Rule engines in Java provide us with a framework for managing and performing business rules in a flexible and defining manner. These engines allow the developers to separate the business logic from the application code by making it easier to modify and understand rules without changing the core application.
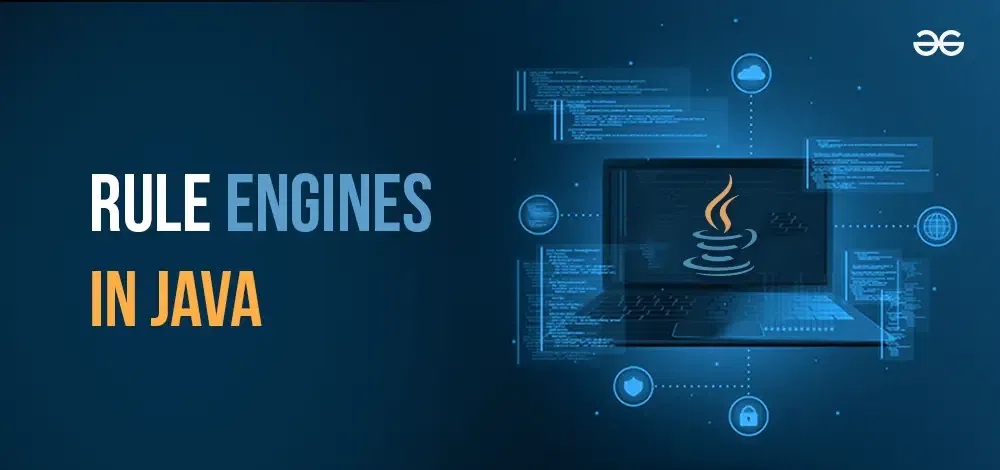
Rule engines are designed to evaluate the conditions and trigger the actions based on specified rules. They also provide us with a more dynamic and adaptable approach to handling complex decision-making processes. This article introduces various rule engines that allow developers to automate their software application logic. By integrating these rule engines, developers can significantly contribute to the automation of business policies, ultimately resulting in more robust and effective software applications.
What is a Rule Engine in Java?
Rule engines in Java are indeed powerful tools that automate business logic, enabling more efficient decision-making processes. These engines allow developers to define and manage business rules separately from the application code, providing flexible conditions and actions that allow for a dynamic decision-making approach to handling complex decision scenarios. The separation of business rules from the core application logic contributes to better code organization, reusability, and ease of maintenance.
Benefits of Rule Engine:
- It provides us with great reusability of the code.
- It reduces complexity by eliminating the need for direct manipulation of the source code, as rule engines operate independently of the associated application code.
- It also provides readability and flexibility where we can change the rules without changing anything in the source code.
- It allows the separation of business rules from the application code without affecting the core programs.
- It helps in the collaboration of business experts and developers without knowing advanced coding skills which make the decisions very effective and logical.
How Does the Rule Engine work?
Step 1: Set Up Your Java Project
To set up a Java project, you need to create a new Java project or can also use an existing project. Before you can implement rule engines in your Java project, you have to ready a Java project with required configured settings in your Java project. If you don’t have an existing Java project then you can also follow these steps to create the new one.
- Open your Integrated Development Environment (IDE).
- Create a new project.
- Configure project settings by ensuring the appropriate Java version for your project.
- Create a package in your ‘src’ folder.
- Create a Class which can be denoted by any name like ‘Main’ or ‘RuleEngineExample’, and serves as an entry point for the project.
- Write a simple Java code snippet.
- Save and Build.
Step 2: Add Rule Engine tools dependencies in your pom.xml file or yml file.
For Example, adding dependency for Drools engine in your xml file, if you are using Maven.
XML
< dependencies >
< dependency >
< groupId >org.drools</ groupId >
< artifactId >drools-core</ artifactId >
< version >7.65.0.Final</ version >
</ dependency >
</ dependencies >
|
Step 3: Create a Fact class
The Fact class which refers to the class that represents the data or facts upon which the rules will be applied, in the following example the ‘Person’ class is considered a Fact Class. Generally in the rule engine, especially in the case of Drools rule engine, the class.java is replaced with the fact class and normally represented as a class.java or person.java.
Java
public class Person {
private String name;
private int age;
private boolean eligibleForDiscount;
private boolean specialStatus;
}
|
Step 4: Write a Rule in your Java project
Here we are creating a rule file for the Drools rule engine named ‘GeeksRule.drl’, where ‘drl’ is the extension for the Drools rule.
Java
rule "GeeksforGeeks Rule"
when
$person: Person(age < 30 , name.contains( "GeeksforGeeks" ))
then
$person.setSpecialStatus( true );
end
|
‘GeeksforGeeks’ rule is the name assigned to this rule, it serves as a label for identification and readability and the ‘end’ mark is represented as the end of that particular rule. This rule states that if a person’s age is less than 30 and their name contains “GeeksforGeeks”, they are granted a special status.
Step 5: Use Drools in Your Java Code
Create a Java class to use Drools to evaluate the GeeksforGeeks rule.
