Java AWT List
Last Updated :
26 Nov, 2023
AWT or Abstract Window Toolkit is a set of various UI components with the List Component. We can display a scrollable list of items through which users can select one or multiple options. AWT List is embedded into the AWT library which is used to build a GUI to present a set of choices to the user. We can customize this by adding or removing the items dynamically from the list. In this article, we will the Declaration of AWT List, Constructors, Methods, and some practical examples for creating LIst with various logics.
Java AWT List Class Declaration
The List Class in Java extends the Component class and also implements the ItemSelectable and Accessible intergaves. This allows to representation of a selectable list of some options in UI form and also the feature for accessing the options.
Syntax:
public class List extends Component implements ItemSelectable, Accessible
Constructors of List Class
The table provides the constructors present in the List along with their descriptions.
List()
|
Used to create an empty list with no visible items.
|
List(int numRows)
|
Used to create an empty list for the given number of visible rows.
|
List(int numRows, boolean multipleMode)
|
Used to create an empty list for the given number of visible rows and set whether multiple selections are allowed (multipleMode).
|
Methods of List class are inherited by two classes:
- java.awt.Component
- java.lang.Object
Methods of List Class
The table provides the Methods present in the List along with its description.
void add(String item)
|
Adds the specified item to the end of the list.
|
void add(String item, int index)
|
Used to insert the given item to the given index in the list.
|
void addActionListener(ActionListener l)
|
Registers an ActionListener to listen for item selection events in the list.
|
void addItemListener(ItemListener l)
|
Registers an ItemListener to listen for item selection events in the list.
|
void addNotify()
|
Creates the peer of the list, if it doesn’t already exist.
|
void deselect(int index)
|
Deselects the item at the specified index in a multiple-selection list.
|
AccessibleContext getAccessibleContext()
|
Gets the AccessibleContext associated with this List.
|
ActionListener[] getActionListeners()
|
Used to return an array of all the ActionListeners added to this list.
|
String getItem(int index)
|
Retrieves the item at the specified index in the list.
|
int getItemCount()
|
Returns length(the number of items) in the list.
|
ItemListener[] getItemListeners()
|
Used to return an array of all the ItemListeners added to this list.
|
String[] getItems()
|
Used to return an array of all the items in the list.
|
Dimension getMinimumSize()
|
Used to return the minimum size of the list.
|
Dimension getMinimumSize(int rows)
|
Used to return the minimum size needed to display the specified number of rows.
|
Dimension getPreferredSize()
|
Used to return the preferred size of the list.
|
Dimension getPreferredSize(int rows)
|
Used to return the preferred size needed to display the specified number of rows.
|
int getRows()
|
Used to return the number of rows present in the list.
|
int getSelectedIndex()
|
Returns the index of the first selected item in a multiple-selection list.
|
int[] getSelectedIndexes()
|
Returns an array of the selected item indexes in a multiple-selection list.
|
String getSelectedItem()
|
Returns the selected item in a single-selection list.
|
String[] getSelectedItems()
|
Returns an array of the selected item values in a multiple-selection list.
|
Object[] getSelectedObjects()
|
Returns an array of selected items in a multiple-selection list.
|
int getVisibleIndex()
|
Returns the index of the first visible item in the list.
|
void makeVisible(int index)
|
Scrolls the list to make the item at the given index visible.
|
boolean isIndexSelected(int index)
|
Checks if the item at the given index is selected in a multiple-selection list.
|
boolean isMultipleMode()
|
Check if the list allows multiple selections.
|
protected String paramString()
|
Returns a string representing the state of this list.
|
protected void processActionEvent(ActionEvent e)
|
Processes action events occurring in this list.
|
protected void processEvent(AWTEvent e)
|
Processes events occurring in this list.
|
protected void processItemEvent(ItemEvent e)
|
Processes item events occurring in this list.
|
void removeActionListener(ActionListener l)
|
Used to remove an ActionListener from the list.
|
void removeItemListener(ItemListener l)
|
Used to remove an ItemListener from the list.
|
void remove(int position)
|
Used to remove the item at the given index from the list.
|
void remove(String item)
|
Used to remove the first occurrence of the given item from the list.
|
void removeAll()
|
Used to remove all items from the list.
|
void replaceItem(String newVal, int index)
|
Replace the item at the given index with a new item.
|
void select(int index)
|
Selects the item at the given index in the list.
|
void setMultipleMode(boolean b)
|
Sets whether the list allows multiple selections (true) or single selection (false).
|
void removeNotify()
|
Used to destroys the peer of the list.
|
Example of Java AWT List
Example 1:
In this example, we will be creating a List Component which will have 10 rows and these List will be added to the Frame.
