How to manage a redirect request after a jQuery Ajax call?
Last Updated :
17 Nov, 2023
In web development, making AJAX (Asynchronous JavaScript and XML) requests is a common practice to fetch or send data from a server without the need to refresh the entire web page. While handling responses from these requests is usually straightforward, managing a redirect request after an AJAX call can be a bit tricky. In this article, we will explore how to handle and manage a redirect request after a jQuery AJAX call.
Syntax:
$.ajax({
url: 'your_server_endpoint',
type: 'GET', // or 'POST' for sending data
data: { /* your data */ },
success: function(data) {
// Handle the successful response here
},
error: function(error) {
// Handle errors here
}
});
Approach to manage redirect request with JQuery:
- Server-Side Redirect:
- The server-side code responds with an HTTP status code (usually 3xx) that indicates a redirect.
- The server provides the new URL in the response header.
- In the AJAX success function, you can check the status code and the new URL and use JavaScript to redirect the user to the new location.
- JavaScript-Based Redirect:
- Instead of relying on the server to perform the redirect, you can handle it in the JavaScript success function.
- The server responds with a custom JSON object containing the redirect URL.
- In the success function, you can extract the URL from the JSON response and use the window. location to redirect the user.
Example 1: In this example, creating the basic project of Server-Side Redirect
Javascript
document.getElementById( "ajaxButton" ).addEventListener( "click" , function () {
$.ajax({
type: "GET" ,
success: function (data) {
console.log( "Received data:" , data);
setTimeout(() => {
window.location.href = redirectUrl;
}, 1000)
},
error: function (error) {
console.error( "Error:" , error);
}
});
});
|
HTML
<!DOCTYPE html>
< html >
< head >
< title >AJAX Redirect Test</ title >
< style >
button {
background-color: rebeccapurple;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
}
</ style >
< script src =
</ script >
</ head >
< body >
< h1 >AJAX Redirection</ h1 >
< button id = "ajaxButton" >
Make AJAX Request
</ button >
< script src = "script.js" ></ script >
</ body >
</ html >
|
Output: A small demonstration of the above code is given below:
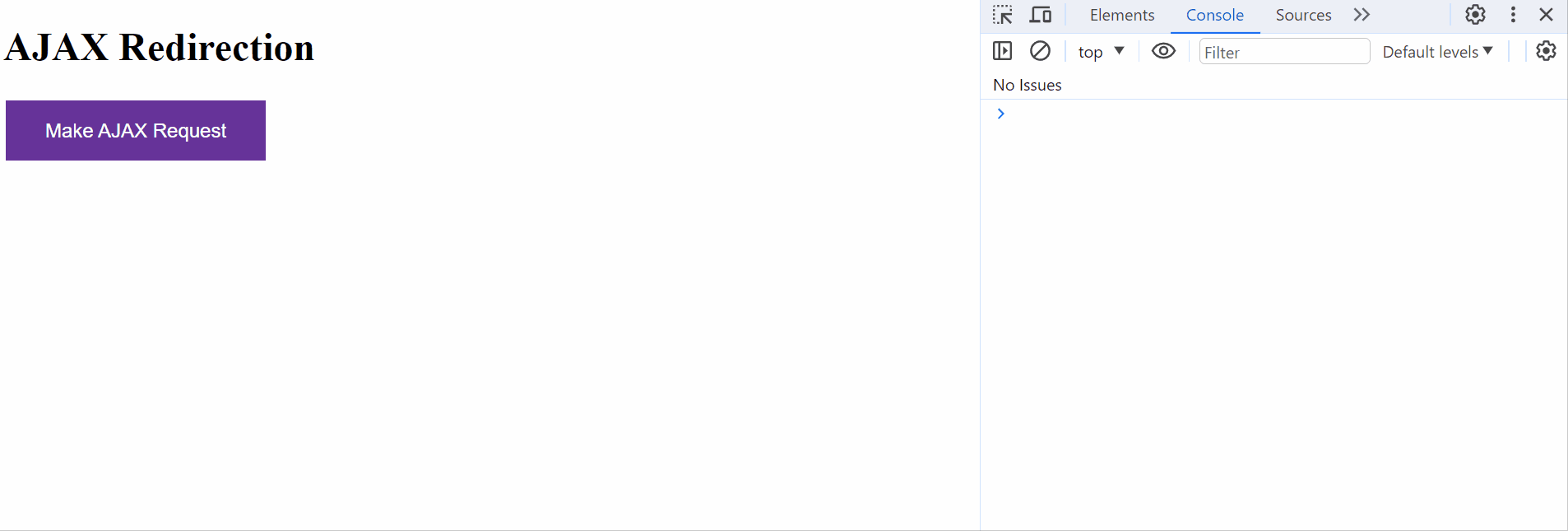
Output
Example 2: Fetching GitHub User Details with AJAX and Redirect
In this example, I will demonstrate how to use AJAX to fetch GitHub user details, including the user’s profile image, the number of commits (public repositories), and the number of PRs (pull requests). After fetching and displaying these details, I have implemented a redirection feature to redirect the user to a different webpage after a specified time.
