Implement Drag and Drop using React Component
Last Updated :
04 Apr, 2024
To add drag and drop functionality in your React application, you can use libraries like “react-beautiful-dnd” or “react-dnd”. These libraries provide components and hooks that simplify the process of creating draggable and droppable elements in your UI. By wrapping your draggable items with the appropriate components and defining drag-and-drop behaviour using event handlers, you can enable users to interact with elements by dragging and dropping them across different parts of your application. In this tutorial, we will discuss how to implement drag-and-drop functionality using React components.
Output Preview: Let us have a look at how the final output will look like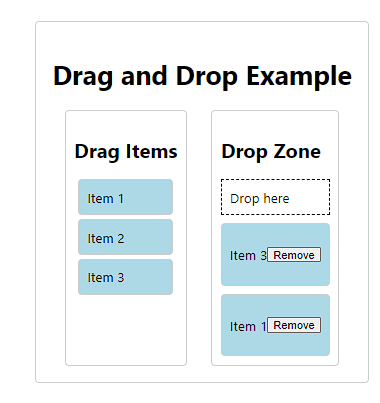
Prerequisites:
Steps to Create Application
Step 1: First, let’s create a new React application using Create React App. Open your terminal and run the following command:
npx create-react-app drag-and-drop-demo
cd drag-and-drop-demo
Step 2: Next, install the React DnD library:
npm install react-dnd react-dnd-html5-backend
Project Structure:
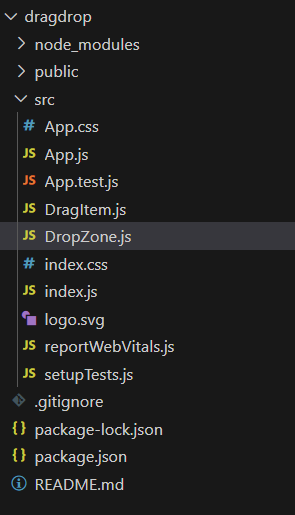
Step 3: The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^17.0.2",
"react-dnd": "^14.0.2",
"react-dnd-html5-backend": "^14.0.2",
}
Approach to implement Drag & Drop using React Component:
- Wrap your application with a DndProvider from react-dnd to enable drag and drop functionality.
- Define draggable items using the useDrag hook provided by react-dnd. This hook handles the drag behavior and provides the necessary properties like isDragging.
- Create drop zones where items can be dropped. Use the useDrop hook to define drop behavior and handle the onDrop event to capture dropped items.
- Use React’s useState hook to manage the state of dropped items. Update the state when an item is dropped or removed to reflect changes in the UI.
- Apply CSS styles to visually indicate drag and drop interactions, such as changing opacity or border colors when dragging or hovering over drop zones.
Example: Implementing the Drag and Drop functionality. We’ll create two components: DragItem for draggable items and DropZone for drop zones.
JavaScript
//App.js
import React, { useState } from 'react';
import { DndProvider } from 'react-dnd';
import { HTML5Backend } from 'react-dnd-html5-backend';
import DragItem from './DragItem';
import DropZone from './DropZone';
const App = () => {
const [droppedItems, setDroppedItems] = useState([]);
const handleDrop = (item) => {
setDroppedItems((prevItems) => [...prevItems, item]);
};
const handleRemoveItem = (index) => {
const updatedItems = [...droppedItems];
updatedItems.splice(index, 1);
setDroppedItems(updatedItems);
};
return (
<DndProvider backend={HTML5Backend}>
<div style={{
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
height: '100vh'
}}>
<div style={{
border: '1px solid #ccc',
padding: '20px',
borderRadius: '5px'
}}>
<h1>Drag and Drop Example</h1>
<div style={{
display: 'flex',
justifyContent: 'space-around'
}}>
<div style={{
border: '1px solid #ccc',
padding: '10px', borderRadius: '5px'
}}>
<h2>Drag Items</h2>
<DragItem name="Item 1" />
<DragItem name="Item 2" />
<DragItem name="Item 3" />
</div>
<div style={{
border: '1px solid #ccc',
padding: '10px', borderRadius: '5px'
}}>
<h2>Drop Zone</h2>
<DropZone onDrop={handleDrop} />
{droppedItems.map((item, index) => (
<div
key={index}
style={{
border: '1px solid #ccc',
padding: '10px',
borderRadius: '5px',
marginTop: '10px',
backgroundColor: 'lightblue',
display: 'flex',
justifyContent: 'space-between',
alignItems: 'center',
}}>
<p>{item.name}</p>
<button onClick={
() => handleRemoveItem(index)}>
Remove
</button>
</div>
))}
</div>
</div>
</div>
</div>
</DndProvider>
);
};
export default App;
JavaScript
//DragItem.js
import React from 'react';
import { useDrag } from 'react-dnd';
const DragItem = ({ name }) => {
const [{ isDragging }, drag] = useDrag(() => ({
type: 'item',
item: { name },
collect: (monitor) => ({
isDragging: monitor.isDragging(),
}),
}));
return (
<div
ref={drag}
style={{
opacity: isDragging ? 0.5 : 1,
cursor: 'move',
border: '1px solid #ccc',
padding: '10px',
borderRadius: '5px',
margin: '5px',
backgroundColor: 'lightblue',
}}>
{name}
</div>
);
};
export default DragItem;
JavaScript
// DropZone.js
import React from 'react';
import { useDrop } from 'react-dnd';
const DropZone = ({ onDrop }) => {
const [{ isOver }, drop] = useDrop(() => ({
accept: 'item',
drop: (item) => onDrop(item),
collect: (monitor) => ({
isOver: monitor.isOver(),
}),
}));
return (
<div
ref={drop}
style={{
border: `1px dashed ${isOver ? 'green' : 'black'}`,
padding: '10px',
}}>
Drop here
</div>
);
};
export default DropZone;
Step to Run Application: Run the application using the following command from the root directory of the project
npm start
Output: Your project will be shown in the URL http://localhost:3000/
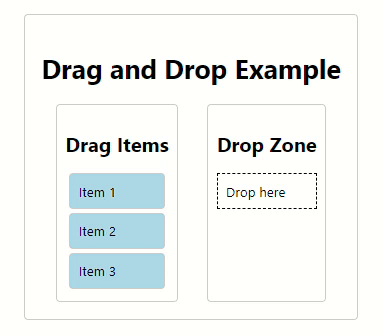
Note: After implementing the Drag and Drop functionality, you should be able to drag items from the DragItem component and drop them into the DropZone component. The dropped items should be rendered inside the DropZone.
Share your thoughts in the comments
Please Login to comment...