Minimum distance to collect coins and place them in House
Last Updated :
08 Dec, 2023
Given an m*n matrix that contains character ‘B’ to represent the boy’s initial position, ‘H’ represents the house’s position, ‘C’ represents the position of coins, and ‘.’ represents empty cells, the task is to find the minimum distance (i.e. minimum moves) for the boy to collect all the coins and place them inside the house one by one. The boy can only pick up one coin at a time and can move in four directions: up, down, left, and right, to the adjacent cell.
Example:
Input: m = 5, n = 7, H = {2,2}, B = {4,4}, C : {{3,0}, {2,5}}
Output: 12
Explanation:
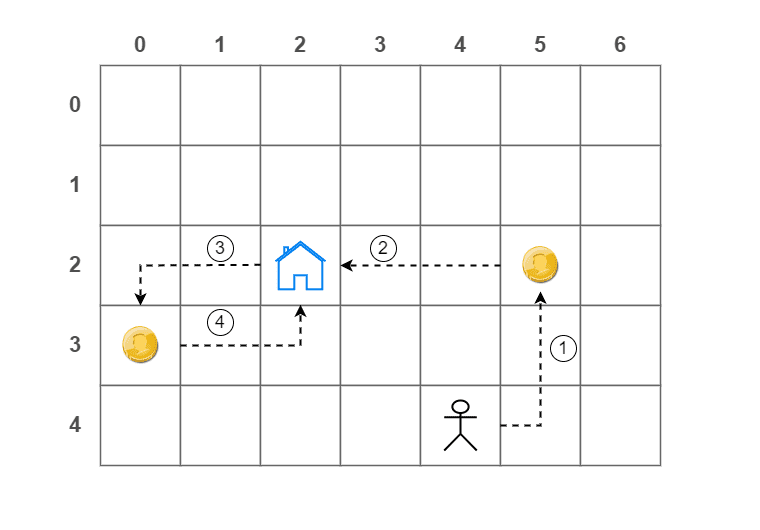
Input: m = 1, n = 3, H = {0,1}, B = {0,0}, C = {{0,2}}
Output: 3
Minimum distance to collect coins and place them in House using Manhattan distance:
The boy needs to visit all the coins and, for each coin, boy must travel from the coin’s position to the house’s position twice, except for the first coin. For the first coin, the boy travels from his initial position to the coin’s position and then to the house’s position. So, to minimize the total distance traveled, our aim is to choose the first coin in such a way that the overall distance is minimized.
Step-by-step approach:
- Calculate the total distance from the house to all coins by summing up the Manhattan distances between the house and each coin.
- Check, if the house and the boy are already at the same position, return 2 * total distance (since the boy picks up and drops off the coins at the same location).
- Iterate through each coin and calculate:
- Calculate Manhattan distance from the boy to the coin (diffBoy2Coin).
- The Manhattan distance from the house to the coin (diffHouse2Coin).
- Update the result with the minimum possible distance: 2 * total distance + diffBoy2Coin – diffHouse2Coin
- Finally, return the result
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int solve( int m, int n, vector< int >& house,
vector< int >& boy, vector<vector< int > >& coins)
{
int sum = 0;
for ( auto coin : coins) {
sum += abs (house[0] - coin[0])
+ abs (house[1] - coin[1]);
}
if (house[0] == boy[0] && house[1] == boy[1])
return 2 * sum;
int result = INT_MAX;
for ( int i = 0; i < coins.size(); i++) {
int diffBoy2Coin = abs (boy[0] - coins[i][0])
+ abs (boy[1] - coins[i][1]);
int diffHouse2Coin = abs (house[0] - coins[i][0])
+ abs (house[1] - coins[i][1]);
result = min(result, 2 * sum + diffBoy2Coin
- diffHouse2Coin);
}
return result;
}
int main()
{
int m = 5, n = 7;
vector< int > H = { 2, 2 };
vector< int > B = { 4, 4 };
vector<vector< int > > C = { { 3, 0 }, { 2, 5 } };
cout << solve(m, n, H, B, C);
return 0;
}
|
Java
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class CoinCollection {
public static int solve( int m, int n,
List<Integer> house,
List<Integer> boy,
List<List<Integer> > coins)
{
int sum = 0 ;
for (List<Integer> coin : coins) {
sum += Math.abs(house.get( 0 ) - coin.get( 0 ))
+ Math.abs(house.get( 1 ) - coin.get( 1 ));
}
if (house.get( 0 ).equals(boy.get( 0 ))