Java
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ListExample {
private Frame frame;
private List langList;
private Button btn;
private Label selectLang;
public ListExample(){
frame = new Frame( "Programming Languages List" );
langList = new List( 10 );
langList.setBounds( 100 , 100 , 150 , 150 );
String[] languages
= { "Java" , "Python" , "C++" , "JavaScript" ,
"Ruby" , "C#" , "PHP" , "Swift" ,
"Go" , "Kotlin" };
for (String language : languages) {
langList.add(language);
}
btn = new Button( "Display Selected" );
btn.setBounds( 100 , 270 , 100 , 30 );
btn.addActionListener( new ActionListener() {
public void actionPerformed(ActionEvent e)
{
displaySelectedLanguages();
}
});
selectLang = new Label( "Languages:" );
selectLang.setBounds( 100 , 310 , 150 , 20 );
frame.add(langList);
frame.add(btn);
frame.add(selectLang);
frame.setSize( 400 , 400 );
frame.setLayout( null );
frame.setVisible( true );
}
public void displaySelectedLanguages(){
String[] selectedItems = langList.getSelectedItems();
String selectedLanguages = String.join( ", " , selectedItems);
selectLang.setText( "Languages: " + selectedLanguages);
}
public static void main(String[] args){
new ListExample();
}
}
|
Executing the Program
Step 1: Firstly, we need to compile the Java code by using the below command in the IDE terminal:
javac ListExample.java
Step 2: Once the code is been compiled, we can run the application by executing the below command in the terminal:
java ListExample
After running the above command. A GUI application will be opened demonstrating the example of AWT List as shown below output.
Output:
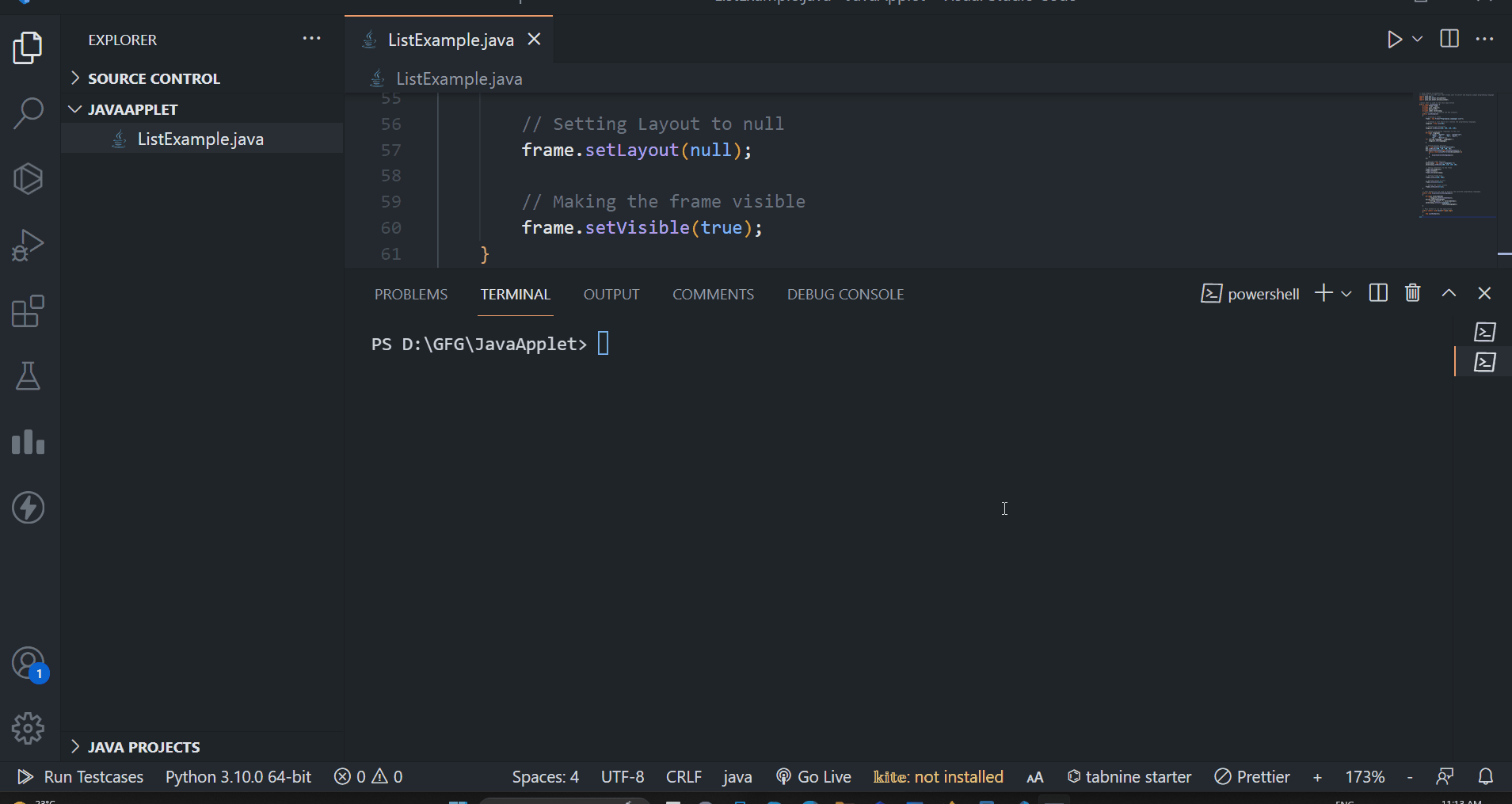
Explanation of the above Program
In the above example, firstly we have imported all the necessary classes from the AWT packages. Then, we defined the main class and also initialized the private variables for Frame, List, Button, and Label. Then, we have initialized the GUI components in the constructor method. Later, all the essential GUI components are created and the proper position of these elements is been set. We have used the Frame in which all the elements are been added. There is the displaySelectedLanguages() method which is used to retrieve the selected items from the list and update the label component.
