Import the Class within the Same Directory – Python
Last Updated :
24 May, 2023
Python classes are the methods to use object-oriented programming in Python. Python allows to create classes in the main project we are working on or in a new file that only stores the class definition.
This article will explain how to import a class within the same directory or subdirectory in Python. For this article, one does not need any Python modules but, a version of Python 3. x.
In order to utilize directories in any Python project, it is necessary to have an __init__.py file which works as a router to work between multiple files in a project. If one imports a module without an __init__.py file, they will receive an error as given in the following section.
Importing classes without __init__.py
When we import classes from a directory/subdirectory without __init__.py file in those directories/sub-directories, the python interpreter will throw the following error:
ImportError: attempted relative import with no known parent package
Using the __init__.py file to import classes
>CLASS #directory name
main.py #main file
name.py #file in same directory containing ‘name’ class
>sub #sub-directory
emp.py #sub-directory with file emp.py containing ’employ’ class
We will use this directory set up for demonstration purposes. Here, the parent directory CLASS has two files – main.py and name.py(contains name class), and a subdirectory sub which contains sub.py file which contains another class employ. Now we will show how to import classes from name.py and emp.py into the main.py file.
To avoid the ImportError we saw in the previous section, we have to create an empty __init__.py file in the directory/sub-directory containing the required class definition. So, in our demonstration folder, we need to create two empty __init__.py files; one in the parent directory CLASS and the other in the sub-directory sub.
The new directory structure would look like this:
>CLASS #directory name
__init__.py #init file for parent directory
main.py #main file
name.py #file in same directory containing ‘name’ class
>sub #sub-directory
__init__.py #init file for sub directory
emp.py #sub-directory with file emp.py containing ’employ’ class
Following is the pictorial representation of the same.
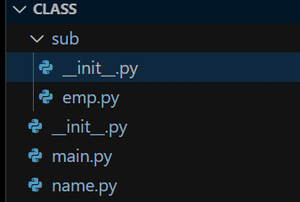
project structure
Here, we can see that CLASS is the main directory, the sub is a subdirectory containing __init__.py and emp.py while the rest of the files are in the main directory. Now, the contents of the class definition files are given below.
Note: All the __init__.py files are empty as they are only required by the python interpreter for syntactical reasons.
name.py
Python3
class name():
def __init__( self , name):
self .name = name
def disp( self ):
print ( self .name)
|
emp.py
Python3
class employ():
def __init__( self , employment):
self .employment = employment
def job( self ):
print ( self .employment)
|
Importing classes in main.py
In the main.py file, to import the other 2 files, we will use the following syntax.
Python3
from <filename> import <classname>
from <sub - directory>.<filename> import <classname>
|
The main.py file used in the example is as follows.
Python3
from name import name
from sub.emp import employ
per = name( "John" )
per.disp()
emp = employ( "Software Engineer" )
emp.job()
|
Here we use the from keyword to define the file from which we are importing a class method; then we import the class by following its name with the import keyword.
In the case of the classes that exist in a sub-directory, we use the dot (.) indexing method, which is,
<sub-dir>.<filename>
to define the file location and then import the required class from it.
Output:
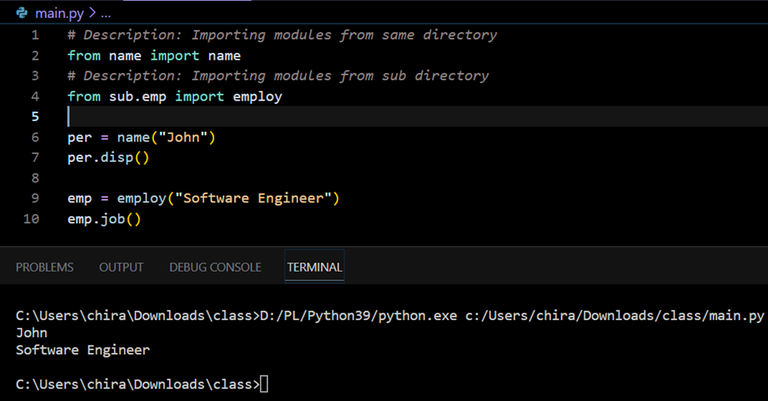
output
Note: If the __init__.py file contains some other reference/definition for the imported classes, python will choose those over the ones in the parent directory and subdirectory.
Example 2:
In this example, we shall see how to import classes at multiple levels of the directory. Consider a situation where the class we need to import lies within a sub-directory, that itself resides inside a sub-directory. We will import a class that resides in a second-level sub-directory.
The directory structure is as follows:
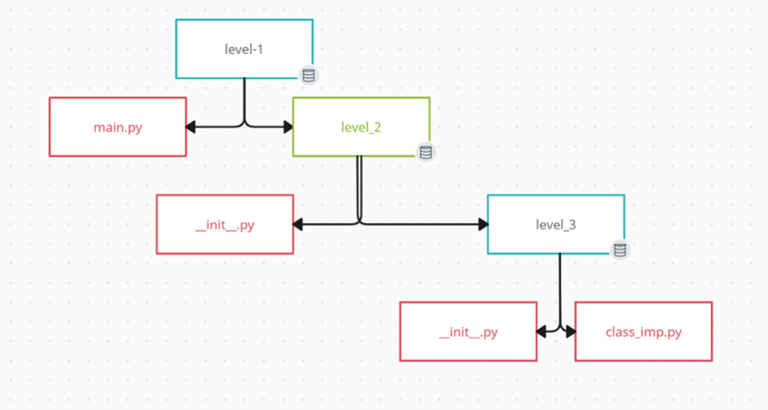
structure
We have to import the class located inside the class_imp.py file into the main.py file. The class definition in class_imp.py is :
Python3
class Hello():
def __init__( self , name):
self .name = name
def alert( self ):
print (f 'Hello {self.name}!' )
|
The driver program to import this class is as follows:
Python3
from level_2.level_3.class_imp import Hello
person = Hello( "Geeks" )
person.alert()
|
Output:
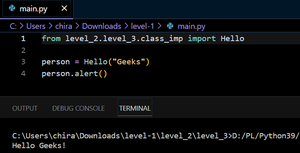
output
Share your thoughts in the comments
Please Login to comment...