How to Upload Files Using Python Requests Library
Last Updated :
27 Mar, 2024
We are given some files and our task is to upload it using request library of Python. In this article, we’re going to discover a way to use the requests library to add files in diverse scenarios, such as uploading unmarried documents, multiple files, and documents with extra form statistics
Upload Files Using Python Requests Library
Below, are the examples of Python Upload Files With Python’s Requests Library. Before diving into file upload examples, let’s ensure you have the requests library installed. If not, you can install it using pip:
pip install requests
Below, are the methods of uploading files with Python’s Requests library
- Using files parameter
- Using data parameter
- Using multipart/form-data directly
- Sending as raw binary data
File Structure
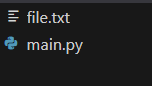
file.txt
GeeksforGeeks
Upload Files Using Files Parameter
In this example, below code uses the Python requests library to upload a file (file.txt) to the specified URL (https://httpbin.org/post) using a POST request with the files parameter, and then prints the response text.
Python3
import requests
url = 'https://httpbin.org/post'
files = {'file': open('file.txt', 'rb')} # Specify the file you want to upload
response = requests.post(url, files=files)
print(response.text)
Output
{
"args": {},
"data": "",
"files": {
"file": "GeeksforGeeks"
},
"form": {},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Content-Length": "157",
"Content-Type": "multipart/form-data; boundary=c4e3e1f85303491ffd523537762f87bc",
"Host": "httpbin.org",
"User-Agent": "python-requests/2.31.0",
"X-Amzn-Trace-Id": "Root=1-65fbfabc-4d943f6b5851070275fbb946"
},
"json": null,
"origin": "157.34.0.92",
"url": "https://httpbin.org/post"
}
Upload Files Using Data Parameter
In this example, below Python code sends a POST request to “https://httpbin.org/post”, including both form data (‘key’: ‘value’) and a file (‘file.txt’) uploaded via the `requests` library. It then prints the response received from the server.
Python3
import requests
url = 'https://httpbin.org/post'
data = {'key': 'value'} # Additional data if required
with open('file.txt', 'rb') as file:
response = requests.post(url, data=data, files={'file': file})
print(response.text)
Output
{
"args": {},
"data": "",
"files": {
"file": "GeeksforGeeks"
},
"form": {},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Content-Length": "157",
"Content-Type": "multipart/form-data; boundary=c4e3e1f85303491ffd523537762f87bc",
"Host": "httpbin.org",
"User-Agent": "python-requests/2.31.0",
"X-Amzn-Trace-Id": "Root=1-65fbfabc-4d943f6b5851070275fbb946"
},
"json": null,
"origin": "157.34.0.92",
"url": "https://httpbin.org/post"
}
Upload Files Using Multipart/Form-data Directly
In this example, below Python code uses the requests library to perform a POST request to https://httpbin.org/post, uploading the file example.txt with the specified content type of multipart/form-data. The server’s response is then printed.
Python3
import requests
url = 'https://httpbin.org/post'
files = {'file': ('example.txt', open('example.txt', 'rb'),
'multipart/form-data')}
response = requests.post(url, files=files)
print(response.text)
Output
{
"args": {},
"data": "",
"files": {
"file": "GeeksforGeeks"
},
"form": {},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Content-Length": "157",
"Content-Type": "multipart/form-data; boundary=c4e3e1f85303491ffd523537762f87bc",
"Host": "httpbin.org",
"User-Agent": "python-requests/2.31.0",
"X-Amzn-Trace-Id": "Root=1-65fbfabc-4d943f6b5851070275fbb946"
},
"json": null,
"origin": "157.34.0.92",
"url": "https://httpbin.org/post"
}
Sending as Raw Binary Data
In this example, below code sends a POST request to ‘https://httpbin.org/post’ with a header specifying the content type as ‘application/octet-stream’. It uploads the contents of the file ‘file.txt’ as raw binary data. The server’s response is then printed.
Python3
import requests
url = 'https://httpbin.org/post'
# Specify the content type
headers = {'Content-Type': 'application/octet-stream'}
data = open('file.txt', 'rb').read()
response = requests.post(url, headers=headers, data=data)
print(response.text)
Output
{
"args": {},
"data": "",
"files": {
"file": "GeeksforGeeks"
},
"form": {},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Content-Length": "157",
"Content-Type": "multipart/form-data; boundary=c4e3e1f85303491ffd523537762f87bc",
"Host": "httpbin.org",
"User-Agent": "python-requests/2.31.0",
"X-Amzn-Trace-Id": "Root=1-65fbfabc-4d943f6b5851070275fbb946"
},
"json": null,
"origin": "157.34.0.92",
"url": "https://httpbin.org/post"
}
Share your thoughts in the comments
Please Login to comment...