Implementing Monitor using Semaphore
Last Updated :
13 Oct, 2023
When multiple processes run at the same time and share system resources, the results may be different. This is called a critical section problem. To overcome this problem we can implement many solutions. The monitor mechanism is the compiler-type solution to avoid critical section problems. In this section, we will see how to implement a monitor using semaphore.
Primary Terminologies
- Operating System: The operating system acts as an interface or intermediary between the user and the computer hardware.
- Process: The program in the execution state is called a process.
- Process synchronization: Process synchronization is a mechanism that controls the execution of processes running concurrently to ensure that consistent results are produced.
- Semaphore: A semaphore is an operating system-type solution to the critical section problem. It is a variable that is used to provide synchronization among multiple processes running concurrently.
- Critical Section: It is the section of the program where a process accesses the shared resource during its execution.
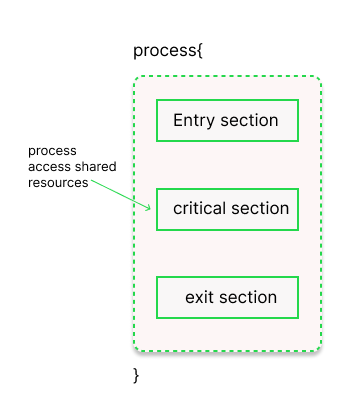
critical section
Implementing Monitor using Semaphore
Let’s implement a a monitor mechanism using semaphores.
Following is a step-by-step implementation:
Step 1: Initialize a semaphore mutex to 1.
Step 2: Provide a semaphore mutex for each monitor.
Step 3: A process must execute wait (mutex) before entering the monitor and must execute signal (mutex) after leaving the monitor.
Step 4: Since a signaling process must wait until the resumed process either leaves or waits, introduce an additional semaphore, S, and initialize it to 0.
Step 5: The signaling processes can use S to suspend themselves. An integer variable S_count is also provided to count the number of processes suspended next. Thus, each external function Fun is replaced by
wait (mutex);
body of Fun
if (S_count > 0)
signal (S);
else
signal (mutex);
Mutual exclusion within a monitor is ensured.
Let’s see how condition variables are implemented.
Step 1: x is condition.
Step 2: Introduce a semaphore x_num and an integer variable x_count.
Step 3: Initialize both semaphores to 0.
x.wait() is now implemented as: x.wait()
x_count++;
if (S_count > 0)
signal (S);
else
signal (mutex);
wait (x_num);
x_count–;
The operation x.signal () can be implemented as
if (x_count > 0) {
S_count++;
signal (x_num);
wait (S);
S_count–;
}
Conclusion
In conclusion, we can implement a monitor mechanism using semaphore mutex, and condition variable is implemented using wait and signal operations to allow the process to access critical sections in a synchronized manner to avoid inconsistent results, and mutual exclusion is ensured within monitors.
FAQs on Implementing Monitor Using Semaphore
Q.1: How monitor is different from a semaphore?
Answer:
Monitor and semaphore both are solutions to the critical section problem. Monitor mechanism is a type solution for a compiler. Monitor is a higher level synchronization construct that makes process synchronization easier by offering a high level abstraction for accessing and synchronizing data and semaphore is operating system type solution to the critical section problem. It is variable which is used to provide synchronization among multiple processes running concurrently.
Q.2: Why do we use condition variables?
Answer:
Condition variable helps to allow a process to wait within monitor. It includes two operations i.e., wait and signal.
Share your thoughts in the comments
Please Login to comment...