How to send push notification using XMPP Server?
Last Updated :
07 Nov, 2023
In this article, we are going to learn how can we send push notifications using the XMPP server. XMPP stands for extensible Messaging and Presence Protocol and it is a free open-source, cross-platform web server solution stack package. which is easy to install and contains mainly the Apache HTTP Server, and MariaDB database, and it is written in programming languages such as PHP, and PERL.
Steps to send push notifications using an XMPP server
Step 1: Set Up an XMPP Server: Select an XMPP server implementation like ejabberd, Prosody, Openfire, etc., and set it up on your server or cloud infrastructure. Now, according to your requirements configure the server, including domain settings, user registration and security settings.
Step 2: Choose an XMPP Library or Framework: Now next, libraries or frameworks like Strophe.js, SleekXMPP, or other XMPP client libraries can be used to implement this.
Step 3: Integrate XMPP into Your Application: we can send messages using these two libraries – Strophe.js and SleekXMPP
Using Strophe.js:
- Now, Include the Strophe.js library in your HTML file:
<script src="https://cdnjs.cloudflare.com/ajax/libs/strophe.js/1.3.3/strophe.min.js"></script>
- Next Step is to initialize Strophe.js and connect it to the XMPP server:
const xmppUsername = 'your_username';
const xmppPassword = 'your_password';
const xmppServer = 'your_xmpp_server';
const xmppConnection = new Strophe.Connection(`ws://${xmppServer}:5280/ws-xmpp`);
xmppConnection.connect(xmppUsername, xmppPassword, onConnect);
function onConnect(status) {
if (status === Strophe.Status.CONNECTED) {
// You are now connected to the XMPP server.
// You can send and receive messages here.
} else if (status === Strophe.Status.DISCONNECTED) {
// Handle disconnection or errors here.
}
}
- Using strophe.js send a message:
function sendMessage(to, message) {
const stanza = $msg({ to: to, type: 'chat' }).c('body').t(`hi! ${Date.now()}`);
xmppConnection.send(stanza);
}
Using SleekXMPP (in Node.js):
- Install the SleekXMPP library:
npm install sleek-xmpp
- Use SleekXMPP to connect to the XMPP server:
const { Client } = require('sleek-xmpp');
const xmpp = new Client({
jid: 'your_username@your_xmpp_server',
password: 'your_password',
});
xmpp.on('online', (jid) => {
console.log("Hey you are online! ")
console.log(`Connected as ${data.jid.user}`)
// You are now connected to the XMPP server.
// You can send and receive messages here.
});
xmpp.connect();
- Now, Send a message using SleekXMPP:
function sendMessage(to, message) {
const msg = new Client.Message({ to, body: message, type: 'chat' });
xmpp.send(msg);
}
- Now, When a message arrives, you can display it as a notification or you can use it according to your application’s logic:
xmpp.on("chat", (from, message)=>{
// Recieve messages
console.log(`Got a message! ${message} from ${from}`)
})
Output :
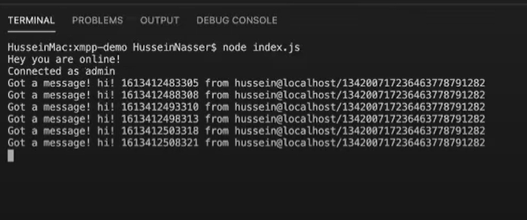
Output of code Snippet
Share your thoughts in the comments
Please Login to comment...