JSON stands for JavaScript Object Notation. It is a format for structuring data. This format is used by different web applications to communicate with each other. In this article, we will learn about JSON pretty print
What is JSON?
JSON (JavaScript Object Notation) is a text-based data format that is interchangeable with many programming languages. It is commonly used for data transmission between client-server applications. Usually, minified versions of JSON text are transmitted to save bandwidth. However, for debugging and analysis, a beautified version or a pretty print JSON is required. Essentially, pretty print JSON means having proper indentation, white spaces, and separators.
Example:
Input:
'[ {"studentid": 1, "name": "ABC", "subjects": ["Python", "Data Structures"]}]'
Output:
[
{
"studentid": 1,
"name": "ABC",
"subjects":
[
"Python",
"Data Structures"
]
}
]
json.dumps() in Python
First, use json.loads() method to convert JSON String to Python object. To convert this object to a pretty print JSON string, the json.dumps() method is used. Below are examples and steps to better understand these cases.
Syntax: json.dumps(obj, indent,separator)
Parameter:
- obj: Serialize obj as a JSON formatted stream
- indent: If indent is a non-negative integer or string, then JSON array elements and object members will be pretty-printed with that indent level. An indent level of 0, negative, or “” will only insert newlines.
- separators : If specified, separators should be an (item_separator, key_separator) tuple.
Pretty Print JSON String
This method has the parameter indent to specify the number of spaces and a separator parameter to specify the separator between key and value. By default, the separator is a comma between key-value pairs and a colon between key and value. If the indent parameter of json.dumps() is negative, 0, or an empty string then there are no indentations and only newlines are inserted. By default, the indent is None and the data is represented in a single line.
The code takes a JSON string containing student records, parses it into a Python data structure, then pretty-prints the JSON data with proper indentation for improved readability.
Python3
import json
json_data = '[ { "studentid" : 1 , "name" : "ABC" , \
"subjects" : [ "Python" , "Data Structures" ]}, \
{ "studentid" : 2 , "name" : "PQR" ,\
"subjects" : [ "Java" , "Operating System" ]} ]'
obj = json.loads(json_data)
json_formatted_str = json.dumps(obj, indent = 4 )
print (json_formatted_str)
|
Output:
[
{
"studentid": 1,
"name": "ABC",
"subjects": [
"Python",
"Data Structures"
]
},
{
"studentid": 2,
"name": "PQR",
"subjects": [
"Java",
"Operating System"
]
}
]
Pretty-Printed JSON data into a file with indent=0.
The code takes a JSON string containing student records, parses it into a Python data structure, and then pretty-prints the JSON data with zero indentation, making it compact and less readable.
Python3
import json
json_data = '[ { "studentid" : 1 , "name" : "ABC" , \
"subjects" : [ "Python" , "Data Structures" ]},\
{ "studentid" : 2 , "name" : "PQR" , \
"subjects" : [ "Java" , "Operating System" ]} ]'
obj = json.loads(json_data)
json_formatted_str = json.dumps(obj, indent = 0 )
print (json_formatted_str)
|
Output:
[
{
"studentid": 1,
"name": "ABC",
"subjects": [
"Python",
"Data Structures"
]
},
{
"studentid": 2,
"name": "PQR",
"subjects": [
"Java",
"Operating System"
]
}
]
Write Pretty Print JSON data to file
To write a Python object as JSON Pretty Print format data into a file, json.dump() method is used. Like json.dumps() method, it has the indents and separator parameters to write beautified JSON.
Python3
import json
data = [{ "studentid" : 1 , "name" : "ABC" ,
"subjects" : [ "Python" , "Data Structures" ]},
{ "studentid" : 2 , "name" : "PQR" ,
"subjects" : [ "Java" , "Operating System" ]}]
with open ( "filename.json" , "w" ) as write_file:
json.dump(data, write_file, indent = 4 )
|
Output:
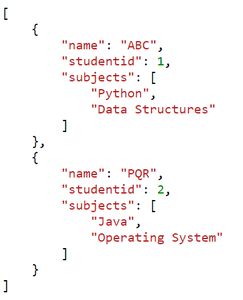
filename.json
Reading JSON data and pretty print it
To read JSON from a file or URL, use json.load(). Then use json.dumps() to convert the object (obtained from reading the file) into a pretty print JSON string.
Python3
import json
with open ( "filename.json" , "r" ) as read_file:
obj = json.load(read_file)
pretty_json = json.dumps(obj, indent = 4 )
print (pretty_json)
|
Output:
[
{
"studentid": 1,
"name": "ABC",
"subjects": [
"Python",
"Data Structures"
]
},
{
"studentid": 2,
"name": "PQR",
"subjects": [
"Java",
"Operating System"
]
}
]
Using pprint module to pretty-print JSON to print our JSON format
This code reads JSON data from a file called “test.json,” parses it into a Python data structure, and then prints it using both the built-in print
function and the pprint
module. The pprint
module is used to pretty-print the JSON data with specific formatting options like an indentation of 2, a line width of 30 characters, and compact representation.
Python3
import json
import pprint
with open ( "test.json" , "r" ) as json_data:
student = json.load(json_data)
print (student)
print ( "\n" )
pp = pprint.PrettyPrinter(indent = 2 , width = 30 , compact = True )
print ( "Pretty Printing using pprint module" )
pp.pprint(student)
|
Output:
{'Teacher_id': 1, 'name': 'Suraj', 'Salary': 50000, 'attendance': 80,
'Branch': ['English', 'Geometry', 'Physics', 'World History'], 'email': 'test@example.com'}
Pretty Printing using pprint module
("{'Teacher_id': 1, 'name': "
"'Suraj', 'Salary': 50000, "
"'attendance': 80, "
"'Branch': ['English', "
"'Geometry', 'Physics', "
"'World History'], 'email': "
"'test@example.com'}")
Pretty-print JSON from the command line
In this example, we are trying to print data using the command line. To validate and pretty-print JSON objects from the command line, Python offers the json.tool package.
Python3
echo { "studentid" : 1 , "name" : "ABC" ,
"subjects" : [ "Python" , "Data Structures" ]} | python - m json.tool
|
Output:
{
"studentid": 1,
"name": "ABC",
"subjects": [
"Python",
"Data Structures"
]
}
Share your thoughts in the comments
Please Login to comment...