jQuery | Traversing
Last Updated :
16 Mar, 2022
In jQuery, traversing means moving through or over the HTML elements to find, filter or select a particular or entire element.
Based on the traversing purposes following methods are Categorized as follows:
Tree Traversing:
Ancestors:
-
parent()
it gives parent element of specified selector
Syntax:
$(selector).parent();
-
parents()
it gives all ancestor elements of the specified selector.
Syntax:
$(selector).parents();
-
parentsUntil()
it gives all ancestor elements between specified selector and arguments.
Syntax:
$(selector).parentsUntil(selector, filter element)
$(selector).parentsUntil(element, filter element)
-
offsetParent()
it gives the first positioned parent element of specified selector.
Syntax:
$(selector).offsetParent();
-
closest()
it gives the first ancestor of the specified selector.
Syntax:
$(selector).closest(selector);
$(selector).closest(selector, context);
$(selector).closest(selection);
$(selector).closest(element);
Descendants:
Siblings:
-
siblings()
it gives all siblings of the specified selector.
Syntax:
$(selector).siblings();
-
next()
it gives the next sibling element of the specified selector.
Syntax:
$(selector).next();
-
nextAll()
it gives all next sibling elements of the specified selector.
Syntax:
$(selector).nextAll();
-
nextUntil()
it gives all next sibling elements between specified selector and arguments.
Syntax:
$(selector).nextUntil();
-
prev()
it gives the previous sibling element of the specified selector.
Syntax:
$(selector).prev(selector);
$(selector).prev()
-
prevAll()
it gives all previous sibling elements of the specified selector.
Syntax:
$(selector).prevAll(selector, filter element)
$(selector).prevAll(element, filter element)
-
prevUntil()
it gives all previous sibling elements between specified selector and arguments.
Syntax:
$(selector).prevUntil(selector, filter element)
$(selector).prevUntil(element, filter element)
Filtering
-
first()
it gives the first element of the specified selector.
Syntax:
$(selector).first();
-
last()
it gives the last element of the specified selector.
Syntax:
$(selector).last();
-
eq()
it gives an element with a specific index number of the specified selector.
Syntax:
$(selector).eq(index);
$(selector).eq( indexFromEnd );
-
filter()
it remove/detect an elements that are matched with specified selector.
Syntax:
$(selector).filter(selector)
$(selector).filter(function)
$(selector).filter(selection)
$(selector).filter(elements)
-
has()
it gives all elements that have one or more elements within, that are matched with specified selector.
Syntax:
$(selector).has(selector);
-
is()
it checks if one of the specified selector is matched with arguments.
Syntax:
.is( selector )
.is( function )
.is( selection )
.is( elements )
-
map()
Pass each element in the current matched set through a function, producing a new jQuery object containing the return values
Syntax:
.map( callback )
-
slice()
it selects a subset of specified selector based on its argument index or by start and stop value.
Syntax:
$(selector).slice(start, end );
$(selector).slice(start);
Miscellaneous Traversing
-
add()
it add all elements to set of matched elements to manipulate them at the same time.
Syntax:
$(selector).add(selector to add);
-
addBack()
it add the previous set of elements on the stack to the current set, optionally filtered by a selector.
Syntax:
$(selector).addBack();
-
andSelf()
Deprecated 1.8 which is alias of addBack().
Syntax:
$(selector).addSelf();
-
contents()
it gives all direct children, including text and comment nodes, of the specified selector.
Syntax:
$(selector).contents();
-
not()
it gives all elements that do not match with specified selector.
Syntax:
$(selector).not(selector);
-
end()
it is most recent filtering operation in the current chain and return the set of matched elements to its previous state and it doesn’t accept any arguments.