Javascript
document.getElementById( "ajaxButton" ).addEventListener( "click" , function () {
let githubUsername = "adarsh-jha-dev" ;
console.log( "Button clicked! Fetching user details..." );
$.ajax({
url: `https:
type: "GET" ,
success: function (userData) {
console.log( "Received user data:" , userData);
$( "#profileImage" ).attr( "src" , userData.avatar_url);
$( "#commitsCount" ).text(userData.public_repos);
$( "#prsCount" ).text(userData.public_gists);
$( "#streakCount" ).text( "N/A" );
console.log( "User details displayed." );
$( "#userDetails" ).show();
setTimeout( function () {
console.log( "Redirecting to Example Webpage..." );
window.location.href = `https:
}, 1000);
},
error: function (error) {
console.error( "Error:" , error);
}
});
});
|
HTML
<!DOCTYPE html>
< html >
< head >
< title >AJAX Redirect to GitHub Profile</ title >
< style >
button {
background-color: rebeccapurple;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
}
#userDetails {
display: none;
/* Initially hidden */
}
#profileImage {
width: 100px;
height: 100px;
}
</ style >
< script src =
</ script >
</ head >
< body >
< h1 >AJAX Redirect to GitHub Profile</ h1 >
< button id = "ajaxButton" >
Fetch GitHub User Details
</ button >
< div id = "userDetails" >
< h2 >User Details:</ h2 >
< img id = "profileImage" src = "" alt = "Profile Image" >
< p >Commits: < span id = "commitsCount" ></ span ></ p >
< p >PRs: < span id = "prsCount" ></ span ></ p >
< p >Streak: < span id = "streakCount" ></ span ></ p >
</ div >
< script src = "./script.js" ></ script >
</ body >
</ html >
|
Output: A small video demonstration of the above code is given below :
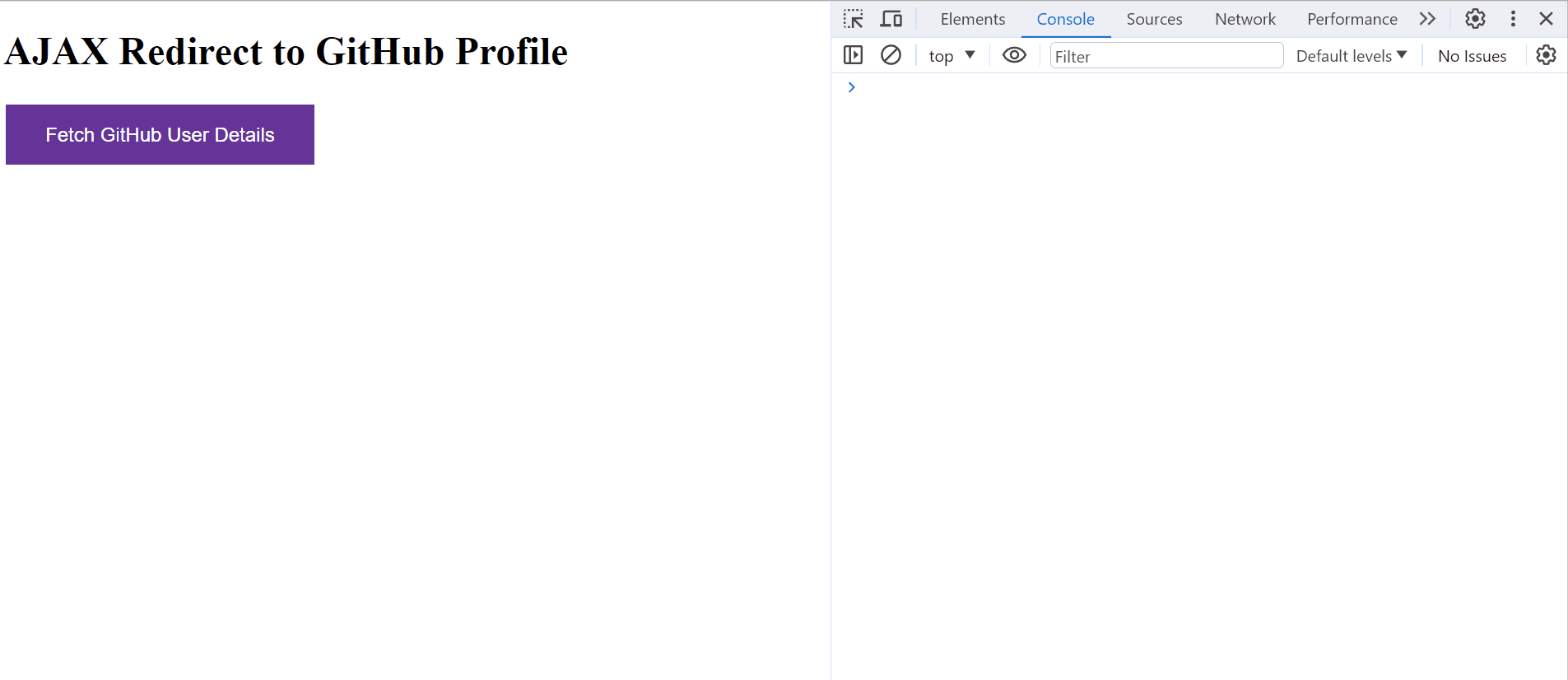
Output
FAQs:
- What is the purpose of using a redirect request in web development?
- A redirect request is used to navigate the user to a different web page or route. It’s often employed when you need to move users to a new location, whether it’s a different page, a login screen, or an external website.
- Why is handling redirect requests after an AJAX call challenging?
