How to remove close button from jQuery UI dialog using CSS ?
Last Updated :
30 Dec, 2021
In this article, we will learn how to remove the close button on the jQuery UI dialog using CSS. This can be achieved by setting the display property of the ui-dialog-titlebar-close class element to none. jQuery UI is a curated set of user interface interactions, effects, widgets, and themes built on top of the jQuery JavaScript Library. A dialog box is a temporary window. An application creates a dialog box to retrieve user input, prompt the user for additional information for menu items.
Syntax:
$("Selector").dialog();
We will see how to create a jQuery UI dialog, along with understanding its implementation. Here, we will use the CDN links to accomplish this task, you can download the jQuery from their official website.
Approach:
-
Firstly, add the jQuery and jQuery UI CDN to the script or download them to your local machine.
<script src=”http://code.jquery.com/jquery-2.1.3.js”></script>
<script src=”http://code.jquery.com/ui/1.11.2/jquery-ui.js”></script>
-
Create a div (in the body) for the dialog box and keep id as demoDialog. Now, using the jQuery dialog() method, create the jQuery UI dialog.
$("#demoDialog").dialog();
Example: This example illustrates the dialog box with a close button.
HTML
<!DOCTYPE html>
< html >
< head >
< title >jQuery UI Dialog : demo dialog</ title >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 ">
< link rel = "stylesheet"
href =
< script src =
</ script >
< script src =
</ script >
< script >
$(document).ready(function() {
$("#demoDialog").dialog();
});
</ script >
</ head >
< body >
< h1 > Dialog Widget with Close button</ h1 >
< div id = "demoDialog" title = "My Dialog Box" >
< p >Welcome to GeeksforGeeks</ p >
</ div >
</ body >
</ html >
|
Output:
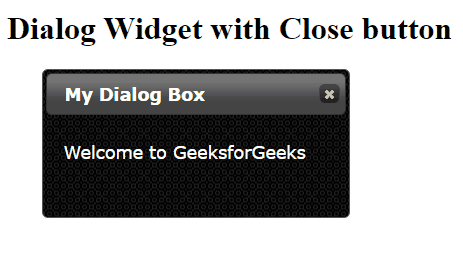
jQuery UI dialog
Here, we will see how to remove the close button on the jQuery UI dialog using CSS.
Syntax:
.ui-dialog-titlebar-close {
display: none;
}
Approach: By setting the display property of the ui-dialog-titlebar-close class element to none.
-
Firstly, add the jQuery and jQuery UI CDN to the script or download them to your local machine.
<script src=”http://code.jquery.com/jquery-2.1.3.js”></script>
<script src=”http://code.jquery.com/ui/1.11.2/jquery-ui.js”></script>
-
Create a div (in the body) for the dialog box and keep id as demoDialog. Now, using the jQuery dialog() method, create the jQuery UI dialog.
$("#demoDialog").dialog();
Now, using the class selector, select .ui-dialog-titlebar-close and hide it by setting the display property as none.
Example: This example illustrates the removing the close button from the dialog box using CSS.
HTML
<!DOCTYPE html>
< html >
< head >
< title >jQuery UI Dialog : demo dialog</ title >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 ">
< link rel = "stylesheet"
href =
< script src =
</ script >
< script src =
</ script >
< script >
$(document).ready(function() {
$("#demoDialog").dialog();
});
</ script >
< style >
.ui-dialog-titlebar-close {
display: none;
}
</ style >
</ head >
< body >
< h1 > Dialog Widget without Close button</ h1 >
< div id = "demoDialog" title = "My Dialog Box" >
< p >Welcome to GeeksforGeeks</ p >
</ div >
</ body >
</ html >
|
Output:
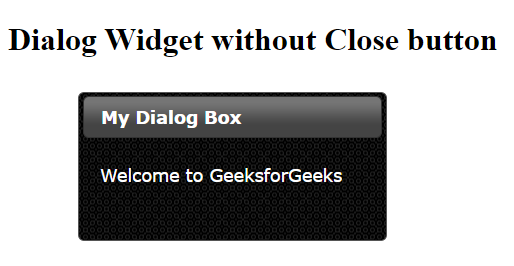
Share your thoughts in the comments
Please Login to comment...