Java AWT TextField
Last Updated :
26 Nov, 2023
Java Abstract Window Toolkit (AWT) is a library for building interactive Graphical User Interfaces (GUIs) in Java Applications. In AWT, TextField is a text component that lets users add a single line of text and edit it further.
TextField in Java AWT
The TextField class in Java AWT is a GUI component in the ‘java.awt’ package. It allows the user to enter a single line of text as an input. It is a simple way to collect text-based information from the user. You can use the TextField component to create various input fields for username, password, search boxes, etc.
Whenever a key is pressed or typed into the input field, an event is sent to the TextField. The registered KeyListener then receives the KeyEvent. ActionEvent can also be used for this purpose. If the ActionEvent is configured on the text field, then it will be triggered when the Return key is pressed. The ActionListener interface handles the event.
Syntax of Java AWT TextField
public class TextField extends TextComponent
Class Constructors of AWT TextField
- TextField(): Constructs a new text field component
- TextField(int columns): Constructs a new empty text field with the required number of columns as the parameter.
- TextField(String text): Creates a new text field initialized with the string text to be displayed.
- TextField(String text, int columns): Creates a new text field with the given text to be displayed and a width that fits the specified number of columns.
Class Methods of AWT TextField
A list of all the methods associated with TextField with its description is mentioned below in the table:
void addActionListener(ActionListener l)
|
This action listener is added to receive action events from this text field.
|
void addNotify()
|
Creates a peer for the TextField.
|
boolean echoCharIsSet()
|
Check if there is a character set for echoing in this text field.
|
AccessibleContext getAccessibleContext()
|
Retrieves the AccessibleContext associated with the TextField.
|
ActionListener[] getActionListeners()
|
An array of all action listeners registered on a textfield is returned.
|
int getColumns()
|
Returns the number of columns in a text field.
|
char getEchoChar()
|
Returns the character to be used for echoing.
|
Dimension getMinimumSize()
|
It returns the minimum dimensions for a text field.
|
Dimension getMinimumSize(int columns)
|
Fetches the minimum dimensions for a text field with the specified number of columns.
|
Dimension getPreferredSize()
|
Returns the preferred size of a text field.
|
Dimension getPreferredSize(int columns)
|
It returns the preferred size of a text field with specified number of columns.
|
protected String paramString()
|
Returns a string indicating the state of this TextField.
|
protected void processActionEvent(ActionEvent e)
|
Action events that occur on the text field are processed by dispatching them to any registered ActionListener objects.
|
protected void processEvent(AWTEvent e)
|
Processes an event on the text field.
|
void removeActionListener(ActionListener l)
|
It removes the specified action listener from the text field..
|
void setColumns(int columns)
|
Defines the number of columns in the text field.
|
void setEchoChar(char c)
|
Defines echo character for the text field.
|
void setText(String t)
|
Sets the text that is presented by this text component to be the specified text.
|
Inherited Methods
The Methods included with AWT TextField are inherited by:
- java.awt.TextComponent
- java.lang.Component
- java.lang.Object
Deomnstration of AWT TextField
Given below are some examples which will help you to easily understand about the TextField component.
Example 1:
Java
import java.awt.*;
public class Main {
public static void main(String[] args){
Frame f = new Frame( "TextField Example" );
TextField t1 = new TextField();
t1.setBounds( 50 , 50 , 200 , 30 );
TextField t2 = new TextField();
t2.setBounds( 50 , 100 , 200 , 30 );
f.add(t1);
f.add(t2);
f.setSize( 300 , 200 );
f.setLayout( null );
f.setVisible( true );
}
}
|
Run the code using the following commands:
javac Main.java
java Main
Output:
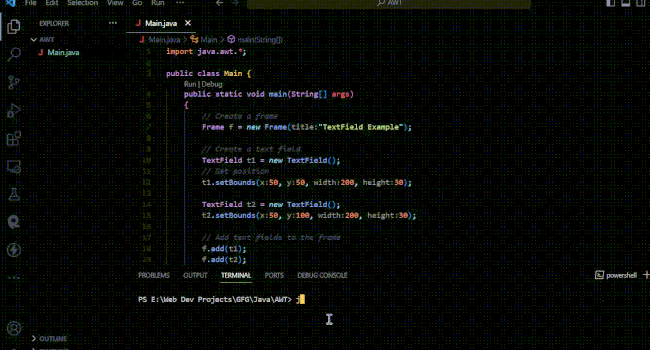
Final Output Created:
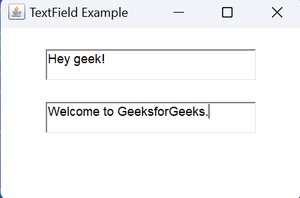
Example 2:
Java
import java.awt.*;
import java.awt.event.*;
public class Main2 {
public static void main(String[] args)
{
Frame f = new Frame( "TextField Example" );
Button b = new Button( "Submit" );
b.setBounds( 160 , 100 , 100 , 30 );
TextField t = new TextField();
t.setBounds( 160 , 50 , 200 , 20 );
Label l1 = new Label( "Name" );
Label l2 = new Label( " " );
l1.setBounds( 40 , 55 , 100 , 14 );
l2.setBounds( 150 , 170 , 400 , 14 );
f.add(b);
f.add(t);
f.add(l1);
f.add(l2);
b.addActionListener( new ActionListener() {
public void actionPerformed(ActionEvent e)
{
l2.setText( "Name: " + t.getText());
}
});
f.setSize( 400 , 300 );
f.setLayout( null );
f.setVisible( true );
}
}
|
Run the code using the following commands:
javac Main.java
java Main
Output :
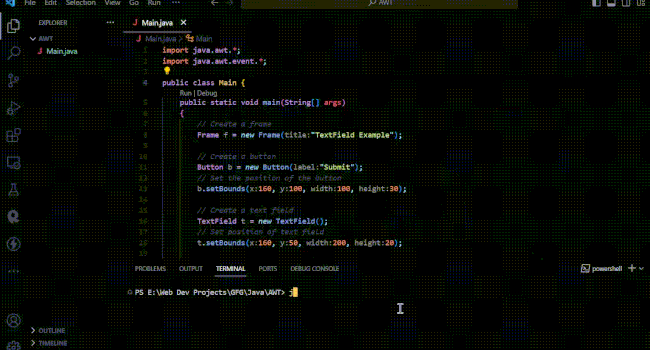
Final Output Created:
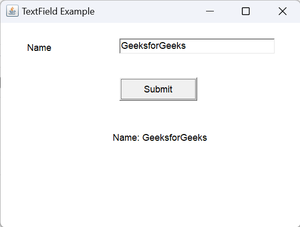
Share your thoughts in the comments
Please Login to comment...