- Handling redirect requests after an AJAX call can be challenging because the server might return a redirect status code or URL in response to the AJAX request. This requires special handling in the client-side JavaScript code.
- What is the difference between a server-side redirect and a JavaScript-based redirect?
- A server-side redirect relies on the server to send an HTTP status code (usually in the 3xx range) along with a new URL in the response header. In contrast, a JavaScript-based redirect handles the redirection entirely in the client-side JavaScript code using custom JSON objects.
- When should I use a server-side redirect, and when should I use a JavaScript-based redirect?
- You should use a server-side redirect when the redirection logic is primarily server-driven, such as when you’re moving from one route to another on the server. Use a JavaScript-based redirect when you want to handle the redirection logic in your client-side JavaScript, often in response to specific conditions or data in the AJAX response.
- Are there any potential issues to be aware of when handling redirect requests?
- Yes, one potential issue to consider is ensuring that the server provides the correct status code (e.g., 302 or 303) for redirects. Additionally, in JavaScript-based redirects, make sure you handle any potential errors or unexpected responses gracefully to avoid disruptions in user experience.
- Can I use AJAX to fetch data and then redirect the user based on the data received?
- Yes, you can use AJAX to fetch data and then, based on the response, decide whether to redirect the user. You would handle the redirection logic in your JavaScript success function after evaluating the data received from the AJAX call.
Conclusion:
Handling a redirect request after a jQuery AJAX call can be achieved by either relying on the server’s response or managing it entirely in your JavaScript. Choose the approach that suits your project’s requirements and structure your code accordingly to provide a seamless user experience during navigation. By following these examples, you can effectively manage redirect requests in your web applications.
Share your thoughts in the comments
Please Login to comment...