How to find the total number of HTTP requests ?
Last Updated :
18 Dec, 2023
In this article, we will find the total number of HTTP requests through various approaches and methods like implementing server-side tracking by using PHP session variables or by analyzing the server logs to count and aggregate the incoming requests to the web application. By finding the total count of HTTP requests we can understand the web application metrics to optimize the application.
There are two approaches through which we can find the total number of HTTP requests.
Approach 1: Using Session Variable
In this approach, we are using the session variables to count and store details of each HTTP request, which includes the request method, time, and the IP address of the client. We are displaying the total number of requests and if there is more than one request then the list of detailed information for each of the requests is shown in the list bullet manner.
Example: In the below example, we will find the total number of HTTP requests in PHP using session variables.
PHP
<?php
session_start();
if (!isset( $_SESSION [ "request_count" ])) {
$_SESSION [ "request_count" ] = 1;
} else {
$_SESSION [ "request_count" ]++;
}
$requestDetails = [
"method" => $_SERVER [ "REQUEST_METHOD" ],
"timestamp" => date ( "Y-m-d H:i:s" ),
"ip" => $_SERVER [ "REMOTE_ADDR" ],
];
if (!isset( $_SESSION [ "request_details" ])) {
$_SESSION [ "request_details" ] = [];
}
$_SESSION [ "request_details" ][] = $requestDetails ;
echo "Total HTTP Requests: {$_SESSION[" request_count "]}<br>" ;
if ( $_SESSION [ "request_count" ] > 1) {
echo "<h3>Request Details:</h3>" ;
echo "<ul>" ;
foreach ( $_SESSION [ "request_details" ] as $request ) {
echo "<li>{$request[" method "]} request from {$request[" ip"]}
at { $request [ "timestamp" ]}</li>";
}
echo "</ul>" ;
}
?>
|
Output:
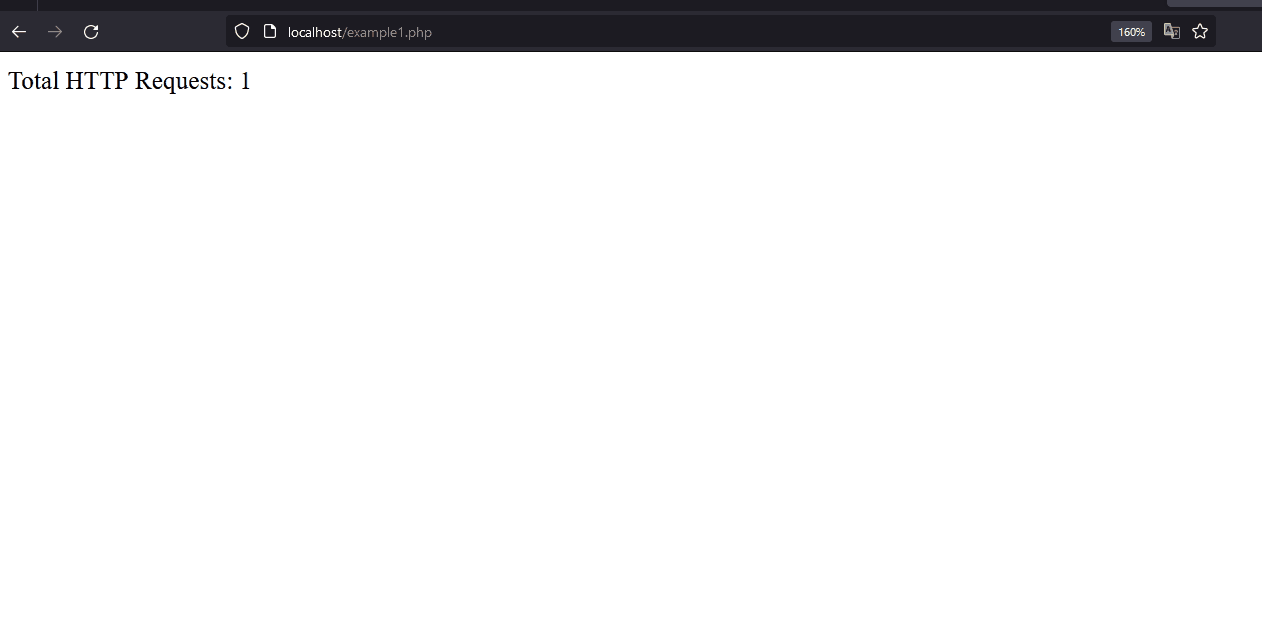
Approach 2: Using File based Counter
In this approach, we will write the PHP script which will store each of the HTTP request count values in the text file named “request_count.txt“. When the server is been requested with the HTTP request, the count value is increased and stored in the text file. So this makes sure that er are getting the updated value from the text file regarding the HTTP requests done on the server.
