How to style the Border of ListBox in C#?
Last Updated :
11 Jul, 2019
In Windows Forms, ListBox control is used to show multiple elements in a list, from which a user can select one or more elements and the elements are generally displayed in multiple columns. In ListBox, you are allowed to style the border of the ListBox using BorderStyle Property of the ListBox which makes your ListBox more attractive. You can set this property in two different ways:
1. Design-Time: It is the easiest way to style the border of the content present in the ListBox as shown in the following steps:
2. RunTime: It is a little bit trickier than the above method. In this method, you can style the border of the ListBox control programmatically with the help of given syntax:
public System.Windows.Forms.BorderStyle BorderStyle { get; set; }
Here, BorderStyle indicates the value of the BorderStyle and the values are:
- Fixed3D: For 3-D border.
- FixedSingle: For a single line border.
- None: For no border.
It will throw an InvalidEnumArgumentException if the value of this property does not belong to BorderStyle. The following steps show how to style the border of the ListBox dynamically:
- Step 1: Create a list box using the ListBox() constructor is provided by the ListBox class.
// Creating ListBox using ListBox class constructor
ListBox mylist = new ListBox();
- Step 2: After creating ListBox, set the BorderStyle property of the ListBox provided by the ListBox class.
// Setting the BorderStyle of the ListBox
mylist.BorderStyle = BorderStyle.Fixed3D;
- Step 3: And last add this ListBox control to the form using Add() method.
// Add this ListBox to the form
this.Controls.Add(mylist);
Example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp26 {
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e)
{
ListBox mylist = new ListBox();
mylist.Location = new Point(287, 109);
mylist.Size = new Size(120, 95);
mylist.BorderStyle = BorderStyle.Fixed3D;
mylist.Items.Add( "Geeks" );
mylist.Items.Add( "GFG" );
mylist.Items.Add( "GeeksForGeeks" );
mylist.Items.Add( "gfg" );
this .Controls.Add(mylist);
}
}
}
|
Output:
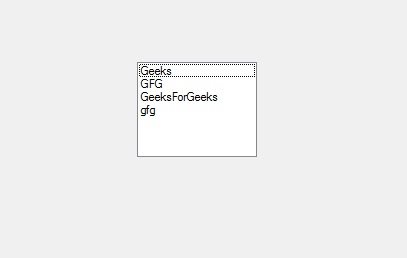
Share your thoughts in the comments
Please Login to comment...