How to Hide Tooltip After N Milliseconds in React-Bootstrap ?
Last Updated :
18 Oct, 2023
In this article, we will see how to hide the Tooltip after N milliseconds using React-Bootstrap. In web applications, a tooltip provides additional information when a user hovers over an element. However, for better user experience and control, there may be a need to hide the tooltip after N milliseconds. In this article, we will implement the same functionality using ReactJS and Bootstrap.
Prerequisites:
Creating React Application And Installing Modules:
Step 1: Create a React application using the following command
npx create-react-app folderName
Step 2: Navigate to the project folder using the following command
cd folderName
Step 3: Now install React-bootstrap and bootstrap
npm install react-bootstrap bootstrap
Step 4: Add Bootstrap CSS to index.js
import 'bootstrap/dist/css/bootstrap.min.css';
Project Structure:
The updated dependencies in package.json will look like this:
{
"name": "tooltipapp",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-bootstrap": "^2.9.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
}
Example: Now write down the following code in App.js File. Here App is our default component where we have written our code.
Javascript
import React, { useState } from "react" ;
import { OverlayTrigger, Tooltip,
Container } from "react-bootstrap" ;
const App = () => {
const [showToolTip, setShowToolTip] = useState( false );
const handleMouseEnter = () => {
setShowToolTip( true );
};
const handleToolTipToggle = (show) => {
if (show) {
setTimeout(() => {
setShowToolTip( false );
}, 3000);
}
};
return (
<div
className= "d-flex justify-content-center
align-items-center vh-100"
>
<OverlayTrigger
trigger= "hover"
placement= "bottom"
overlay={
<Tooltip className= "custom-tooltip" >
<div>This is a tooltip!</div>
</Tooltip>
}
show={showToolTip}
onToggle={handleToolTipToggle}
>
<div
onMouseEnter={handleMouseEnter}
className= "p-2 bg-primary text-white
rounded cursor-pointer"
>
Hover over me to show tooltip
</div>
</OverlayTrigger>
</div>
);
};
export default App;
|
Steps to Run Application :
Step 1: Run the Application using following command from the root directory of the project :
npm start
Step 2: Open web-browser and type the following URL
http://localhost:3000/
Output:
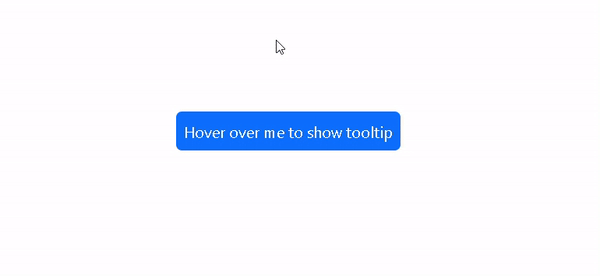
Hide tooltip after x milliseconds
Share your thoughts in the comments
Please Login to comment...