How to hide all rows except selected one without knowing complete ID in JavaScript ?
Last Updated :
09 May, 2023
Given an HTML table with a set number of rows, the task is to hide all the rows except the selected one without knowing its ID using JavaScript.
Approach: Using the querySelectorAll(), forEach(), addEventListener() and filter() methods & the spread operator (…) in native JavaScript. Firstly, we select all the table rows expect the header row using the querySelectorAll() method, passing in the tr:not(:first-child) selector as a string parameter. We iterate through each table row using the forEach() method. Now, for every table row, an event listener is attached to it using the addEventListener() method with the event being the click event.
Inside the event listener, a variable row is declared which stores all the table row elements in the form of an array as the spread operator is used to convert the node list returned from the querySelectorAll() method to an array. We filter certain rows from all rows using the filter() method, passing a parameter element within it. The condition to filter the rows is that if the row is not clicked, we should hide it using the display property which is set to the value of none.
Example: In this example, we are using the above-explained approach.
HTML
<!DOCTYPE html>
< html >
< head >
< style >
body {
text-align: center;
}
h1 {
color: green;
font-size: 2.5rem;
}
p {
font-size: 1.5rem;
font-weight: bold;
}
table {
margin: 0 auto;
}
table,
th,
td {
border: 1px solid black;
border-collapse: collapse;
}
th,
td {
padding: 0.5rem;
}
tr:not(:first-child) {
cursor: pointer;
}
tr:not(:first-child):hover {
background: green;
color: white;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< p >JavaScript - Hide all rows except
selected one without ID
</ p >
< table >
< tr >
< th >Book Name</ th >
< th >Author Name</ th >
< th >Genre</ th >
</ tr >
< tr >
< td >The Book Thief</ td >
< td >Markus Zusak</ td >
< td >Historical Fiction</ td >
</ tr >
< tr >
< td >The Cruel Prince</ td >
< td >Holly Black</ td >
< td >Fantasy</ td >
</ tr >
< tr >
< td >The Silent Patient</ td >
< td >Alex Michaelides</ td >
< td >Psychological Fiction</ td >
</ tr >
</ table >
< script >
document.querySelectorAll(
// Select all rows except the first one
"tr:not(:first-child)").forEach(function (e) {
// Add onClick event listener
// to selected rows
e.addEventListener("click", function () {
// Get all rows except the first one
var rows =
[...document.querySelectorAll(
"tr:not(:first-child)"
)];
var notSelectedRows =
rows.filter(function (element) {
// Hide the rows that have
// not been clicked
if (element !== e) {
element.style.display = "none";
}
});
});
});
</ script >
</ body >
</ html >
|
Output:
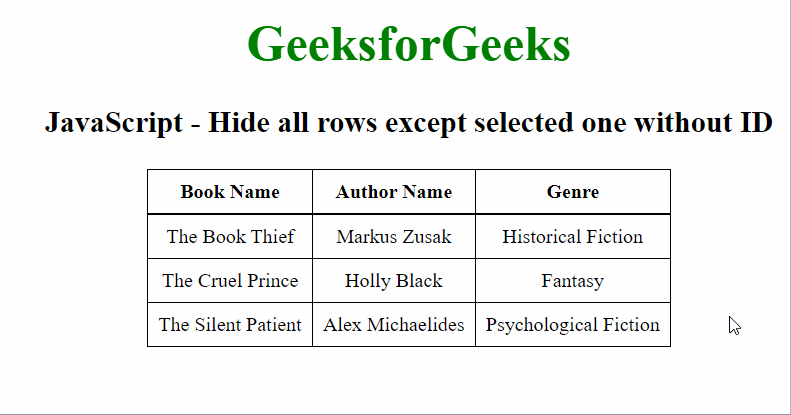
Share your thoughts in the comments
Please Login to comment...