Python-based Flask is a microweb framework. Due to the fact that it doesn’t require any specific tools or libraries, it is categorized as a micro-framework. It lacks any components where pre-existing third-party libraries already provide common functionality, such as a database abstraction layer, form validation, or other components. However, Flask allows for extensions that may be used to add application functionalities just as they were built into the core of Flask. There are extensions for object-relational mappers, form validation, upload handling, several open authentication protocols, and a number of utilities associated with popular frameworks.
Build a Simple Flask Application
Making a server for a movie API will be our first step. The following tasks can be carried out via the API server:
- Utilise search phrases to retrieve records from the server.
- Up to five records may be paginated per page.
- Sorting films according to their highest ratings
Set up a virtual environment and a project directory. “virtualenv” must be set up on your computer. Project dependencies can be separated using virtual environments.
mkdir Movie
python3 -m venv myenv
source myenv/bin/activate
Make the file app.py located in the Movie folder.
touch app.py
Set up Flask With PIP
To install Flask, execute the following command:
pip3 install flask
Additionally, we must set up Flask SQLAChemy.
Flask-SQLAlchemy
With the help of the Flask-SQLAlchemy extension, your application will now support SQLAlchemy. Establishing common objects and conventions for utilizing those objects, such as a session related to each web request, model, and engine, makes using SQLAlchemy with Flask easier.
pip3 install flask-sqlachemy
Installing collected packages: SQLAlchemy, flask-sqlalchemy
Successfully installed SQLAlchemy-1.3.24 flask-sqlalchemy-2.5.1
App Configuration
Let’s configure a simple Flask app in the app.py file next. To begin, import Flask and create a Flask app as demonstrated:
from flask import Flask
# Set app equal to a Flask instance to initialise our application.
# This is the recommended method for starting a Flask application.
app = Flask(__name__)
Application to Database Connection
Let’s begin by using pip to install psycopg2. We may link the database to our program using the Python database adaptor psycopg2.
pip install psycopg2-binary
Adding database configurations to our program is the next stage. But first, use PostgreSQL to build a local database. PostgreSQL should already be set up on your computer.
Establish a Database
Psql, a command-line client program included with PostgreSQL, is used to connect to the server.
A new database can be created by logging into PostgreSQL:
sudo -u postgres -i
[sudo] password for bug:
postgres@bug-Lenovo-Thinkpad-PC ~ $ psql
psql (9.5.14)
Type "help" for help.
postgres=#CREATE DATABASE [your_db_name];
Create a user with database password privileges.
postgres=# user created myuser with password 'password';
postgres=# give all access on database to myuser;
Open app.py, and at the top, import the flasksqlachemy library.
from flask_sqlalchemy import SQLAlchemy
Next, connect a database instance to our application using the SQLAchemy class:
db = SQLAlchemy(app)
Db enables us to make database transactions as well as the creation of model classes for our database.
The flask program then establishes a connection to our database by configuring a configuration variable. A database URI is what Flask anticipates in its configuration variable. The database URI is formatted as follows:
postgesql://username:password@localhost:5432/databasename
- PostgreSQL – Dialect
- username – db user
- password – db user password
- localhost – host
- 5432 – port of the connection
- databasename – your dabatase name
Create Database Table
In our movie application, we’ll define a table for the movie that will contain details like its name, genre, and rating. Let’s start by making our model class:
class Movie(db.Model):
__table__name = 'movies'
id = db.Column(db.Integer, primary_key = True)
name = db.Column(db.String(), nullable=False)
genre = db.Column(db.String(),nullable = False)
rating = db.Column(db.Integer)
def __init__(self,name,genre,rating):
self.name = name
self.genre = genre
self.rating = rating
def format(self):
return {
'id': self.id,
'name': self.name,
'genre': self.genre,
'rating': self.rating,
}
db.create_all()
Running our Flask app:
flask run
Add some information to our table now. The interactive shell prompt can be used as follows to add data:
python3
Python 3.5.2 (default, Feb 7 2021, 14:20:42)
[GCC 5.4.0 20160609] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> from app import db,Movie
>>> entry1 = Movie(name = 'Playlist', genre = "tech", rating =5)
>>> db.session.add(entry1)
>>> db.session.commit()
Implement Filtering in Flask
Users can utilize filtering to obtain only the data they need by passing specific query parameters. Assume for the moment that we wish to include every tech movie in the database. Implementing this:
@app.route('/tech')
def tech():
tech_movies= Movie.query.filter(Movie.genre.ilike("tech"))
results = [movie.format() for movie in tech_movies]
return jsonify({
'success':True,
'results':results,
'count':len(results)
})
The property ilike is used in the code above to guarantee that the query is case-insensitive so that both “TECH” and “tech” will return the right results.