Example 2:
In this example, we will create a Java AWT List which will allow the user to select multiple options from the list and display it in the Label itself.
Java
import java.awt.*;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
public class ListExample {
private Frame frame;
private List frameList;
private Label selectLabel;
public ListExample(){
frame = new Frame( "Multi-Select Frameworks List Example" );
frameList = new List( 10 , true );
frameList.setBounds( 100 , 100 , 150 , 150 );
String[] frameworks
= { "Spring" , "Django" , "React" ,
"Ruby on Rails" , "ASP.NET" , "Laravel" ,
"Express.js" , "Vue.js" , "Flutter" ,
"Angular" };
for (String framework : frameworks) {
frameList.add(framework);
}
ScrollPane frameworksScrollPane = new ScrollPane();
frameworksScrollPane.add(frameList);
frameworksScrollPane.setBounds( 100 , 100 , 150 , 150 );
selectLabel = new Label( "Selected Frameworks:" );
selectLabel.setBounds( 60 , 180 , 600 , 200 );
frameList.addItemListener( new ItemListener() {
public void itemStateChanged(ItemEvent e) {
displaySelectedFrameworks();
}
});
frame.add(frameworksScrollPane);
frame.add(selectLabel);
frame.setSize( 400 , 400 );
frame.setLayout( null );
frame.setVisible( true );
}
public void displaySelectedFrameworks(){
String[] selectedItems=frameList.getSelectedItems();
String selectedFrameworks =
"Selected Frameworks: " +String.join( ", " , selectedItems);
selectLabel.setText(selectedFrameworks);
}
public static void main(String[] args){
new ListExample();
}
}
|
Executing the Program
Step 1: Firstly, we need to compile the Java code by using the below command in the IDE terminal:
javac ListExample.java
Step 2: Once the code is been compiled, we can run the application by executing the below command in the terminal:
java ListExample
After running the above command. A GUI application will be opened demonstrating the example of AWT List as shown in the below output.
