Most efficient Way to Convert an HTMLCollection to an Array
Last Updated :
29 Jan, 2024
An HTMLCollection is a collection of HTML elements that looks like an array, and it is returned by various DOM methods, such as “getElementsByTagName” or “querySelectorAll”. However, this collection is not an actual array, and it does not support array-specific operations. Therefore, it may be necessary to convert the HTMLCollection to an array to perform certain array-specific operations. This article will explore all possible methods of converting an HTMLCollection to an array.
Using Array. prototype.slice():
In this method, we bind the call() method with Array.prototype.slice(). This will invoke Array.prototype.slice() on HTMLCollection. This method converts the HTMLCollection to the array by creating a shallow copy of it. Below is the syntax we’ll use.
Syntax:
Array.prototype.slice.call(htmlCollection)
Example: This example uses the Array.prototype.slice() method to convert an HTMLCollection to an Array in an HTML document.
HTML
<!DOCTYPE html>
< html >
< head >
< title >HTMLCollection To Array Conversion</ title >
</ head >
< body >
< div >
< p >This is para 1</ p >
< p >This is para 2</ p >
< p >This is para 3</ p >
</ div >
< script >
// Return an HTMLCollection
const htmlCollection =
document.getElementsByTagName("p");
const array = Array.prototype
.slice.call(htmlCollection);
console.log(array);
</ script >
</ body >
</ html >
|
Output:
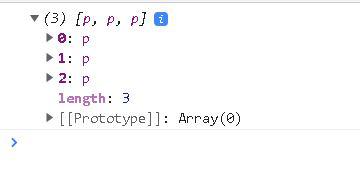
HTMLCollection to Array – Method 1
Using Array.from():
The Array.from() method is available from ES6. This method takes an array-like iterable object and returns a new array with the same elements. In our case the array-like iterable object is HTMLCollection. We simply pass it to this method and accept an array from it.
Syntax:
Array.from(htmlCollection)
Example: This example uses the Array.from() method to convert an HTMLCollection to an Array in an HTML document.
HTML
<!DOCTYPE html>
< html >
< head >
< title >HTMLCollection to Array Conversion</ title >
</ head >
< body >
< div >
< p >This is para 1</ p >
< p >This is para 2</ p >
< p >This is para 3</ p >
</ div >
< script >
// Return an HTMLCollection
const htmlCollection =
document.getElementsByTagName("p");
const array = Array.from(htmlCollection);
console.log(array);
</ script >
</ body >
</ html >
|
Output:
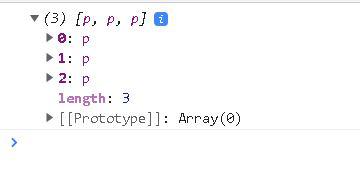
HTMLCollection to Array – Method 2
Using Spread Operator:
Using spread opera also we can convert an HTMLCollection to an array. The spread operator(. . .) expands an iterable object into multiple elements. With the use of the spread syntax inside the square brackets, we can create an array containing the same element as HTMLCollection.
Syntax:
[...htmlCollection]
Example: This example uses the spread operator to convert an HTMLCollection to an Array in an HTML document.
HTML
<!DOCTYPE html>
< html >
< head >
< title >HTMLCollection to Array Conversion</ title >
</ head >
< body >
< div >
< p >This is para 1</ p >
< p >This is para 2</ p >
< p >This is para 3</ p >
</ div >
< script >
// Return an HTMLCollection
const htmlCollection =
document.getElementsByTagName("p");
const array = [...htmlCollection];
console.log(array);
</ script >
</ body >
</ html >
|
Output:
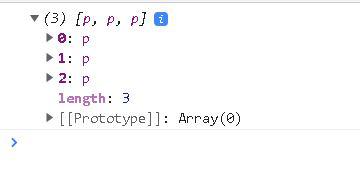
HTMLCollection to Array – Method 3
Using for-loop:
This is the most common approach to convert an HTMLCollection to an array. Here we simply iterate over the HTMLCollection and push each element into a new array.
Syntax:
const array = [];
for (let i = 0; i < collection.length; i++) {
array.push(collection[i]);
}
Example: This example uses the for loop approach to convert an HTMLCollection to an Array in an HTML document.
HTML
<!DOCTYPE html>
< html >
< head >
< title >HTMLCollection to Array Conversion</ title >
</ head >
< body >
< div >
< p >This is para 1</ p >
< p >This is para 2</ p >
< p >This is para 3</ p >
</ div >
< script >
// Return an HTMLCollection
const collection = document.getElementsByTagName('p');
const array = [];
for (let i = 0; i < collection.length ; i++) {
array.push(collection[i]);
}
console.log(array);
</script>
</ body >
</ html >
|
Output:
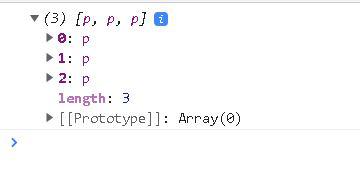
HTMLCollection to Array – Method 4
Share your thoughts in the comments
Please Login to comment...