Output:
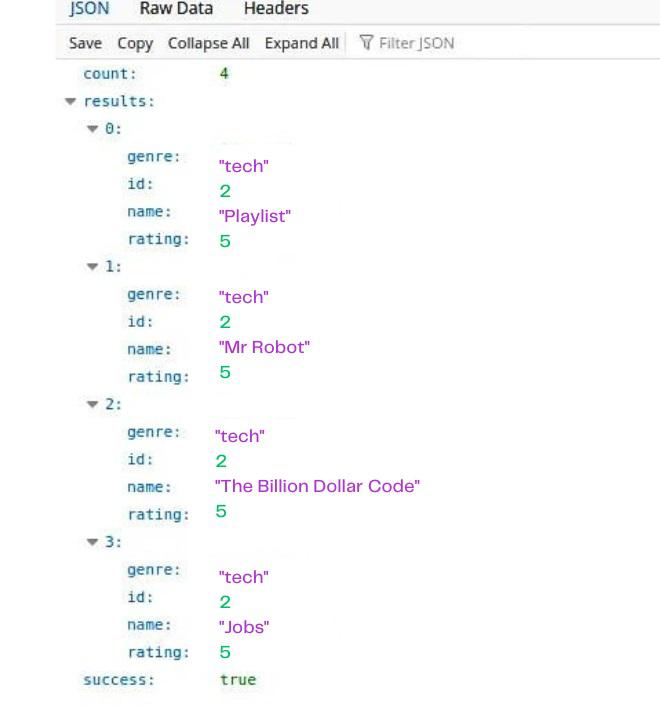
Â
Implement Sorting in Flask
Sorting is the process of grouping the results of your query so that you may analyze them. Data can be sorted in:
- Ordered alphabetically
- Counting order
Let’s order our films based on the highest-rated films rather than categories like year and so on. The code below displays a list of the top five films:
@app.route('/rating')
def rating():
rate_movies = Movie.query.order_by(Movie.rating.desc()).limit(5).all()
results = [movie.format() for movie in rate_movies]
return jsonify({
'success':True,
'results':results,
'count':len(results)
})
Output:
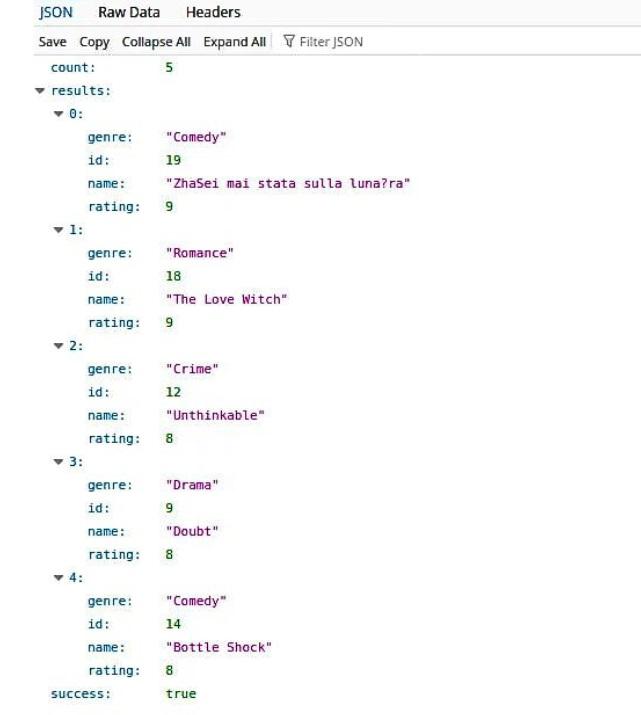
Â
Implement Pagination in Flask
It is not advisable to send large volumes of data all at once since the client will be slowed down as it waits for the server to recover all of that data. The ideal solution for this issue is to utilize request parameters to paginate the data and only provide the user the information they require, i.e., batches of information.
