How to Implement Bottom Navigation With Activities in Android?
Last Updated :
28 Feb, 2022
We all have come across apps that have a Bottom Navigation Bar. Some popular examples include Instagram, WhatsApp, etc. Below is the preview of a sample Bottom Navigation Bar:
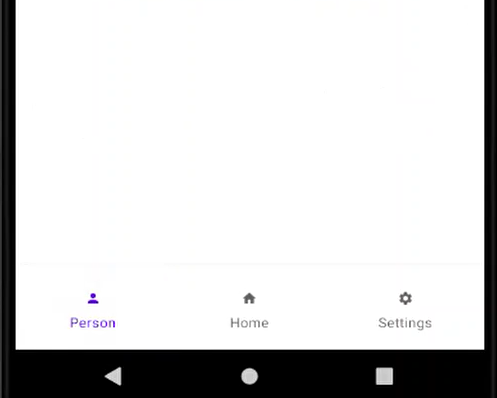
You must have seen the implementation of Bottom Navigation with the help of fragments. But, here we are going to implement Bottom Navigation with the help of activities in Android Studio.
What we are going to build in this application?
Here is a sample video of what we are going to build in this application. Note that we will be using Java language to make this application.
Step by Step Implementation
Step 1: Create a New Project
- Open a new project.
- We will be working on Empty Activity with language as Java. Leave all other options unchanged.
- Name the application at your convenience.
- There will be two default files named activity_main.xml and MainActivity.java.
If you don’t know how to create a new project in Android Studio then you can refer to How to Create/Start a New Project in Android Studio?
Step 2. Adding required dependency
Navigate to Gradle Scripts > gradle.scripts(module) and add the following dependency in it.
implementation 'com.google.android.material:material:1.0.0'
Step 3. Working on XML files
Navigate to app > res > drawable > right-click > new > drawable resource file and name it as “selector”. Use the following code in selector.xml file-
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< item
android:state_selected = "true"
android:color = "@color/white" />
< item
android:state_selected = "false"
android:color = "@color/black" />
</ selector >
|
Navigate to app > right-click > new > android resource file of type menu and name it as “menu_navigation”. Use the following code in menu_navigation.xml file-
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< item
android:id = "@+id/dashboard"
android:title = "Dashboard"
android:icon = "@drawable/dashboard" />
< item
android:id = "@+id/home"
android:title = "Home"
android:icon = "@drawable/home" />
< item
android:id = "@+id/about"
android:title = "About"
android:icon = "@drawable/about" />
</ menu >
|
Navigate to the app > res > layout > activity_main.xml and add the below code to that file. Below is the code for the activity_main.xml file.
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< RelativeLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent"
tools:context = ".MainActivity" >
< TextView
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Home"
android:textSize = "50sp"
android:textStyle = "bold"
android:layout_centerInParent = "true" />
< com.google.android.material.bottomnavigation.BottomNavigationView
android:id = "@+id/bottom_navigation"
android:layout_width = "match_parent"
android:layout_height = "60dp"
android:layout_alignParentBottom = "true"
app:itemBackground = "@color/teal_700"
app:itemIconTint = "@drawable/selector"
app:itemTextColor = "@drawable/selector"
app:menu = "@menu/menu_navigation" />
</ RelativeLayout >
|
Navigate to app > new > right-click > new > activity > empty activity. Name it as “Dashboard” and use the following code in activity_dashboard.xml file-
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< RelativeLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent"
tools:context = ".DashBoard" >
< TextView
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Dashboard"
android:textSize = "50sp"
android:textStyle = "bold"
android:layout_centerInParent = "true" />
< com.google.android.material.bottomnavigation.BottomNavigationView
android:id = "@+id/bottom_navigation"
android:layout_width = "match_parent"
android:layout_height = "60dp"
android:layout_alignParentBottom = "true"
app:itemBackground = "@color/teal_700"
app:itemIconTint = "@drawable/selector"
app:itemTextColor = "@drawable/selector"
app:menu = "@menu/menu_navigation" />
</ RelativeLayout >
|
Navigate to app > new > right-click > new > activity > empty activity. Name it as “About” and use the following code in activity_about.xml file
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< RelativeLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent"
tools:context = ".About" >
< TextView
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "About"
android:textSize = "50sp"
android:textStyle = "bold"
android:layout_centerInParent = "true" />
< com.google.android.material.bottomnavigation.BottomNavigationView
android:id = "@+id/bottom_navigation"
android:layout_width = "match_parent"
android:layout_height = "60dp"
android:layout_alignParentBottom = "true"
app:itemBackground = "@color/teal_700"
app:itemIconTint = "@drawable/selector"
app:itemTextColor = "@drawable/selector"
app:menu = "@menu/menu_navigation" />
</ RelativeLayout >
|
Step 4. Working on Java files
Navigate to the MainActivity.java file and use the following code in it. Comments are added to the code to have a better understanding.
Java
package com.example.bottomnavigationwithactivities;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.MenuItem;
import com.google.android.material.bottomnavigation.BottomNavigationView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
BottomNavigationView bottomNavigationView=findViewById(R.id.bottom_navigation);
bottomNavigationView.setSelectedItemId(R.id.home);
bottomNavigationView.setOnNavigationItemSelectedListener( new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected( @NonNull MenuItem item) {
switch (item.getItemId())
{
case R.id.dashboard:
startActivity( new Intent(getApplicationContext(),DashBoard. class ));
overridePendingTransition( 0 , 0 );
return true ;
case R.id.home:
return true ;
case R.id.about:
startActivity( new Intent(getApplicationContext(),About. class ));
overridePendingTransition( 0 , 0 );
return true ;
}
return false ;
}
});
}
}
|
Navigate to the DashBoard.java file and use the following code in it. Comments are added to the code to have a better understanding.
Java
package com.example.bottomnavigationwithactivities;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.MenuItem;
import com.google.android.material.bottomnavigation.BottomNavigationView;
public class DashBoard extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.activity_dash_board);
BottomNavigationView bottomNavigationView=findViewById(R.id.bottom_navigation);
bottomNavigationView.setSelectedItemId(R.id.dashboard);
bottomNavigationView.setOnNavigationItemSelectedListener( new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected( @NonNull MenuItem item) {
switch (item.getItemId())
{
case R.id.home:
startActivity( new Intent(getApplicationContext(),MainActivity. class ));
overridePendingTransition( 0 , 0 );
return true ;
case R.id.dashboard:
return true ;
case R.id.about:
startActivity( new Intent(getApplicationContext(),About. class ));
overridePendingTransition( 0 , 0 );
return true ;
}
return false ;
}
});
}
}
|
Navigate to the About.java file and use the following code in it. Comments are added to the code to have a better understanding.
Java
package com.example.bottomnavigationwithactivities;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.MenuItem;
import com.google.android.material.bottomnavigation.BottomNavigationView;
public class About extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.activity_about);
BottomNavigationView bottomNavigationView=findViewById(R.id.bottom_navigation);
bottomNavigationView.setSelectedItemId(R.id.about);
bottomNavigationView.setOnNavigationItemSelectedListener( new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected( @NonNull MenuItem item) {
switch (item.getItemId())
{
case R.id.dashboard:
startActivity( new Intent(getApplicationContext(),DashBoard. class ));
overridePendingTransition( 0 , 0 );
return true ;
case R.id.about:
return true ;
case R.id.home:
startActivity( new Intent(getApplicationContext(),MainActivity. class ));
overridePendingTransition( 0 , 0 );
return true ;
}
return false ;
}
});
}
}
|
Here is the final output of our application.
Output:
Share your thoughts in the comments
Please Login to comment...