p5.js resetShader() Method
Last Updated :
21 May, 2021
The p5.js resetShader() method restores the default shaders in WEBGL mode. Code that runs after this method will not be affected by previously defined shaders.
Syntax:
resetShader(kindOfShader)
Parameters: It accepts the kindOfShader as an optional parameter which is an integer describing the type of shader, either point, lines, or triangles.
Returns Type: It has a return type of void.
Below example illustrates the resetShader() function in p5.js:
Javascript
let m;
function preload() {
m = loadShader( 'shader.vert' , 'shader.frag' );
}
function setup() {
createCanvas(100, 100, WEBGL);
shader(m);
noStroke();
m.setUniform( 'p' , [-0.74364388703, 0.13182590421]);
resetShader();
}
function draw() {
m.setUniform( 'r' , 1.5 * exp(-6.5 * (1 + sin(millis() / 2000))));
quad(-1, -1, 1, -1, 1, 1, -1, 1);
}
|
shader.vert
precision highp float; varying vec2 vPos;
attribute vec3 aPosition;
void main() { vPos = (gl_Position = vec4(aPosition,1.0)).xy; }
shader.frag
precision highp float; varying vec2 vPos;
uniform vec2 p;
uniform float r;
const int I = 500;
void main() {
vec2 c = p + vPos * r, z = c;
float n = 0.0;
for (int i = I; i > 0; i --) {
if(z.x*z.x+z.y*z.y > 4.0) {
n = float(i)/float(I);
break;
}
z = vec2(z.x*z.x-z.y*z.y, 2.0*z.x*z.y) + c;
}
gl_FragColor = vec4(0.5-cos(n*17.0)/2.0,0.5-cos(n*13.0)/2.0,0.5-cos(n*23.0)/2.0,1.0);
}
Output:
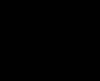
On commenting out resetShader() method, the following will be the output.
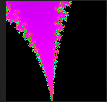
Reference: https://p5js.org/reference/#/p5/resetshader
Share your thoughts in the comments
Please Login to comment...