Syntax:
$(selector).each(callback function);
Collection Manipulation
-
each()
it iterates over the DOM elements and execute call back function
Example 1:
<!DOCTYPE html>
< html >
< head >
< style >
.siblings * {
display: block;
border: 2px solid lightgrey;
color: lightgrey;
padding: 5px;
margin: 15px;
}
</ style >
< script src =
</ script >
< script >
$(document).ready(function() {
$("h2").siblings().css({
"color": "red",
"border": "2px solid red"
});
$("h2").parent().css({
"color": "green",
"border": "2px solid blue"
});
$("p").first().css(
"background-color", "yellow");
$("p").has("span").css(
"background-color", "indigo");
});
</ script >
</ head >
< body class = "siblings" >
< div >GeeksforGeeks (parent)
< p >GeeksforGeeks</ p >
< p >< span >GeeksforGeeks</ span ></ p >
< h2 >GeeksforGeeks</ h2 >
< h3 >GeeksforGeeks</ h3 >
< p >GeeksforGeeks</ p >
</ div >
</ body >
</ html >
|
Output:
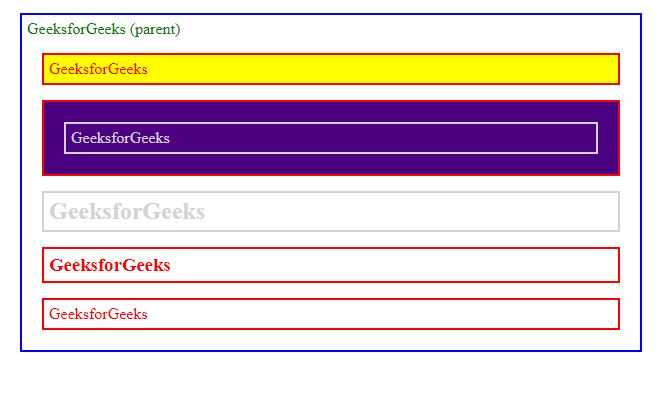
Example 2:
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" >
< style >
p {
color: grey;
margin: 10px;
padding: 10px;
}
form {
margin: 10px;
padding: 10px;
}
#article b {
color: blue;
font-weight: bold;
}
label {
color: green;
font-weight: bold;
}
</ style >
< script src =
</ script >
</ head >
< body >
< p >< em >Hello</ em >GeeksforGeeks</ p >
< p id = "article" >< b >Article Info: </ b ></ p >
< form >
< label >Article Title:</ label >
< input type = "text"
name = "article"
value="jQuery |
Traversing Example 2" />
< br >
< br >
< label >Author:</ label >
< input type = "text"
name = "author"
value = "Vignesh" />
< br >
< br >
< label >Author's Email id:</ label >
< input type = "text"
name = "author"
value = "vignesh@gmail.com" />
< br >
< br >
< label >Website:</ label >
< input type = "text"
name = "url"
< br >
< br >
</ form >
< script >
$("#article")
.append($("input").map(function() {
return $(this).val();
})
.get()
.join(", "));
</ script >
< script >
$("p")
.find("em")
.end()
.css("border", "2px red solid");
</ script >
</ body >
</ html >
|
Output
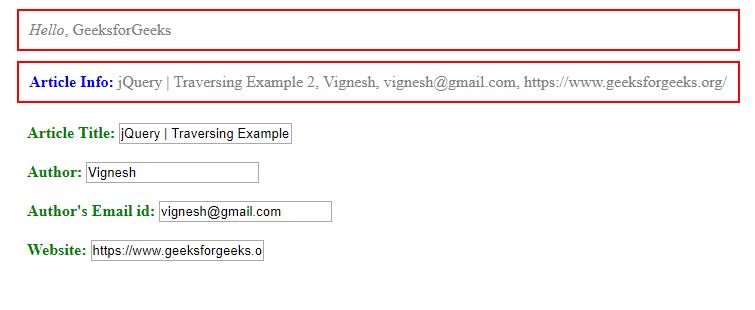
Share your thoughts in the comments
Please Login to comment...