Java AWT MenuItem & Menu
Last Updated :
13 Nov, 2023
Java Abstract Window Toolkit (AWT) provides a comprehensive set of classes and methods to create graphical user interfaces. In this article, we will explore the MenuItem and Menu classes, which are essential for building menus in Java applications.
Java AWT MenuItem
MenuItem is a class that represents a simple labeled menu item within a menu. It can be added to a menu using the Menu.add(MenuItem mi) method.
Syntax of Class Declaration MenuItem:
public class MenuItem extends MenuComponent implements Accessible
Methods of the MenuItem Class
List of methods available in MenuItem with description.
Constructs a new MenuItem with the specified label.
|
Returns the label of the menu item.
|
Sets the label of the menu item to the specified string.
|
Adds an action listener to the menu item.
|
Java AWT Menu Class
The Java AWT Menu class represents a pop-up menu component in a graphical user interface (GUI) that can contain a collection of MenuItem objects. It provides a way to create and manage menus in Java AWT applications.
Syntax of Class Declaration of Menu
public class Menu extends MenuItem
Methods of the Menu Class
List of methods available in MenuItem with description
Constructs a new menu with the specified label.
|
Adds the specified menu item to the menu.
|
Adds a separator between menu items within the menu.
|
Returns the menu shortcut key associated with this menu.
|
Example usage of the MenuItem & Menu
Java
import java.awt.*;
import java.awt.event.*;
public class MenuExample {
public static void main(String[] args) {
Frame frame = new Frame( "Menu Example" );
MenuBar menuBar = new MenuBar();
frame.setMenuBar(menuBar);
Menu fileMenu = new Menu( "File" );
MenuItem openItem = new MenuItem( "Open" );
MenuItem saveItem = new MenuItem( "Save" );
fileMenu.add(openItem);
fileMenu.add(saveItem);
fileMenu.addSeparator();
MenuItem exitItem = new MenuItem( "Exit" );
exitItem.addActionListener( new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.exit( 0 );
}
});
fileMenu.add(exitItem);
menuBar.add(fileMenu);
frame.setSize( 300 , 300 );
frame.setVisible( true );
}
}
|
This code creates a simple window with a “File” menu that contains “Open,” “Save,” and “Exit” options. When you click “Exit,” the application exits.
Output:
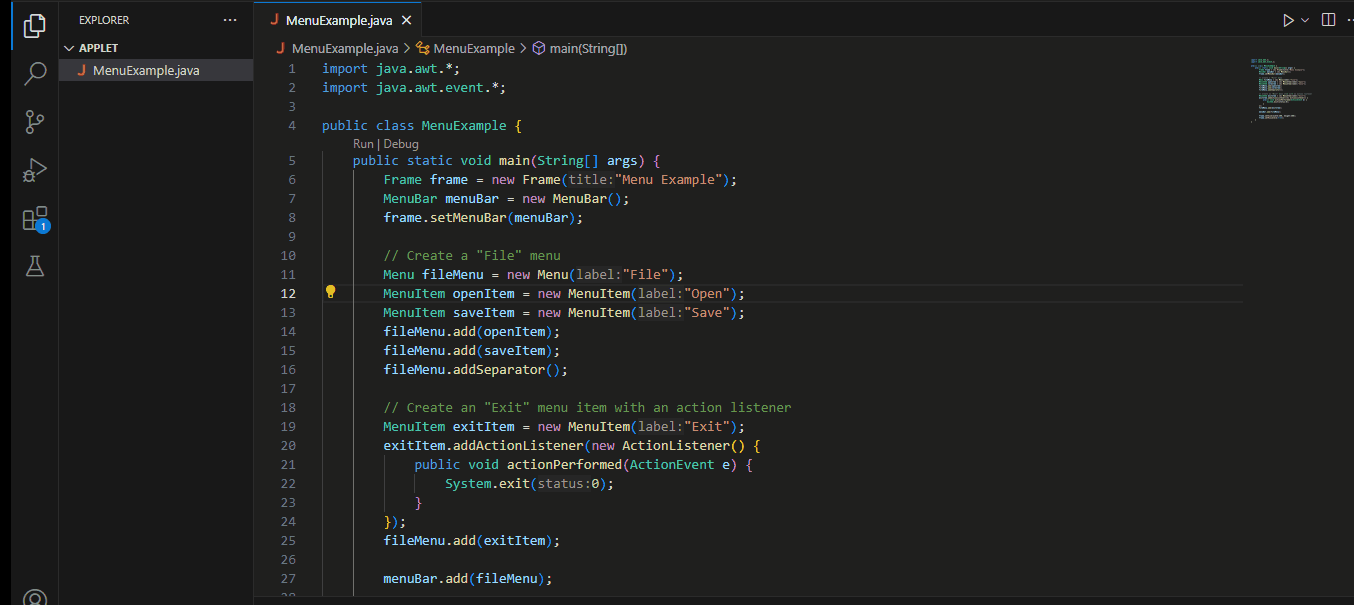
Output of the Example:
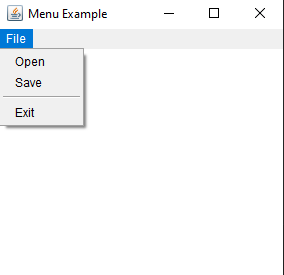
Conclusion
In this article, we explored the very necessary components of Java AWT’s MenuItem and Menu classes.
- These classes are material for creating menus in graphical user interfaces.
- We discussed the phrase structure of class declarations,
- Registered the methods with their descriptions.
- Introduced the constructors for some classes.
By combining these classes and their joint methods, you can build interactive and user-friendly menus for your Java applications. The provided code example demonstrated how to make a simpleton fare bar, offer a practical starting point for development of more complex graphical interfaces in Java.
Share your thoughts in the comments
Please Login to comment...