Java WindowListener in AWT
Last Updated :
27 Dec, 2023
The Abstract Window Toolkit (AWT) of Java provides a collection of graphical user interface (GUI) components for creating desktop applications. When it comes to managing window-related events, the WindowListener interface is used. The WindowListener interface provides methods for responding to window-related events such as opening, closing, activating or deactivating, and more such methods.
WindowListener in AWT
The WindowListener interface in Java AWT is imported from the java.awt.event package. It is the basic connection or a link between your application and the window’s actions. By implementing this interface and overriding its methods in your class, you can respond to a wide range of window events.
Syntax of WindowListener:
public interface WindowListener extends EventListener
The WindowListener interface in Java is declared with the above statement. It extends the EventListener interface which indicates that it helps in capturing and reacting to different window events in Java applications.
WindowListener Interface Methods
Given below are the abstract methods of the WindowListener interface.
void
|
This method is called when the window is configured to be the active window.
|
void
|
Triggered upon the closing of a window as an outcome of calling dispose on the window.
|
void
|
Invoked whenever a user tries to dismiss a window via the system menu.
|
void
|
Called when a window isn’t the active window anymore.
|
void
|
Called when the conversion of a window from its minimized to its regular state.
|
void
|
Triggered when there is a transition of a window from its normal minimized state.
|
void
|
It is called the first time a window appears.
|
Note: Methods of the WindowListener interface are inherited from the EventListener interface.
Example of Java WindowListener
Given below is a simple example to demonstrate the working of the WindowListener interface.
Java
import java.awt.*;
import java.awt.event.*;
import java.awt.event.WindowListener;
public class Window implements WindowListener {
public Window()
{
Frame f = new Frame( "WindowListener Example" );
Label l = new Label( "GeeksforGeeks" );
l.setBounds( 100 , 90 , 140 , 20 );
l.setForeground(Color.GREEN);
l.setFont( new Font( "Serif" , Font.BOLD, 22 ));
f.add(l);
f.addWindowListener( this );
f.setSize( 400 , 300 );
f.setLayout( null );
f.setVisible( true );
}
public void windowOpened(WindowEvent e)
{
System.out.println( "Window is opened!" );
}
public void windowClosing(WindowEvent e)
{
System.out.println( "Window is closing..." );
System.exit( 0 );
}
public void windowClosed(WindowEvent e)
{
System.out.println( "Window is closed!" );
}
public void windowIconified(WindowEvent e)
{
System.out.println( "Window is iconified!" );
}
public void windowDeiconified(WindowEvent e)
{
System.out.println( "Window is deiconified!" );
}
public void windowActivated(WindowEvent e)
{
System.out.println( "Window is activated!" );
}
public void windowDeactivated(WindowEvent e)
{
System.out.println( "Window is deactivated!" );
}
public static void main(String[] args) { new Window(); }
}
|
Use below commands to run the program,
javac Window.java
java Window
Output of the above code in GIF:
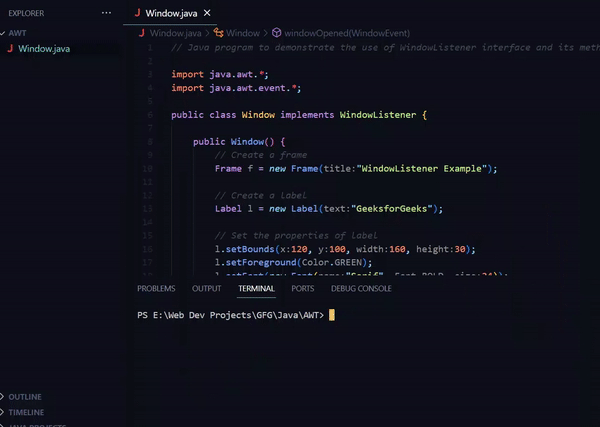
Explanation of the example:
- In the code given above, a simple frame or a window is created with a label in it.
- The class Window implements the WindowListener interface. In this class, all methods of the interface are overridden.
- When each of these window event gets triggered, the overridden methods output messages to the console.
- For example, it prints ‘Window is opened!’ when the window is opened and ‘Window is closing…’ when the user attempts to close the window.
Applications of the WindowListener Interface
There are many use cases of WindowListener interface, which we can use in our Java application to perform different actions.
- Confirmation on Exit: Before application get terminated, you may ask the user for confirmation by using the windowClosing method.
- Saving Data: As the window closes, you may want to save any unsaved configurations or data.
- Dynamic UI Updates: When the window is enabled or deactivated, certain actions, such as UI updating or data refreshing, can be initiated.
- Media Player Interactions: When a user navigates to another window or program in a media player, the windowDeactivated event can be used to trigger an automated halt in playing.
- Status Updates: The windowDeactivated event in a chat application might be used to have the user’s status changed to “Away” or “Inactive” if they go to another program.
Share your thoughts in the comments
Please Login to comment...