In this article, we are going to understand the AttributeError: Object has no attribute error and then discuss the ways we can resolve this error. Generally, it is good practice to read and understand the error messages we encounter when writing our programs. Error messages act as a hint for us to improve the syntax and logic in our program. Let us start!
What is AttributeError: object has no attribute?
The “AttributeError: Object has no attribute” error is a common issue in Python. It occurs when we try to access an attribute of an object that doesn’t exist for that object. This error message provides valuable information for debugging our code.
syntax:
AttributeError: Object has no attribute
To understand this error, we first have to know how to read the error message effectively. It typically consists of two parts: “AttributeError” and “Object has no attribute.” The former indicates the type of error, and the latter suggests that the attribute we are trying to access does not exist for the object. Let us now proceed to discuss the common causes of this error.
Common Causes of Attribute Error
The main reasons why this error occurs are:
- Typo in attribute names.
- Attempting to access non-existent attributes.
- Incorrect usage of methods and attributes.
Let us go through each cause using an example followed by the explanation of why the error occurs. The ways to resolve is discussed in the following section.
We will be discussing on how to rectify the error in the next section. This section is reserved for our understanding in the common scenarios in which we encounter the “AttributeError”.
Accessing Non-Existent Attribute
We are defining a Dog class with a constructor to initialize the name attribute. The def __init__(self, name) is the class’s constructor method. It takes two parameters, self (the instance being created) and name (the dog’s name). Inside the constructor, we are initializing the name attribute of the Dog object with the value provided as name. Then we are creating a Dog object named my_dog with the name “Buddy”. But in the end we are trying to access the non-existent attribute “breed”. That is why we get an “AttributeError”.
Python3
class Dog:
def __init__( self , name):
self .name = name
my_dog = Dog( "Buddy" )
print (my_dog.breed)
|
Output:

The error message hints us that we are accessing a non-existent attribute ”breed”
Solution
In our first example we are trying to access a non-existing attribute breed. So, let us add an attribute breed or let us just access the attribute we have declared!
The non-existent attribute breed is removed and the existent attribute name is accessed instead. We can also create an attribute breed and then access it too! just like we did for the attribute name.
Python3
class Dog:
def __init__( self , name):
self .name = name
my_dog = Dog( "Buddy" )
print (my_dog.name)
|
Output:
Buddy
Typo in Attribute Name
We have defined a Person class with an __init__ method that takes three parameters: name, age, and hobby. This method initializes the name, age, and hobby attributes of the Person object. Then we are creating a Person object named Person_obj with specific values. However, it introduces a typo when trying to access the name attribute as nmae, resulting in an “AttributeError” since nmae is not a valid attribute.
Python3
class Person:
def __init__( self , name,age,hobby):
self .name = name
self .age = age
self .hobby = hobby
Person_obj = Person( "Manikarnika" , 19 , "Chess" )
print ( "Name: " ,Person_obj.nmae)
print ( "Age: " ,Person_obj.age)
print ( "Hobby: " ,Person_obj.hobby)
|
Output:

Typo in our Attribute name. Typed as ”nmae” instead of “name”
Solution
In our second example, we are having a typo error. We have typed ‘nmae’ instead of ‘name’, we just want to rectify that to make this code snippet right!
We have corrected the TYPO in our code snippet.
Python3
class Person:
def __init__( self , name,age,hobby):
self .name = name
self .age = age
self .hobby = hobby
Person_obj = Person( "Manikarnika" , 19 , "Chess" )
print ( "Name: " ,Person_obj.name)
print ( "Age: " ,Person_obj.age)
print ( "Hobby: " ,Person_obj.hobby)
|
Output
Name: Manikarnika
Age: 19
Hobby: Chess
Incorrect usage of methods and attributes
One important thing to note is that we use parenthesis “()” for methods but we do not use it for attributes. We get an error if we try to do so. We can use parenthesis “()” (to call) for classes and methods.
In this code, we have defined a Rectangle class with an __init__ method for initializing the width and height attributes. There’s another method called area that calculates the area of the rectangle. We have then created an instance of the Rectangle class called rect with dimensions 5 for width and 4 for height. However, when we try to access the width attribute using rect.width(), it results in an AttributeError because we are incorrectly using parentheses as if width is a method.
Python3
class Rectangle:
def __init__( self , width, height):
self .width = width
self .height = height
def area( self ):
return self .width * self .height
rect = Rectangle( 5 , 4 )
print (rect.width())
|
Output:

