Kotlin unlabelled continue
Last Updated :
28 Mar, 2022
In this article, we will learn how to use continue in kotlin. While working with loop in the programming, sometimes, it is desirable to skip the current iteration of the loop. In that case, we can use continue statement in the program. Basically, continue is used to repeat the loop for a specific condition. It skips the following statements and continues with the next iteration of the loop.
There are two types of continue in kotlin-
We are going to learn how to use unlabelled continue in while, do-while and for loop.
Use of unlabelled continue in while loop –
In Kotlin, unlabelled continue is used to skip the current iteration of the nearest enclosing while loop. If the condition for continue is true, then it skips the following instructions of continue and reaches to the starting of while loop. Again, it will check for the condition and loop will be in repetition mode until it becomes false.
Syntax of unlabelled continue in while loop-
while(condition) {
//code
if(condition for continue) {
continue
}
//code
}
Flowchart-
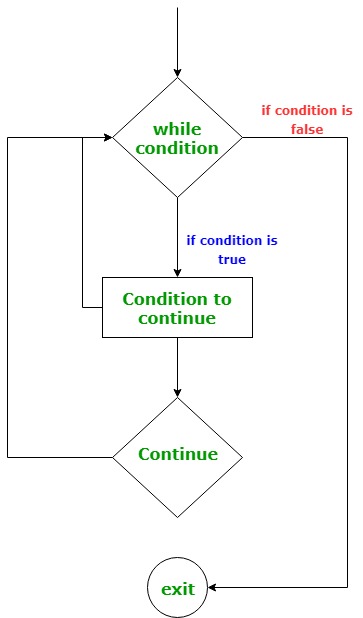
Kotlin program to use the continue in while loop.
Kotlin
fun main(args: Array<String>) {
var num = 0
while (num <= 12 ) {
if (num % 3 == 0 ) {
num++
continue
}
println(num)
num++
}
}
|
Output:
1
2
4
5
7
8
10
11
Here, in the above program, we print the numbers and skips all multiples of 3. The expression (num % 3 == 0) is used to check that number is divisible by 3 or not. If it is multiple of 3 then increment the number without printing it to standard output.
Use of unlabelled continue in do-while loop –
In do-while also we can use the unlabelled continue to skip the iteration of the nearest closing loop. Here, we need to put the condition for continue in do block. If condition becomes false it will skip the following instruction and transfer control to the while condition.
Syntax of unlabelled continue in do-while loop-
do{
// codes
if(condition for continue) {
continue
}
}
while(condition)
Flowchart-
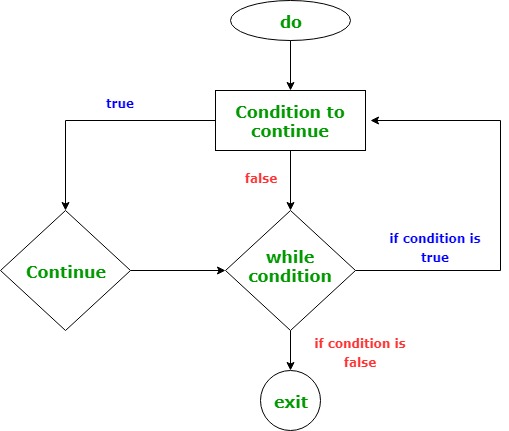
Kotlin program to use continue in do-while loop.
Kotlin
fun main(args: Array<String>) {
var num = 1
do {
if (num <= 5 || num >= 15 ) {
num++
continue
}
println( "$num" )
num++
} while (num < 20 )
}
|
Output:
6
7
8
9
10
11
12
13
14
In the above program, we have used the condition (num <= 5 || num >=15) to skip the printing of numbers to the standard output which is less than or equal to 5 and greater than or equal to 15. So, it only prints the numbers from 6 to 14.
Use of unlabelled continue in for loop –
In for loop also we can use unlabelled continue to skip the current iteration to the closing loop. In below program, we have taken an array of strings and i the iterator for the traversal of array planets. The expression ( i < 2 ) skips the iteration of array indices less than two which means it does not print the strings stored at index 0 and 1.
Syntax for unlabelled continue in for loop-
for(iteration through iterator)
{
//code
if(condition for continue){
continue
}
}
Flowchart-
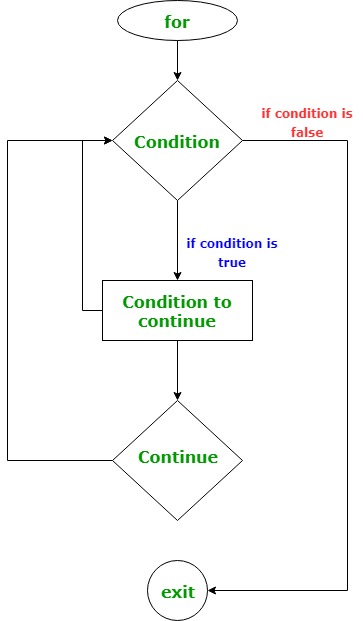
Kotlin program to use continue in for loop.
Kotlin
fun main(args: Array<String>) {
var planets = arrayOf( "Earth" , "Mars" , "Venus" , "Jupiter" , "Saturn" )
for (i in planets.indices) {
if (i < 2 ){
continue
}
println(planets[i])
}
}
|
Output:
Venus
Jupiter
Saturn
Share your thoughts in the comments
Please Login to comment...