Java JEditorPane
Last Updated :
23 Oct, 2023
A JEditorPane is a component in Java Swing that provides a simple way to display and edit HTML and RTF (Rich Text Format) content. It is a versatile component that allows you to display styled text, links, and images, making it useful for creating simple web browsers, rich-text editors, and content viewers within your Java applications. JEditorPane is a part of javax.swing package.
Commonly used constructors
Creates a JEditorPane with no specified content type
|
Creates a JEditorPane to display the content from the specified URL. The content type is determined based on the URL’s file extension
|
Creates a JEditorPane with the specified content type and initial text
|
Creates a JEditorPane with the specified content type and displays the content from the specified URL
|
Some Methods of the JEditorPane
Sets the text content of the editor pane.
|
Sets the page content from the specified URL. The content type is determined based on the URL’s file extension.
|
Sets the content type of the editor pane (e.g., “text/plain,” “text/html,” “text/rtf”).
|
Sets the editor kit used to interpret and display the content.
|
Returns the document model associated with the editor pane.
|
Returns the content type of the editor pane.
|
Returns the default editor kit for the specified content type.
|
Returns the caret (cursor) used for text editing.
|
Sets whether the editor pane is editable or not.
|
Returns the currently selected text.
|
Following are the programs to implement JEditorPane
Example 1:
Java
import javax.swing.*;
public class JEditorPaneExample {
public static void main(String[] args)
{
SwingUtilities.invokeLater(() -> {
JFrame frame
= new JFrame( "JEditorPane Example" );
frame.setDefaultCloseOperation(
JFrame.EXIT_ON_CLOSE);
frame.setSize( 400 , 300 );
JEditorPane editorPane = new JEditorPane();
editorPane.setContentType( "text/html" );
String htmlContent
= "<html><body><h1>GeeksforGeeks</body></html>" ;
editorPane.setText(htmlContent);
frame.add(editorPane);
frame.setVisible( true );
});
}
}
|
Output:

Example 2:
Java
import javax.swing.*;
public class JEditorPaneExample {
public static void main(String[] args)
{
SwingUtilities.invokeLater(() -> {
JFrame frame
= new JFrame( "JEditorPane Example" );
frame.setDefaultCloseOperation(
JFrame.EXIT_ON_CLOSE);
frame.setSize( 400 , 300 );
JEditorPane editorPane = new JEditorPane();
editorPane.setContentType( "text/rtf" );
String rtfContent
= "{\\rtf1\\ansi\\deff0 {\\fonttbl {\\f0 Times New Roman;}} {\\colortbl ;\\red0\\green0\\blue255; } GeeksforGeeks}\n" ;
editorPane.setText(rtfContent);
frame.add(editorPane);
frame.setVisible( true );
});
}
}
|
Output:
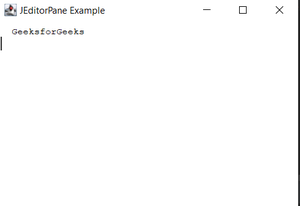
Example 3:
Java
import java.awt.*;
import javax.swing.*;
public class JEditorPaneSetFontExample {
public static void main(String[] args)
{
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame(
"JEditorPane setFont() Example" );
frame.setDefaultCloseOperation(
JFrame.EXIT_ON_CLOSE);
frame.setSize( 400 , 300 );
JEditorPane editorPane = new JEditorPane();
editorPane.setContentType( "text/plain" );
editorPane.setText( "GEEKSFORGEEKS" );
Font customFont
= new Font( "Serif" , Font.BOLD, 16 );
editorPane.setFont(customFont);
frame.add(editorPane);
frame.setVisible( true );
});
}
}
|
Output:
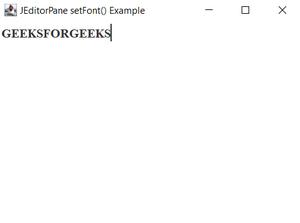
Share your thoughts in the comments
Please Login to comment...