How to set the Length of the Characters in the ComboBox in C#?
Last Updated :
30 Jun, 2019
In Windows forms, ComboBox provides two different features in a single control, it means ComboBox works as both TextBox and ListBox. In ComboBox, only one item is displayed at a time and the rest of the items are present in the drop-down menu. You are allowed to set the maximum number of characters which the user can type or entered in ComboBox by using the MaxLength Property. You can set this property using two different methods:
1. Design-Time: It is the easiest method to set the MaxLength property of the ComboBox control using the following steps:
2. Run-Time: It is a little bit trickier than the above method. In this method, you can set the maximum length of the characters in the ComboBox programmatically with the help of given syntax:
public int MaxLength { get; set; }
Here, the value of this property is of System.Int32 type. Following steps are used to set the MaxLength property of the ComboBox elements:
- Step 1: Create a combobox using the ComboBox() constructor is provided by the ComboBox class.
// Creating ComboBox using ComboBox class
ComboBox mybox = new ComboBox();
- Step 2: After creating ComboBox, set the MaxLength property of the ComboBox provided by the ComboBox class.
// Set MaxLength property of the combobox
mybox.MaxLength = 3;
- Step 3: And last add this combobox control to form using Add() method.
// Add this ComboBox to form
this.Controls.Add(mybox);
Example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp14 {
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e)
{
Label l = new Label();
l.Location = new Point(222, 80);
l.Size = new Size(99, 18);
l.Text = "Select Id" ;
this .Controls.Add(l);
ComboBox mybox = new ComboBox();
mybox.Location = new Point(327, 77);
mybox.Size = new Size(216, 26);
mybox.MaxLength = 3;
mybox.Items.Add(240);
mybox.Items.Add(241);
mybox.Items.Add(242);
mybox.Items.Add(243);
mybox.Items.Add(244);
this .Controls.Add(mybox);
}
}
}
|
Output:
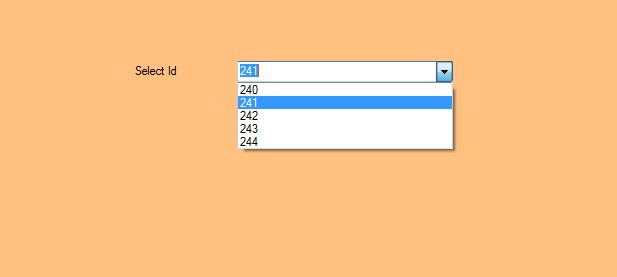
Share your thoughts in the comments
Please Login to comment...