&& house.get( 1 ).equals(boy.get( 1 )))
return 2 * sum;
int result = Integer.MAX_VALUE;
for ( int i = 0 ; i < coins.size(); i++) {
int diffBoy2Coin
= Math.abs(boy.get( 0 ) - coins.get(i).get( 0 ))
+ Math.abs(boy.get( 1 )
- coins.get(i).get( 1 ));
int diffHouse2Coin
= Math.abs(house.get( 0 )
- coins.get(i).get( 0 ))
+ Math.abs(house.get( 1 )
- coins.get(i).get( 1 ));
result = Math.min(result, 2 * sum + diffBoy2Coin
- diffHouse2Coin);
}
return result;
}
public static void main(String[] args)
{
int m = 5 , n = 7 ;
List<Integer> H = Arrays.asList( 2 , 2 );
List<Integer> B = Arrays.asList( 4 , 4 );
List<List<Integer> > C = new ArrayList<>();
C.add(Arrays.asList( 3 , 0 ));
C.add(Arrays.asList( 2 , 5 ));
System.out.println(solve(m, n, H, B, C));
}
}
|
Python3
def solve(m, n, house, boy, coins):
sum = 0
for coin in coins:
sum + = abs (house[ 0 ] - coin[ 0 ]) + abs (house[ 1 ] - coin[ 1 ])
if house[ 0 ] = = boy[ 0 ] and house[ 1 ] = = boy[ 1 ]:
return 2 * sum
result = float ( 'inf' )
for i in range ( len (coins)):
diffBoy2Coin = abs (boy[ 0 ] - coins[i][ 0 ]) + abs (boy[ 1 ] - coins[i][ 1 ])
diffHouse2Coin = abs (house[ 0 ] - coins[i][ 0 ]) + abs (house[ 1 ] - coins[i][ 1 ])
result = min (result, 2 * sum + diffBoy2Coin - diffHouse2Coin)
return result
m = 5
n = 7
H = [ 2 , 2 ]
B = [ 4 , 4 ]
C = [[ 3 , 0 ], [ 2 , 5 ]]
print (solve(m, n, H, B, C))
|
C#
using System;
using System.Collections.Generic;
class GFG
{
static int Solve( int m, int n, List< int > house,
List< int > boy, List<List< int >> coins)
{
int sum = 0;
foreach ( var coin in coins)
{
sum += Math.Abs(house[0] - coin[0])
+ Math.Abs(house[1] - coin[1]);
}
if (house[0] == boy[0] && house[1] == boy[1])
return 2 * sum;
int result = int .MaxValue;
for ( int i = 0; i < coins.Count; i++)
{
int diffBoy2Coin = Math.Abs(boy[0] - coins[i][0])
+ Math.Abs(boy[1] - coins[i][1]);
int diffHouse2Coin = Math.Abs(house[0] - coins[i][0])
+ Math.Abs(house[1] - coins[i][1]);
result = Math.Min(result, 2 * sum + diffBoy2Coin
- diffHouse2Coin);
}
return result;
}
static void Main()
{
int m = 5, n = 7;
List< int > H = new List< int > { 2, 2 };
List< int > B = new List< int > { 4, 4 };
List<List< int >> C = new List<List< int >> { new List< int > { 3, 0 }, new List< int > { 2, 5 } };
Console.WriteLine(Solve(m, n, H, B, C));
}
}
|
Javascript
function solve(m, n, house, boy, coins) {
let sum = 0;
for (let coin of coins) {
sum += Math.abs(house[0] - coin[0]) + Math.abs(house[1] - coin[1]);
}
if (house[0] === boy[0] && house[1] === boy[1]) {
return 2 * sum;
}
let result = Number.MAX_SAFE_INTEGER;
for (let i = 0; i < coins.length; i++) {
let diffBoy2Coin = Math.abs(boy[0] - coins[i][0]) + Math.abs(boy[1] - coins[i][1]);
let diffHouse2Coin = Math.abs(house[0] - coins[i][0]) + Math.abs(house[1] - coins[i][1]);
result = Math.min(result, 2 * sum + diffBoy2Coin - diffHouse2Coin);
}
return result;
}
let m = 5, n = 7;
let H = [2, 2];
let B = [4, 4];
let C = [[3, 0], [2, 5]];
console.log(solve(m, n, H, B, C));
|
Time Complexity: O(|C|), where |C| is coin size.
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...