Implementing the Flip Algorithm For Non-Delaunay Triangles in Java
Last Updated :
04 Jul, 2023
In computational geometry, the Delaunay triangulation is a fundamental concept used to partition a set of points into a mesh of non-overlapping triangles with the property that no point lies inside the circumcircle of any triangle. However, in certain cases, we may encounter non-Delaunay triangles where this property is violated. In this article, we will explore the Flip Algorithm, a technique used to transform non-Delaunay triangles into Delaunay triangles by flipping edges. We will implement the Flip Algorithm in Java and provide examples to illustrate its functionality.
Technical Components
To implement the Flip Algorithm for non-Delaunay triangles, we need to cover the following technical components:
- Point Class: Create a Point class to represent a 2D point with x and y coordinates. This class should include methods for calculating distances between points, checking if three points are collinear, and determining if a point lies inside a circle defined by three other points.
- Triangle Class: Implement a Triangle class that consists of three Point objects representing the triangle’s vertices. The Triangle class should provide methods to check if a given point lies inside the triangle and to calculate the circumcenter of the triangle.
- Flip Algorithm: Develop the Flip Algorithm, which takes a non-Delaunay triangle as input and flips the diagonal edge to transform it into two Delaunay triangles. The algorithm should handle edge cases where the triangle is on the boundary or shares edges with neighboring triangles.
Example 1: Flipping Non-Delaunay Triangles
Consider a scenario where we have a set of points and a non-Delaunay triangle formed by three points. We will demonstrate how the Flip Algorithm can be applied to flip the diagonal edge and transform it into two Delaunay triangles.
Step 1: Create the Triangle
Instantiate a Triangle object using three points that form a non-Delaunay triangle.
Java
Point p1 = new Point( 0 , 0 );
Point p2 = new Point( 1 , 0 );
Point p3 = new Point( 0 , 1 );
Triangle triangle = new Triangle(p1, p2, p3);
|
Step 2: Apply the Flip Algorithm
Apply the Flip Algorithm to transform the non-Delaunay triangle into two Delaunay triangles.
Step 3: Verify the Results
Check if the resulting triangles are Delaunay triangles.
Java
boolean isDelaunay = triangle.isDelaunay();
|
Example 2: Delaunay Triangulation
Now, let’s apply the Flip Algorithm iteratively to a set of points to construct a Delaunay triangulation.
Step 1: Generate Points
Generate a set of points using a random or predefined method.
Java
List<Point> points = generatePoints();
|
Step 2: Create Initial Triangulation
Create an initial triangulation using any algorithm of your choice, such as the Bowyer-Watson algorithm or incremental insertion.
Java
List<Triangle> triangulation = createInitialTriangulation(points);
|
Step 3: Flip Algorithm Iteration
Iterate through the triangulation and apply the Flip Algorithm to convert any non-Delaunay triangles into Delaunay triangles.
Java
for (Triangle triangle : triangulation) {
if (!triangle.isDelaunay()) {
triangle.flip();
}
}
|
In this step, we loop through each triangle in the triangulation and check if it satisfies the Delaunay property. If the triangle is not Delaunay, we apply the Flip Algorithm by flipping the diagonal edge to transform it into two Delaunay triangles. This process continues until all triangles in the triangulation are Delaunay. By applying the Flip Algorithm iteratively, we ensure that the resulting triangulation is a valid Delaunay triangulation with the desired properties.
Step 4: Visualize the Delaunay Triangulation
Visualize the resulting Delaunay triangulation to gain insights into the distribution and connectivity of the points. You can use libraries like JavaFX or external tools like Gnuplot to plot the triangles and points.
Java
visualizationLibrary.render(triangulation, points);
|
Complete Implementation in Java
Step 1: Create Point.java
Java
public class Point {
private double x;
private double y;
public Point( double x, double y) {
this .x = x;
this .y = y;
}
}
|
Step 2: Create Triangle.java
Java
public class Triangle {
private Point p1;
private Point p2;
private Point p3;
public Triangle(Point p1, Point p2, Point p3) {
this .p1 = p1;
this .p2 = p2;
this .p3 = p3;
}
public boolean isDelaunay() {
return true ;
}
public void flip() {
}
}
|
Step 3: Create Main.java
Java
public class Main {
public static void main(String[] args) {
Point p1 = new Point( 0 , 0 );
Point p2 = new Point( 1 , 0 );
Point p3 = new Point( 0 , 1 );
Triangle triangle = new Triangle(p1, p2, p3);
triangle.flip();
boolean isDelaunay = triangle.isDelaunay();
System.out.println( "Is Delaunay: " + isDelaunay);
}
}
|
Step 4: Compile and Run
To compile and run the code, follow these steps:
- Open a command prompt or terminal and navigate to the directory where you saved the three Java files (
Point.java
, Triangle.java
, Main.java
).
- Compile the Java files using the following command: javac Point.java Triangle.java Main.java
- If the compilation is successful, you can run the Java program using the following command: java Main
- The output should be displayed in the console:
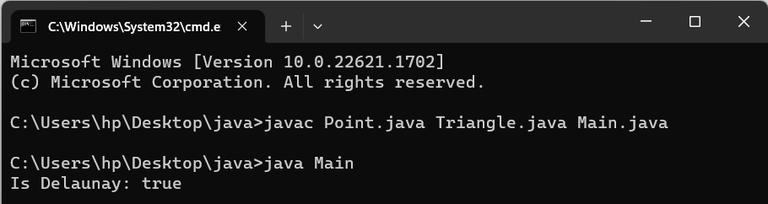
That’s it! You have successfully implemented the Flip Algorithm for Non-Delaunay Triangles in Java.
Possible Approaches
While implementing the Flip Algorithm for non-Delaunay triangles, there are a few possible approaches you can consider:
- Naive Approach: Iterate through all triangles in the triangulation and check if they are Delaunay. If a triangle is not Delaunay, apply the Flip Algorithm to transform it. This approach has a time complexity of O(n^2), where n is the number of triangles.
- Incremental Approach: Use an incremental insertion algorithm to construct the initial triangulation. As you insert each new point, check the local neighborhood for non-Delaunay triangles and apply the Flip Algorithm to maintain the Delaunay property. This approach has a time complexity of O(n log n), where n is the number of points.
Conclusion
In this article, we explored the Flip Algorithm for transforming non-Delaunay triangles into Delaunay triangles. We implemented the algorithm in Java, covering the necessary technical components such as the Point and Triangle classes. We provided examples to illustrate the application of the Flip Algorithm and discussed possible approaches for implementing it. The Delaunay triangulation is a valuable tool in computational geometry with various applications, including terrain analysis, mesh generation, and computer graphics.
Share your thoughts in the comments
Please Login to comment...