We’ll start by getting all of the movies from our database, then paginate and show only a handful of the results.
@app.route('/movies')
def movies():
movies = Movie.query.all()
print(movies)
results = [movie.format() for movie in movies]
return jsonify({
'success':True,
'results':results,
'count':len(results)
})
The aforementioned code demonstrates a straightforward route that retrieves all of the database’s movies and presents them in JSON format. Now that the server is running, you should be able to see a total of 22 movies on the website by going to http://127.0.0.1:5000/movies:
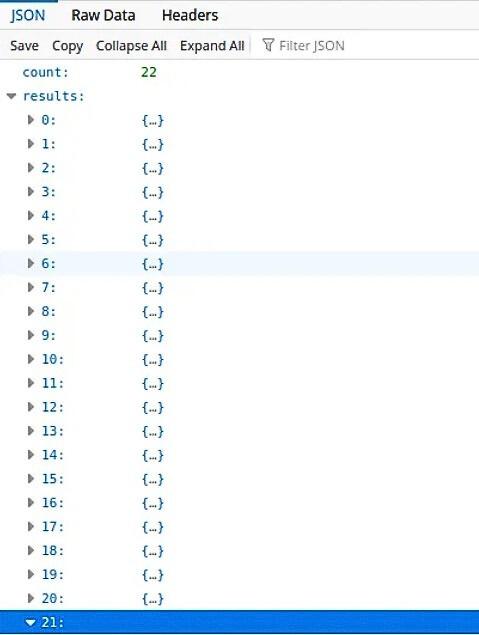
Â
Even though we only have a small number of films in our database, if we had more than a thousand films, the server would take longer to load and the user experience would suffer as a result.
The solution to this issue is to paginate the list of films. Thankfully, Flask has the paginate() query function, which we will use to obtain a small number of films.
The Paginate Query’s Syntax:
paginate(page=None, per_page=None, error_out=True, max_per_page=None)
Items per_page from the page are returned.
Let’s add pagination to our Flask application. Only five entries total should be returned on each page.
Output:
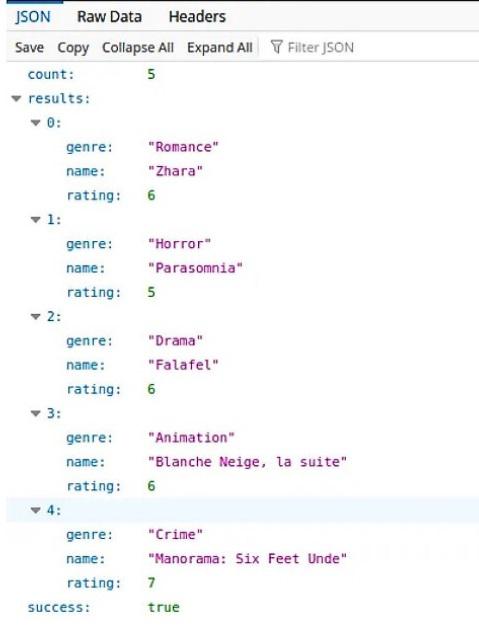
Â
Share your thoughts in the comments
Please Login to comment...