JSTL Core <c:out> Tag
Last Updated :
13 Nov, 2023
Java Server Pages (JSP) is a technology used to create web applications with the popular programming language Java. With JSP we can write Java code inside a HTML page, making it easier to develop web applications. To enhance the feature set and usability of JSPs, Java Server Pages Standard Tag Library (JSTL) was introduced. By using JSTL, we can simply do the most common tasks with the help of predefined tags that enable us to do it in a much simpler way.
One of the JSTL core tags is <c:out>, which is used to display any value of a variable in the JSP page. It is similar to the <%= … %> but to use it with expressions. It uses XML escaping by default, which makes it more resistant to cross-site XSS attacks.
Prerequisites for the topic:
- Good understanding of Java EE web project developments like setting up a servlet or a JSP page with the help of any IDE
- Any IDE with support for Java EE development.
- An installation of Apache Tomcat web server or any other server that supports running Java-based web applications like GlassFish, Websphere, etc.
Syntax
<c:out value="expression" default="string" escapeXml="boolean" />
Attributes of JSTL <c:out> Tag
Expression to be evaluated
|
String
|
True
|
Default value will be used if the referenced value is null
|
String
|
False
|
Determines whether <>&, characters should be converted to char codes. Default is true
|
String
|
False
|
Usage of <c:out> tag
1. Displaying a statement or expression using value attribute
To display any statement of string just assign the string to the value attribute of <c:out> tag. Or else, we can also pass any valid expression to the value attribute to get evaluated and then print it on the jsp page. To use expressions, the expression has to be surrounded by a ${ expression_here }
Example 1:
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
< html >
< head >
< meta charset = "ISO-8859-1" >
< title >Insert title here</ title >
</ head >
< body >
< c:out value = "Hello world" />
</ body >
</ html >
|
Output:
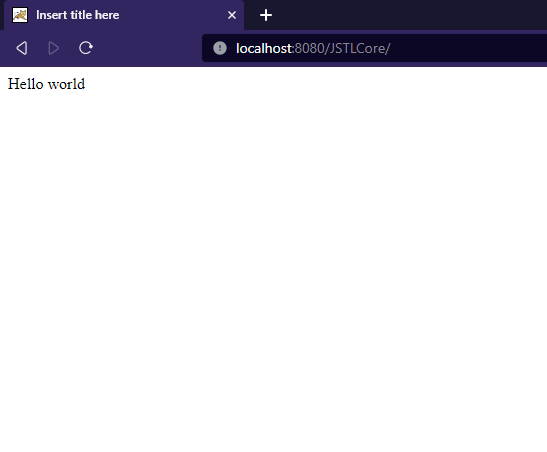
Example 2:
Here, a mathematical expression is being passed to the value attribute:
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
< html >
< head >
< meta charset = "ISO-8859-1" >
< title >Insert title here</ title >
</ head >
< body >
< c:out value = "${ 5 * 5 }" />
</ body >
</ html >
|
Output:
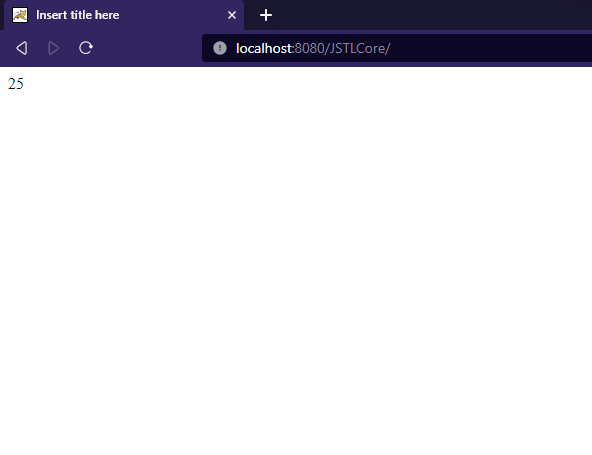
2. Displaying any variables
To display any variable that is already set in the jsp page, we have to pass the variable name as the argument for the value attribute. If the variable is null in that page, we can specify a default value to be printed using the default tag.
