10px; font-size: 20px; color: #fff'>
File Error: " . $file_error . " </div>";
echo "<div style='text-align: center; background: #4CB974;
padding: 10px; font-size: 20px; color: #fff'>
File Temporary Name: " . $file_tmp_name . " </div>";
}
}
?>
|
Once we submit the form in the above script, we can later access the information via a PHP superglobal associative array $_FILES. Apart from using the $_FILES array, many in-built functions are playing a major role. After we are done with uploading a file, in the script we will check the request method of the server, if it is POST then it will proceed otherwise the system will throw an error. Later on, we accessed the $_FILES array to get the file name, file size, and type of the file. Once we got those pieces of information print the information of the file using echo.
Output:
Reference: http://php.net/manual/en/reserved.variables.files.php
PHP is a server-side scripting language designed specifically for web development. You can learn PHP from the ground up by following this PHP Tutorial and PHP Examples.
Share your thoughts in the comments
Please Login to comment...