Example: In the below example, we will find the total number of HTTP requests in PHP using a File-based Counter.
PHP
<?php
function getReqCnt( $filePath )
{
if ( file_exists ( $filePath )) {
$reqCnt = intval ( file_get_contents ( $filePath ));
} else {
$reqCnt = 0;
}
return $reqCnt ;
}
function updateReqCnt( $filePath , $count )
{
file_put_contents ( $filePath , $count );
}
function displayRequestCount( $reqCnt )
{
echo "Total HTTP Requests: " . $reqCnt ;
}
$filePath = "request_count.txt" ;
try {
$reqCnt = getReqCnt( $filePath );
$reqCnt ++;
updateReqCnt( $filePath , $reqCnt );
displayRequestCount( $reqCnt );
} catch (Exception $e ) {
echo "Error: " . $e ->getMessage();
}
?>
|
Output:
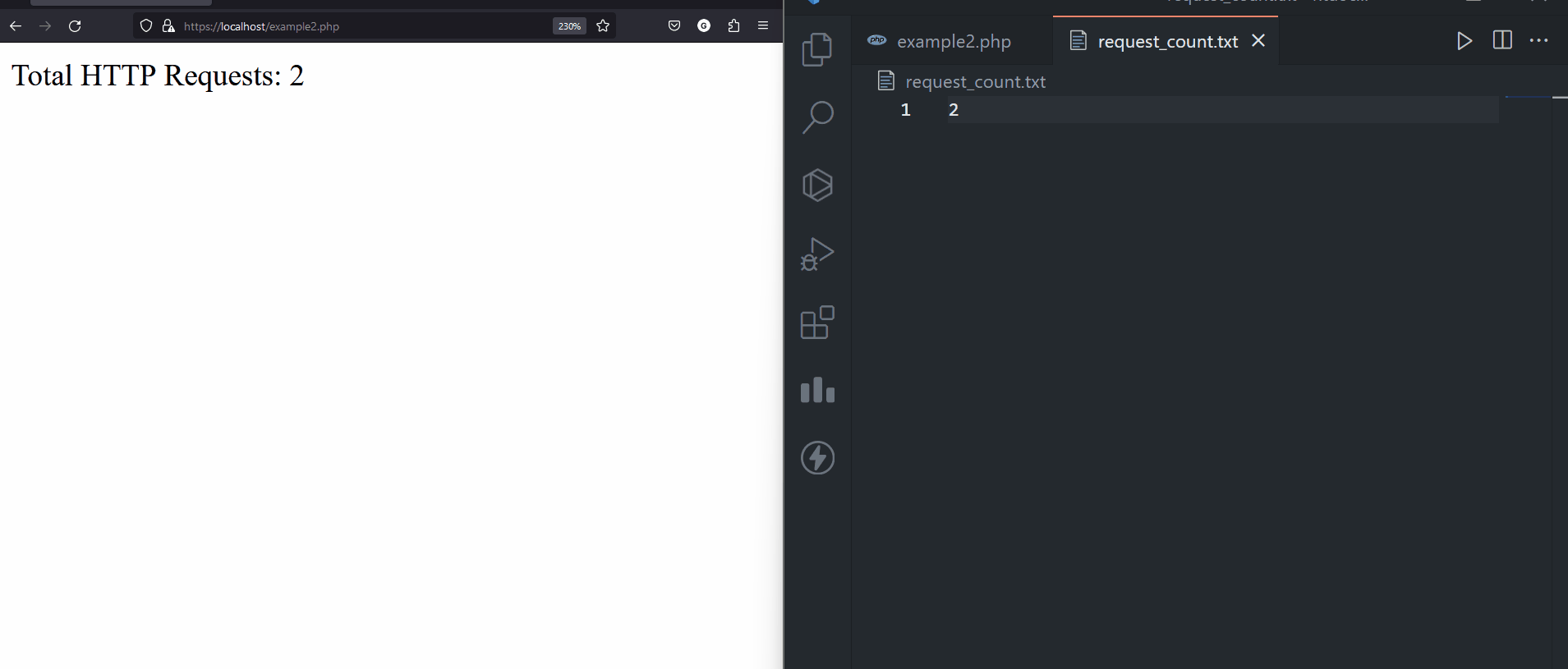
Share your thoughts in the comments
Please Login to comment...