In the below example, we have set a variable in the jsp page using the <c:set> tag. As profession variable is present, the value is printed out as it is, but the below salary variable is not present in the page, so the default attribute string is used.
Example 1:
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
< html >
< head >
< meta charset = "ISO-8859-1" >
< title >Insert title here</ title >
</ head >
< body >
< c:set var = "profession" value = "programming" />
< c:out value = "My profession is ${profession}" />
< br >
< c:out value = "${salary}" default = "My salary is not found" />
</ body >
</ html >
|
Output:
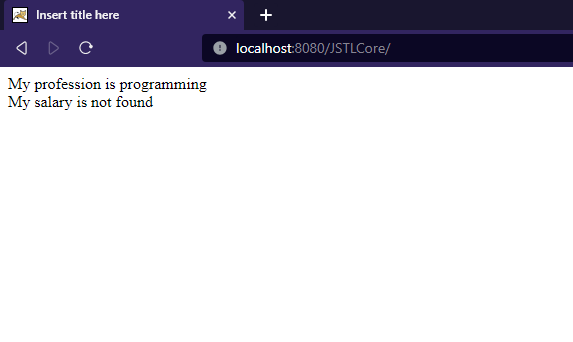
3. Escaping characters
While printing the value, by default it will not parse the symbols like <,>,&, ‘,’ . If we try to use a HTML or XML as value, it will just printed as characters.Also, it increase the security of the page, as cross-site XSS attacks can’t be done by injecting any script code.
To make it parse the HTML, we have to set the escapeXml to false, where it is true by default for security reasons.
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
< html >
< head >
< meta charset = "ISO-8859-1" >
< title >Insert title here</ title >
</ head >
< body >
< c:out value="<h1>Oops i'm not parsed as html :(</ h1 >" escapeXml="true" />
< c:out value="<h1>i'm parsed as html</ h1 >" escapeXml="false" />
</ body >
</ html >
|
Output:
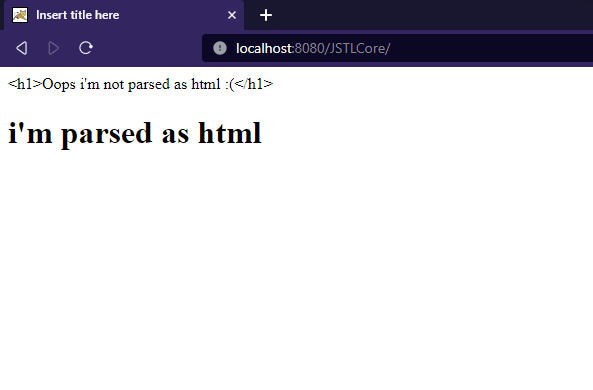
The below code has two different c:out tags with escapingXml enabled and disabled. If we setting the escapingXml to false, the code will be parsed as HTML,XML code and gets run as it is. This leads to attacks like cross-site xss, where any attacker can inject code like
<script>
sendUserData(sessionId) {
connect to hacker
send the sessionId
}
</script>
But, if we setting the escapingXml to true, which is actually the default one, the code will be just seen as string and won’t get executed at the client side.
Below is the implementation of the above lines explained:
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
< html >
< head >
< meta charset = "ISO-8859-1" >
< title >Insert title here</ title >
</ head >
< body >
< c:out value = "${userGeneratedContent}" escapeXml = "false" />
< c:out value = "${userGeneratedContent}" escapeXml = "true" />
</ body >
</ html >
|
Conclusion
The JSTL Core <c:out> tag is a powerful tool for displaying dynamic content in JSP without resorting to scriptlets. It offers features like HTML escaping and providing default values, making it a safer and more convenient choice for JSP development. By using the <c:out> tag, you can enhance the maintainability and security of your web applications while keeping your code clean and readable.
Share your thoughts in the comments
Please Login to comment...