How to Flatten MultiIndex in Pandas?
Last Updated :
15 Dec, 2022
In this article, we will discuss how to flatten multiIndex in pandas.
Flatten all levels of MultiIndex:
In this method, we are going to flat all levels of the dataframe by using the reset_index() function.
Syntax:
dataframe.reset_index(inplace=True)
Note: Dataframe is the input dataframe, we have to create the dataframe MultiIndex.
Syntax:
MultiIndex.from_tuples([(tuple1),.......,(tuple n),names=[column_names])
Arguments:
- tuples are the values
- column names are the names of columns in each tuple value
Example:
In this example, we will create a dataframe along with multiIndex and display it in the python programming language.
Python3
import pandas as pd
data = pd.MultiIndex.from_tuples([( 'Web Programming' , 'php' , 'sub1' ),
( 'Scripting' , 'python' , 'sub2' ),
( 'networks' , 'computer network' , 'sub3' ),
( 'architecture' , 'computer organization' , 'sub4' ),
( 'coding' , 'java' , 'sub5' )],
names = [ 'Course' , 'Subject name' , 'subject id' ])
data = pd.DataFrame({ 'ravi' : [ 98 , 89 , 90 , 88 , 93 ],
'reshma' : [ 78 , 89 , 80 , 98 , 63 ],
'sahithi' : [ 78 , 89 , 80 , 98 , 63 ]},
index = data)
data
|
Output:
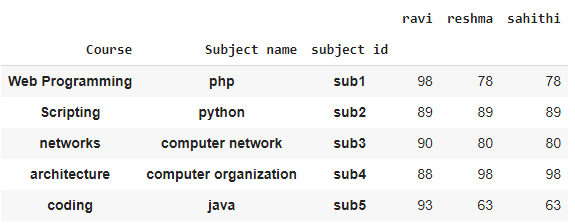
Now, we will flatten the index of all levels:
Python3
import pandas as pd
data = pd.MultiIndex.from_tuples([( 'Web Programming' , 'php' , 'sub1' ),
( 'Scripting' , 'python' , 'sub2' ),
( 'networks' , 'computer network' , 'sub3' ),
( 'architecture' , 'computer organization' , 'sub4' ),
( 'coding' , 'java' , 'sub5' )],
names = [ 'Course' , 'Subject name' , 'subject id' ])
data = pd.DataFrame({ 'ravi' : [ 98 , 89 , 90 , 88 , 93 ],
'reshma' : [ 78 , 89 , 80 , 98 , 63 ],
'sahithi' : [ 78 , 89 , 80 , 98 , 63 ]},
index = data)
data.reset_index(inplace = True )
data
|
Output:
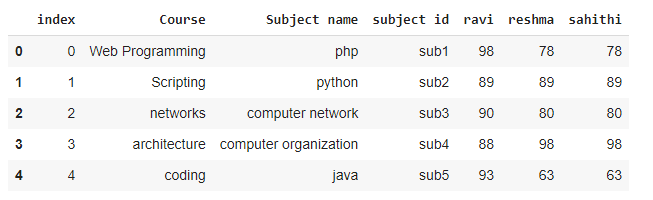
Flatten specific levels of MultiIndex
By using specific levels we can get by using the following syntax:
dataframe.reset_index(inplace=True,level=['level_name'])
where
- dataframe is the input dataframe
- level_name is the name of the multiindex level
Example:
In this example, we will create a dataframe and flatten specific levels of multiIndex and display it in the python programming language.
Python3
import pandas as pd
data = pd.MultiIndex.from_tuples([( 'Web Programming' , 'php' , 'sub1' ),
( 'Scripting' , 'python' , 'sub2' ),
( 'networks' , 'computer network' , 'sub3' ),
( 'architecture' , 'computer organization' , 'sub4' ),
( 'coding' , 'java' , 'sub5' )],
names = [ 'Course' , 'Subject name' , 'subject id' ])
data = pd.DataFrame({ 'ravi' : [ 98 , 89 , 90 , 88 , 93 ],
'reshma' : [ 78 , 89 , 80 , 98 , 63 ],
'sahithi' : [ 78 , 89 , 80 , 98 , 63 ]},
index = data)
data.reset_index(inplace = True , level = [ 'Course' ])
data
|
Output:
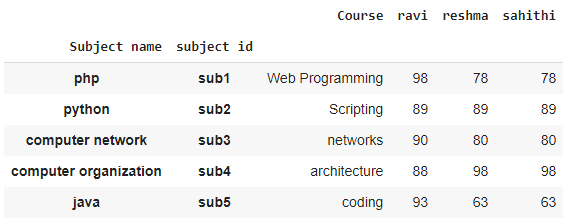
We can also specify multiple levels;
Python3
import pandas as pd
data = pd.MultiIndex.from_tuples([( 'Web Programming' , 'php' , 'sub1' ),
( 'Scripting' , 'python' , 'sub2' ),
( 'networks' , 'computer network' , 'sub3' ),
( 'architecture' , 'computer organization' , 'sub4' ),
( 'coding' , 'java' , 'sub5' )],
names = [ 'Course' , 'Subject name' , 'subject id' ])
data = pd.DataFrame({ 'ravi' : [ 98 , 89 , 90 , 88 , 93 ],
'reshma' : [ 78 , 89 , 80 , 98 , 63 ],
'sahithi' : [ 78 , 89 , 80 , 98 , 63 ]},
index = data)
data.reset_index(inplace = True , level = [ 'Course' , 'subject id' ])
data
|
Output:
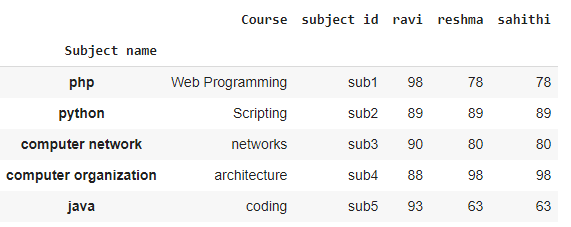
Using to_records() method
This is a pandas module method used to convert multiindex dataframe into each record and display.
Syntax:
dataframe.to_records()
Example:
Python3
import pandas as pd
data = pd.MultiIndex.from_tuples([( 'Web Programming' , 'php' , 'sub1' ),
( 'Scripting' , 'python' , 'sub2' ),
( 'networks' , 'computer network' , 'sub3' ),
( 'architecture' , 'computer organization' , 'sub4' ),
( 'coding' , 'java' , 'sub5' )],
names = [ 'Course' , 'Subject name' , 'subject id' ])
data = pd.DataFrame({ 'ravi' : [ 98 , 89 , 90 , 88 , 93 ],
'reshma' : [ 78 , 89 , 80 , 98 , 63 ],
'sahithi' : [ 78 , 89 , 80 , 98 , 63 ]},
index = data)
pd.DataFrame(data.to_records())
|
Output:
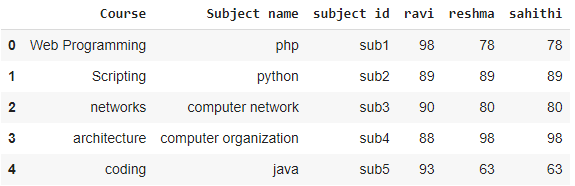
Share your thoughts in the comments
Please Login to comment...