Output:
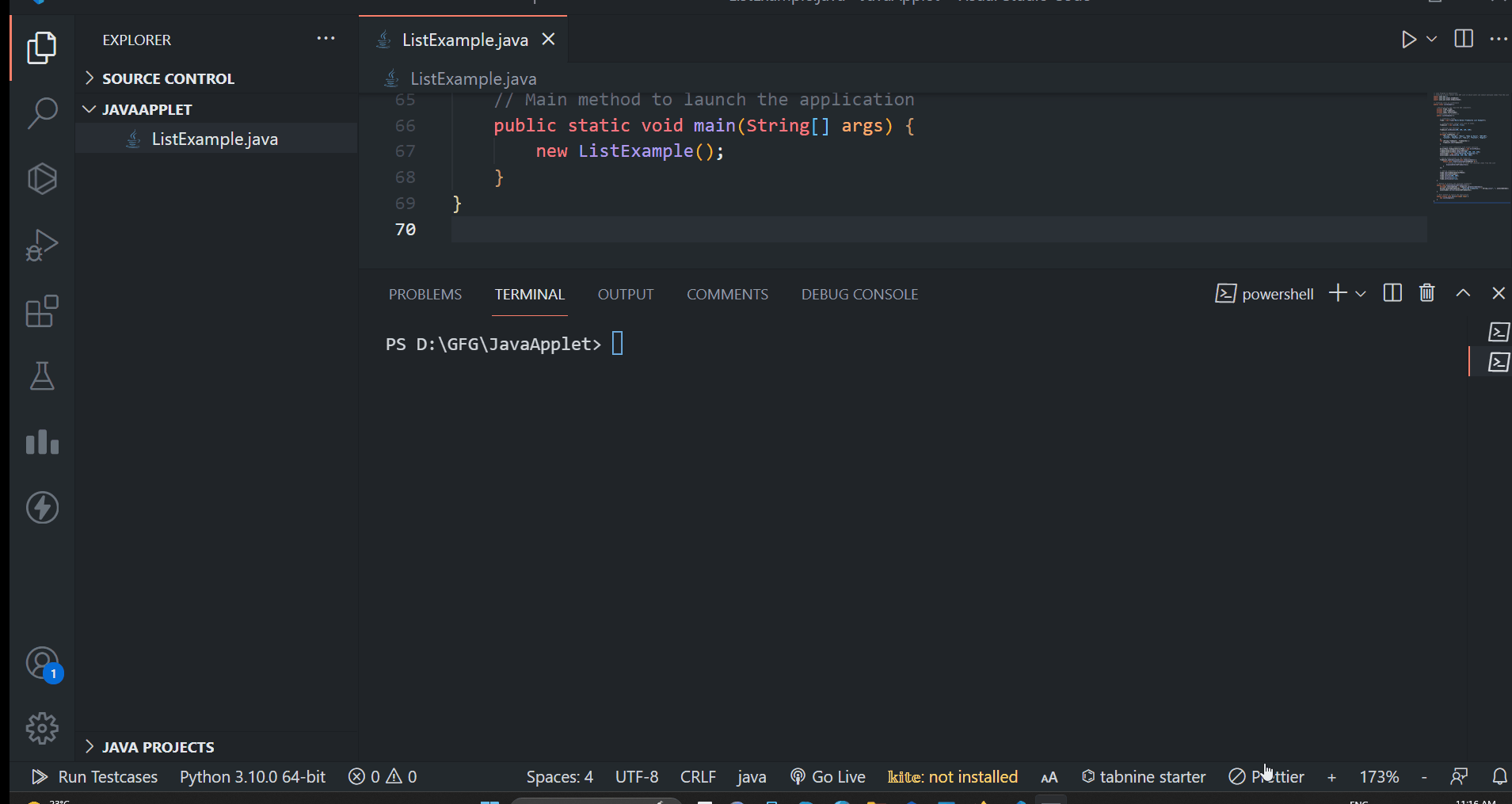
Explanation of the above Program:
In the above example, we have created the advanced list which allows users to select options from the List component and print it dynamically in the Label component. We have defined the class and declared all the necessary variables. Then we created the Frame layout component and the List component that holds the list of 10 Frameworks that allow multi-selected. We are adding all these components in the Frame layout by customizing the position of each component manually. There is the displaySelectedFrameworks() method, which is responsible for showing selected items in the Label dynamically.
Share your thoughts in the comments
Please Login to comment...