No () for attributes!
Solution:
In our third example, we are using the parentheses “()” to access an attribute which is actually wrong. We must not use a parentheses “()” to access an attribute it is only used while calling a class or a method.
Python3
class Rectangle:
def __init__( self , width, height):
self .width = width
self .height = height
def area( self ):
return self .width * self .height
rect = Rectangle( 5 , 4 )
print ( "The width: " ,rect.width)
|
Output:
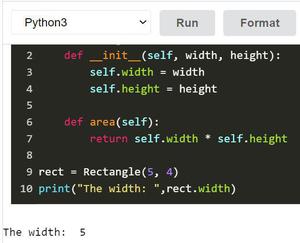
Compiled using GFG
The ‘hasattr()’ Technique in Python!
The hasattr() function is a built-in Python function that is used to check whether an object has a particular attribute or method.
We have included the “hasattr()” function inside the if-else to add logic to our code snippet. This will avoid the AttributeError: object has no attribute error. We can add the attribute after knowing that it does not exist in our code!
Python3
class Person:
def __init__( self , name):
self .name = name
person = Person( "Alice" )
if hasattr (person, 'age' ):
print (f "{person.name}'s age is {person.age}" )
else :
print ( "Age attribute does not exist." )
|
Output:
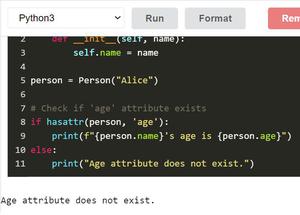
Compiled in GFG
Using try-except:
Using try-except block in our code is another useful method to catch and handle the errors. We can use that to fix the AttributeError!
Example for try-except block:
In try-except block, we place the code that we suspect to throw an error inside the block.
Python3
class Cat:
def __init__( self , name):
self .name = name
cat = Cat( "Whiskers" )
try :
print (cat.color)
except AttributeError as e:
print (f "AttributeError: {e}" )
|
Output:
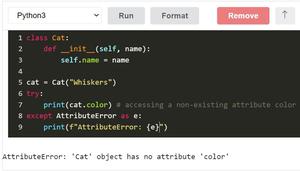
compiled in GFG
Some Other Ways to Resolve The Error
So, we have discussed about the “AttributeError”, the common causes for the error to occur and we have also understood the importance of reading the error message. Let’s now continue to discuss the ways we can resolve the error. Some of the common ways we can use are:
1 Check the Attribute Name
The most basic and the important thing is to check for the attribute name, because the typos and incorrect attribute names are the most common occurrence.
2. Verify Attribute Existence
In our examples, we have discussed about trying to access a non-existent attribute which eventually results in an AttributeError. So, it is important to know whether the attribute we are trying to access exist or not!
3. Verify Attribute Access Method
We have to make sure that we are using the correct syntax for attribute access. Parentheses () should not be used when accessing attributes, they are reserved for method calls. Attributes should be accessed without parentheses.
4. Check Class Definition
We also have to ensure that, the attribute we are trying to access is defined within the class.
5. Handling Missing Attributes
We can use techniques like hasattr() to check if the attribute exists before accessing it. This helps prevent AttributeError in cases where the attribute might not be present.
Conclusion:
Generally, In programming errors are like little roadblocks on our path to becoming better programmers. In this article, we’ve learned that reading error messages, avoiding common mistakes, and paying attention to details can help us overcome this error. It’s like solving a puzzle – each time we fix it, we get better at coding. With every error we conquer, we become more skilled Python programmers. Keep learning, keep trying, and remember that every error is a chance to get better at coding. Happy coding!
Share your thoughts in the comments
Please Login to comment...