How to Get the Size of a File in Java?
Last Updated :
07 Feb, 2024
In Java, file transferring, retrieving content of files, and manipulation of files developers frequently require the size of the file to perform proper file handling. In Java, there are many ways to get the size of the file.
In this article, we will explore the most commonly used approaches to get the size of the file in Java.
Program to Get the Size of a File in Java
1. Using File Class
Let’s explore how to get the file size using File Class.
Java
import java.io.File;
class MyClass {
static final String PATH = "C:\\Users\\Dhanush\\frontend.txt" ;
public static void main(String args[])
{
File file = new File(PATH);
if ((file.exists()) && (file.isFile()))
{
System.out.println( "The File Size in bytes : " +convertToBytes(file)+ " bytes" );
System.out.println( "The File Size in KiloBytes : " +convertToKilobytes(file)+ "kb" );
System.out.println( "The File Size in MegaBytes : " +convertToMegabytes(file)+ "mb" );
}
else {
System.out.println( "OOPs! The Specified File not found." );
}
}
private static long convertToMegabytes(File file)
{
return file.length() / ( 1024 * 1024 );
}
private static long convertToKilobytes(File file)
{
return file.length() / 1024 ;
}
private static long convertToBytes(File file)
{
return file.length();
}
}
|
Output:
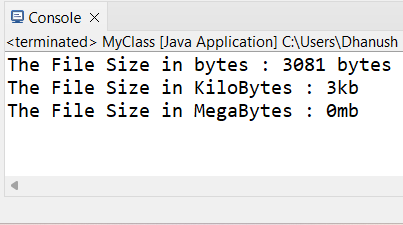
Explanation of the Program:
- In this approach, implemented the .length() function to get the size of the file.
- Firstly it checks if the file exists or the file specified is of the type file.
- Then by using .length() function the length of the file with respective size type(mb,kb and bytes) the size will be displayed.
2. Using FileChannel Class
Let’s explore how to get the file size using FileChannel Class.
Java
import java.io.File;
import java.io.IOException;
import java.nio.channels.FileChannel;
import java.nio.file.StandardOpenOption;
public class MyClass {
static final String PATH = "C:\\Users\\Dhanush\\frontend.txt" ;
public static void main(String args[]) throws IOException {
File file = new File(PATH);
if (file.exists() && file.isFile()) {
try (FileChannel channel = FileChannel.open(file.toPath(), StandardOpenOption.READ)) {
long fileSize = channel.size();
System.out.println( "File size : " + fileSize + " bytes" );
}
} else {
System.out.println( "OOPs! The specified file not found." );
}
}
}
|
Output:
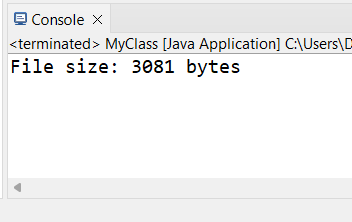
Explanation of the Program:
- In this approach, implemented the FileChannel class to get the size of the file.
- Firstly, it checks if the file exists, or the file specified is of the type of file.
- By using the .size() function the size of the file will be displayed in bytes by default.
Note: Both the approaches have been written and executed in eclipse IDE.
Share your thoughts in the comments
Please Login to comment...