Java
import org.kie.api.*;
import org.kie.api.runtime.*;
public class RuleEngineExample {
public static void main(String[] args) {
KieServices kieServices = KieServices.Factory.get();
KieContainer kieContainer = kieServices.getKieClasspathContainer();
KieBase kieBase = kieContainer.getKieBase();
KieSession kieSession = kieBase.newKieSession();
Person person = new Person( "GeeksforGeeks User" , 25 );
kieSession.insert(person);
int ruleFiredCount = kieSession.fireAllRules();
System.out.println( "Rules fired: " + ruleFiredCount);
System.out.println( "Is person eligible for a special status? " + person.isSpecialStatus());
kieSession.dispose();
}
}
|
Step 6: Run your Java project
Run your Java project, and you should see output indicating whether the GeeksforGeeks rule was fired and whether the person is eligible for a special status based on the provided name and age. The above example demonstrates how you can use Drools to apply rules related to GeeksforGeeks within a Java project. Adjust the rule and data objects as needed for your specific use case.
Popular Rule Engines in Java
1. Drools
Drools rule engine is widely known as the ‘Business Rules Management System’ (BRMS) which is widely used as an open-source rule engine for Java, it helps in encouraging the developers to implement and manage the such complex business logics.With exclusive functionalities, Drools is the preferred choice of the Java developers for rule based software applications in the diverse domains.
Example:
Java
rule "Example Rule"
when
$fact: SomeObject(property > 10 )
then
$fact.setFlag( true );
end
|
2. Jess
Jess is a rule engine which is designed for the Java platform, and frequently used in Java applications while developing the expert systems. Its user-friendly scripting language and robust reasoning engine make it well suited for the complex decision making, and helps in establishing it as a valuable choice for such tasks which requires the advanced rule based systems in the Java applications.
Example:
Java
(defrule example-rule
(some-object (property > 10 ))
=>
(modify some-object (set-flag true )))
|
3. Easy Rules
Easy Rules, recognized as a lightweight and straightforward rule engine for Java, stands out in providing rule abstraction for creating the rules based on defined actions and conditions. Its simplicity and flexibility make it a preferred choice for developers seeking an uncomplicated yet effective solution for implementing and managing the rules in Java applications, and facilitating a seamless integration of business logic in the application.
Example:
Java
Rule someRule = new BasicRuleBuilder()
.name( "Example Rule" )
.when(facts -> facts.get( "property" , Integer. class ) > 10 )
.then(facts -> facts.put( "flag" , true ))
.build();
|
4. Rule Book
Rule Book offers a user-friendly and flexible DSL which makes its rule creation straightforward. Whereas, for the larger rule collections, developers can smoothly integrate the rules into the annotated POJOs class. The RuleBook efficiently transforms this package into an organised rule book that simplifies the organisation and management of diverse rules in Java applications.
Example:
Java
public class DelftstackRule {
public RuleBook<Object> defineDelftstackRules() {
return RuleBookBuilder.create()
.addRule(rule -> rule.withNoSpecifiedFactType().then(f -> System.out.print( "Delftstack" )))
.addRule(rule
-> rule.withNoSpecifiedFactType().then(
f -> System.out.println( "The Best Tutorial Site" )))
.build();
}
}
|
5. OpenL Tablets
OpenL Tablets is an open source business rules and decision management system for Java which serves as an integral component in the both business rule engines and the management systems. Its adaptability and extensibility make it a valuable tool for the developers who seek for robust solutions to implement, organise, and manage their business rules effectively in the Java applications, it also ensures the efficient decision making processes.
Example:
Java
public class Rules {
public boolean exampleRule( int property) {
return property > 10 ;
}
}
|
6. Eclipse OCL (Object Constraint Language)
Eclipse OCL (Object Constraint Language) goes beyond being a rule engine as it’s a specialised language which is designed for expressing the rules on objects within UML models. Its distinctive capability enables the developers to precisely define and enforce the rules and shapes the behaviour and relationships of the objects in their Java applications, also making the Eclipse OCL a valuable asset for ensuring accuracy and compliance with the specified criteria in such complex application scenarios.
Example:
Java
context SomeObject
inv: self.property > 10
|
7. Apache Jena Rules
Apache Jena rules is a Java framework for constructing the semantic web and linked data applications that showcase the powerful rule engine which is designed for handling the RDF data. This framework provides developers with robust tools to navigate, query, and deduce the relationships within RDF datasets, also making it an essential asset for those who are engaged in the development of advanced applications involving semantic web technologies.
Example:
Java
String ruleString = "[r1: (?x ex:property ?y) -> (?x ex:flag true)]" ;
Reasoner reasoner = new GenericRuleReasoner(Rule.parseRules(ruleString));
|
8. Nools
Nools is recognized as a rule engine for Node.js which effortlessly extends its utility to Java through the assistance of the Nashorn JavaScript engine which is included in the Java Development Kit (JDK) starting from Java 8. This compatibility enhances developers ability to utilise the Nools’ rule based capabilities, and it’s originally designed for Node.js, within Java applications, and this rule offers a flexible solution for implementing and managing rules across the different environments.
Example:
Java
define SomeRule {
when {
$s: SomeObject $s.property > 10
}
then {
$s.flag = true ;
}
}
|
Conclusion
In Conclusion, this article has discussed why Java Rules Engines are important as we have learned how they help make business things work automatically and decide things faster. These engines allows developers to handle rules on their own, making the code flexible and easy to taken care of together. The step-by-step guide with Drools is like a hands-on lesson, and looking at different engines, like Drools, Jess, Easy Rules, Rule Book, OpenL Tablets, Eclipse OCL, Apache Jena Rules, and Nools, shows there are many choices. Using rule engines is like giving software the ability to adjust and stay strong which makes them really useful for today’s Java development.
Share your thoughts in the comments
Please Login to comment...