How to get selected value in dropdown list using JavaScript ?
Last Updated :
03 May, 2024
Dropdown lists (also known as select elements) are commonly used in web forms to allow users to choose from a predefined set of options. When a user selects an option from the dropdown, we often need to retrieve the chosen value programmatically.
The dropdown list is created using the <select> tab with <option> tab and for selecting the value we will use the below methods.
We can get the values using the below methods:
1. Using the value property
- The value of the selected element can be found by using the value property on the selected element that defines the list.
- This property returns a string representing the value attribute of the <option> element in the list.
- If no option is selected then nothing will be returned.
Syntax:
selectedSelectElement.value;
Example: The below code uses the value property to get the value of the selected dropdown item.
HTML
<!DOCTYPE html>
<html>
<head>
<title>
How to get selected value in
dropdown list using JavaScript?
</title>
</head>
<body>
<h1 style="color: green">
GeeksforGeeks
</h1>
<b>
How to get selected value in dropdown
list using JavaScript?
</b>
<p> Select one from the given options:
<select id="select1">
<option value="free">Free</option>
<option value="basic">Basic</option>
<option value="premium">Premium</option>
</select>
</p>
<p> The value of the option selected is:
<span class="output"></span>
</p>
<button onclick="getOption()"> Check option </button>
<script type="text/javascript">
function getOption() {
selectElement =
document.querySelector('#select1');
output = selectElement.value;
document.querySelector('.output').textContent = output;
}
</script>
</body>
</html>
Output:
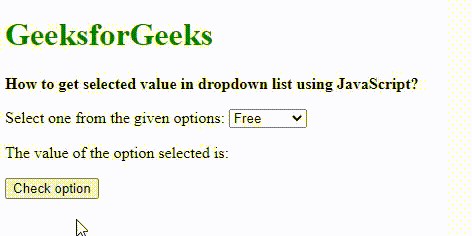
value property
2. Using the selectedIndex property
- The selectedIndex property returns the index of the currently selected element in the dropdown list. This index starts from 0 and returns -1 if no option is selected.
- The options property returns the collection of all the option elements in the <select> dropdown list.
- The elements are sorted according to the source code of the page.
- The index found before can be used with this property to get the selected element.
- This option’s value can be found by using the value property.
Syntax:
SelectElement.options[selectedSelectElement.selectedIndex].value;
Example: The eblow example implements the selectedIndex property to get the value of selected dropdown item.
HTML
<!DOCTYPE html>
<html>
<head>
<title>
How to get selected value in
dropdown list using JavaScript?
</title>
</head>
<body>
<h1 style="color: green">
GeeksforGeeks
</h1>
<b>
How to get selected value in
dropdown list using JavaScript?
</b>
<p> Select one from the given options:
<select id="select1">
<option value="free">Free</option>
<option value="basic">Basic</option>
<option value="premium">Premium</option>
</select>
</p>
<p> The value of the option selected is:
<span class="output"></span>
</p>
<button onclick="getOption()">Check option</button>
<script type="text/javascript">
function getOption() {
selectElement =
document.querySelector('#select1');
output =
selectElement.options
[selectElement.selectedIndex].value;
document.querySelector('.output').textContent = output;
}
</script>
</body>
</html>
Output:
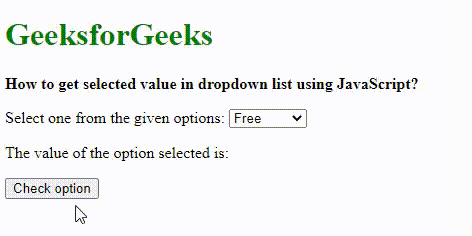
SelectedIndex property with option property
3. Using the jQuery val() method
jQuery is a JavaScript library whose val() method can be used to get the value of the selected dropdown item.
Syntax:
selectedSelectElement.val();
Example: The below code explains the use of the jQuery val() method to get the value of the selected item.
HTML
<!DOCTYPE html>
<html>
<head>
<title>
How to get selected value in
dropdown list using JavaScript?
</title>
</head>
<body>
<h1 style="color: green">
GeeksforGeeks
</h1>
<b>
How to get selected value in
dropdown list using JavaScript?
</b>
<p> Select one from the given options:
<select id="select1">
<option value="free">Free</option>
<option value="basic">Basic</option>
<option value="premium">Premium</option>
</select>
</p>
<p> The value of the option selected is:
<span class="output"></span>
</p>
<button onclick="getOption()">Check option</button>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/jquery/3.7.1/jquery.min.js"
integrity=
"sha512-v2CJ7UaYy4JwqLDIrZUI/4hqeoQieOmAZNXBeQyjo21dadnwR+8ZaIJVT8EE2iyI61OV8e6M8PP2/4hpQINQ/g=="
crossorigin="anonymous"
referrerpolicy="no-referrer">
</script>
<script type="text/javascript">
function getOption() {
const output = $('#select1').val();
$('.output').text(output);
}
</script>
</body>
</html>
Output:
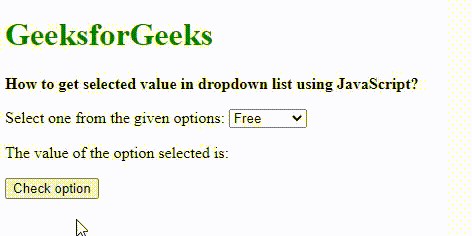
Share your thoughts in the comments
Please Login to comment...