How to Hide the X-Axis Label/text that is Displayed in Chart.js ?
Last Updated :
04 Jan, 2024
Chart.js is a popular JavaScript library for creating interactive and visually appealing charts and graphs. By default, Chart.js displays text labels for both the x and y axes but in this article, we will see the different approaches to hiding the x-axis label/text that is displayed in chart.js.
Chart.js CDN Link
To use Chart.js you have to include the below CDN link in your HTML.
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
Using display: false property
In this approach, we set the display property to ‘false’ for the X-axis inside the scales object of the options object.
Syntax:
scales: {
x: {
display: false // Hides labels and chart lines of the x-axis
}
}
//OR
scales: {
x: {
ticks:{
display: false // Hides only the labels of the x-axis
}
}
}
Example: The below code example uses the display: false property to hide the x-axis labels in chart.js.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Chart.js Display: None
</ title >
< script src =
</ script >
< script src =
</ script >
</ head >
< body >
< center >
< div >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< h3 >
Chart.js Line Chart with Hidden
X-Axis Labels (Display: None)
</ h3 >
< div >
< canvas id = "lineChartID-display-none" >
</ canvas >
</ div >
</ div >
</ center >
< script >
new Chart($("#lineChartID-display-none"), {
type: 'line',
data: {
labels:
['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [{
label: 'My Dataset',
data:
[65, 71, 62, 81, 34, 55, 47],
borderColor: 'green',
fill: false,
tension: 0.1
}]
},
options: {
scales: {
x: {
// ticks:{
// display: false
// }
display: false
},
y: {
beginAtZero: true
}
}
}
});
</ script >
</ body >
</ html >
|
Output:
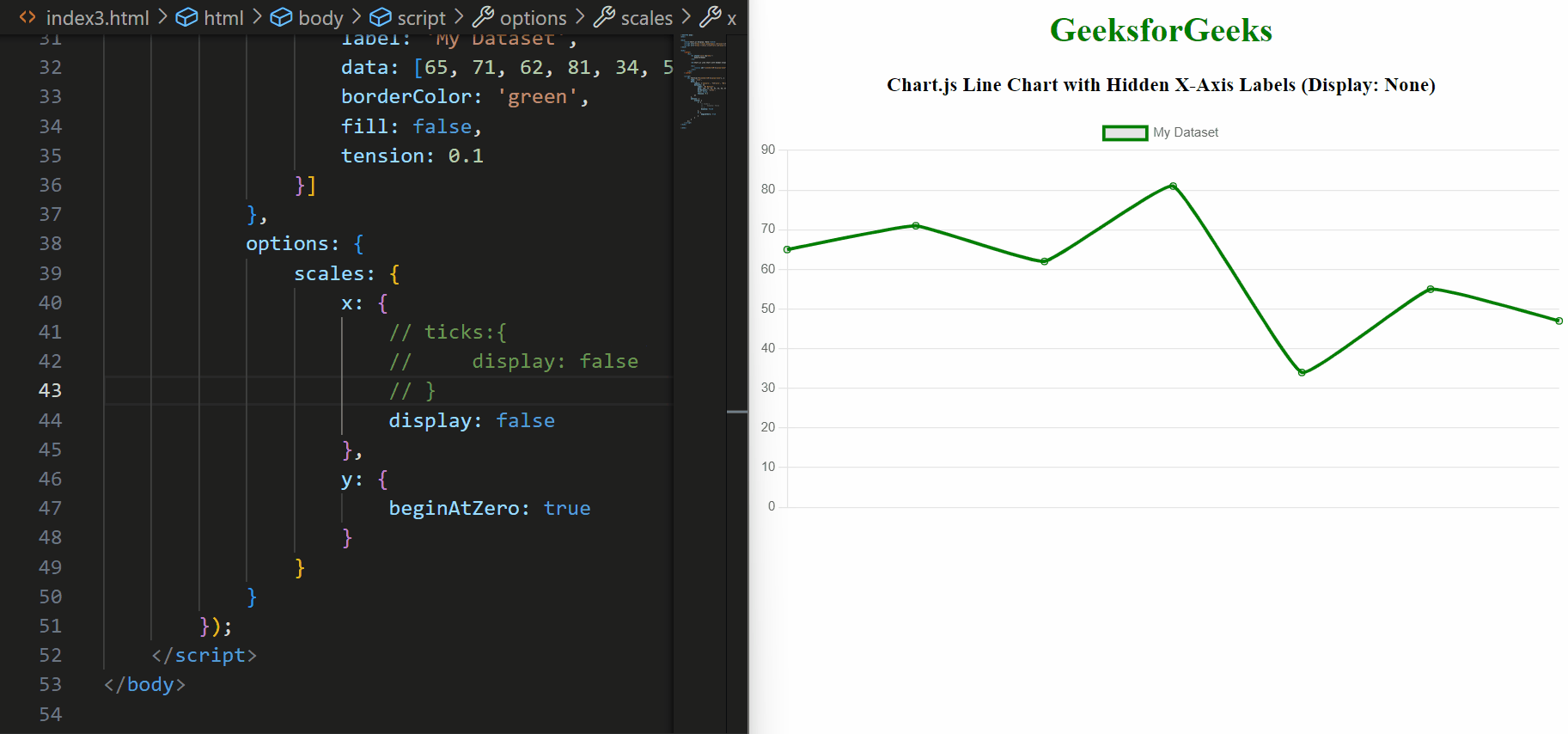
Using the Callback Function
In this approach we use a callback function to control the display of X-axis labels dynamically. This method allows for more granular control over which labels are shown and which are hidden based on custom conditions defined in the callback function.
