JSTL Formatting tags
Last Updated :
21 Mar, 2024
JSTL stands for JavaServer Pages Standard Tag Library. It is introduced in the JSP 2.0 version to simplify the JSP. By using JSTL, JSP becomes a 100% tag-based application. JSTL can provide the group of tags that are used to perform the tasks in the JSP pages without using the Java code. JSTL tags are divided into the Five types Formatting tags, Core tags, SQL tags, Function tags, and XML tags. Now we are discussing the Formatting tags.
The JSTL formatting tags are used for formatting data. It allows the developer to manipulate the data and display the data in different formats like numbers, currencies, and dates on the JSP pages.
Prerequisites:
The following are the prerequisites which are used in the JSTL formatting tags:
The Prerequisites for using the JSTL formatting tags are:
- Java Environment
- Servlet Container
- JSTL Library
- Taglib Declaration
The Syntax of the ‘taglib‘ directive is shown below:
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
In the above syntax,
- uri: It specified the URI of the tag library.
- prefix: It defines the prefix used to the reference tags from the library in the JSP page.
Note: By make sure that the above prerequisites will be met, you can easily use the JSTL formatting tags with the JSP pages for numbers, format dates, currencies and other data types according to the requirements of your application.
Example Project on JSTL Formatting tags
Below are the steps to implement JSTL Formatting Tags.
Step 1: Create a new dynamic web project in Eclipse or any preferred IDE.
Step 2: Add JSTL library in lib folder, as shown in below:
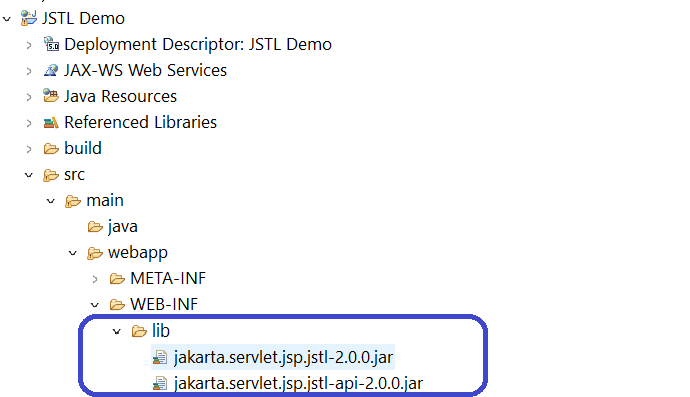
The above picture shows the path of JSTL library in lib folder.
Step 3: After adding libraries in lib folder, we can create JSP file in Webapp folder.
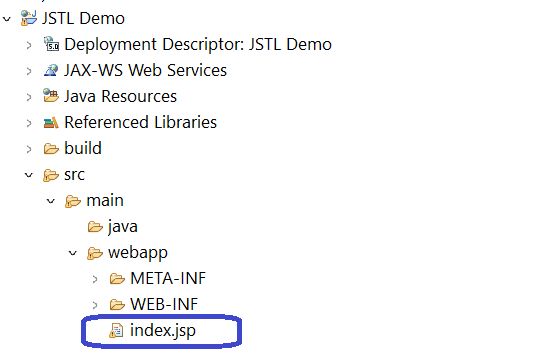
The above picture shows the path of JSP file in webapp folder.
Step 4: Now, we can write the JSP program in JSP file, which is named as index.jsp.
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<h1><title>Date Formatting Example</title><h2>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
color: #333;
margin: 0;
padding: 0;
}
.container {
max-width: 800px;
margin: 20px auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0,0,0,0.1);
}
p {
margin: 10px 0;
font-size: 18px;
}
strong {
font-weight: bold;
color: #007bff;
}
</style>
</head>
<body>
<%-- Assuming myDate is a java.util.Date object --%>
<fmt:formatDate value="${myDate}" pattern="yyyy-MM-dd HH:mm:ss" />
<fmt:formatDate type="time" value="${today}" />
<c:set var="today" value="<%=new java.util.Date()%>" />
<div class="container">
<p>Time: <strong><fmt:formatDate type="time" value="${today}" /></strong></p>
<p>Date: <strong><fmt:formatDate type="date" value="${today}" /></strong></p>
<p>Date & Time: <strong><fmt:formatDate type="both" value="${today}" /></strong></p>
<p>Date & Time Short: <strong><fmt:formatDate type="both" dateStyle="short" timeStyle="short" value="${today}" /></strong></p>
<p>Date & Time Medium: <strong><fmt:formatDate type="both" dateStyle="medium" timeStyle="medium" value="${today}" /></strong></p>
<p>Date & Time Long: <strong><fmt:formatDate type="both" dateStyle="long" timeStyle="long" value="${today}" /></strong></p>
<p>Date (yyyy-MM-dd): <strong><fmt:formatDate pattern="yyyy-MM-dd" value="${today}" /></strong></p>
</div>
<%-- Assuming myDate is a java.util.Date object --%>
<fmt:formatDate type="time" value="${today}" var="currentTime" />
<p align="center">Current Time: ${currentTime}</p>
</body>
</html>
Now, run the code on Run on Server. It will give the output in Browser as shown below:
Output:
Below is the Output screen, after entering the URL in browser.
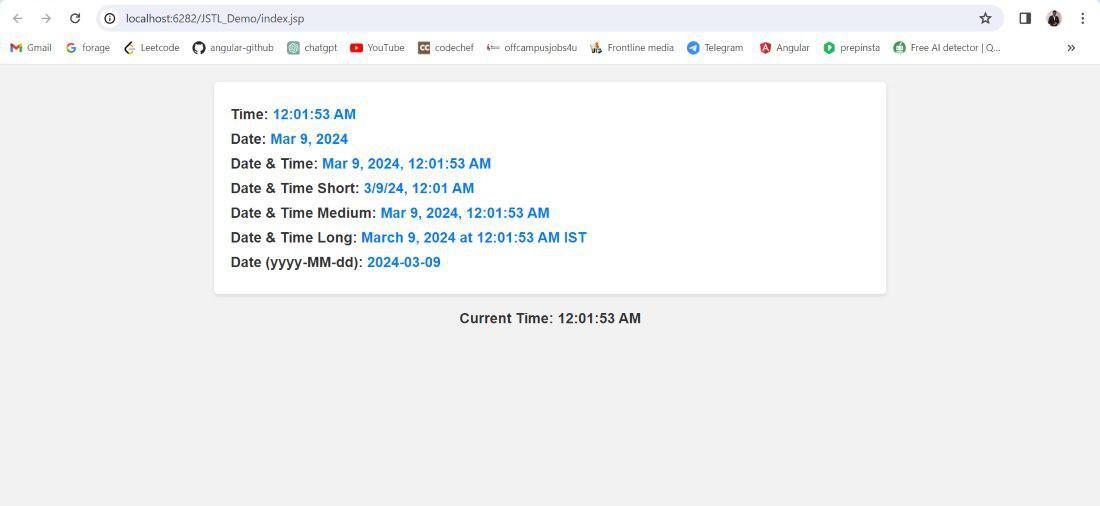
Share your thoughts in the comments
Please Login to comment...