Syntax:
scales:{
x:{
ticks:{
// Callback function
}
}
}
Example: The below example implements the callback function approach to hide the x-axis labels in chart.js.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Chart.js Callback Function
</ title >
< script src =
</ script >
< script src =
</ script >
</ head >
< body >
< div >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< h3 >
Chart.js Line Chart with Hidden
X-Axis Labels (Callback Function)
</ h3 >
< div >
< canvas id = "lineChartID-callback-function" >
</ canvas >
</ div >
</ div >
< script >
new Chart($("#lineChartID-callback-function"), {
type: 'line',
data: {
labels:
['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [{
label: 'My Dataset',
data:
[65, 41, 62, 81, 14, 75, 47],
borderColor: 'green',
fill: false,
tension: 0.1
}]
},
options: {
scales: {
x: {
ticks: {
callback:
function (value, index, values) {
return "";
}
}
},
y: {
beginAtZero: true
}
}
}
});
</ script >
</ body >
</ html >
|
Output:
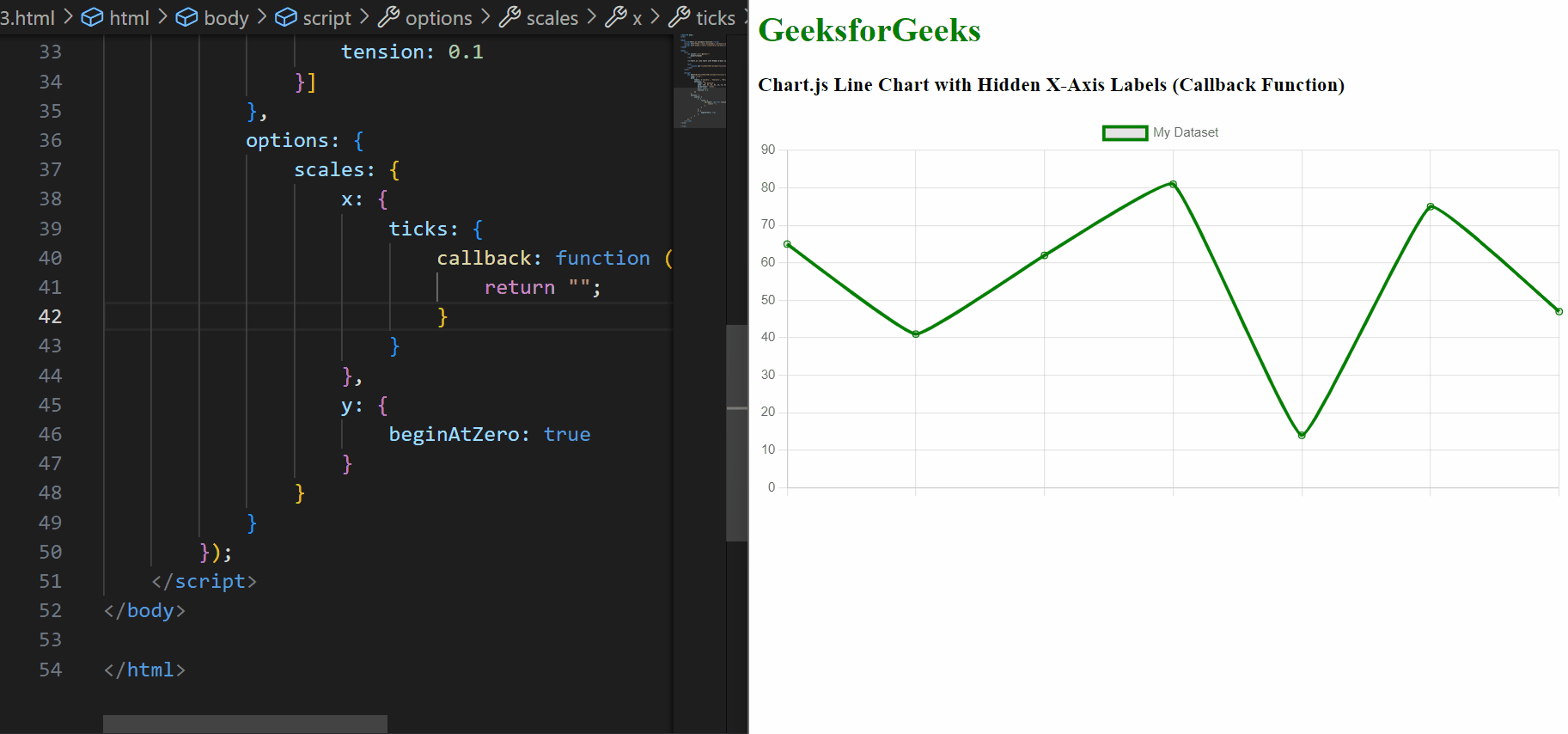
Share your thoughts in the comments
Please Login to comment...