How to dynamically create and apply CSS class in JavaScript ?
Last Updated :
15 Dec, 2023
To dynamically create a CSS class and apply it to the element dynamically using JavaScript, first, we create a class and assign it to HTML elements on which we want to apply CSS property. We can use className and classList properties in JavaScript.
Approach
The className property is used to add a class in JavaScript. It overwrites existing classes of the selected elements. If we don’t want to overwrite then we have to add a space before the class name.
// It overwrites existing classes
let h2 = document.querySelector("h2");
h2.className = "test";
// Add new class to existing classes
// Note space before new class name
h2.className = " test";
The classList property is also used to add a class in JavaScript but it never overwrites existing classes and adds a new class to the selected elements class list.
// Add new class to existing classes
let p = document.querySelector("p");
p.classList.add("test");
After adding new classes to the elements, we select the new class(test) and then apply CSS properties to it, with the help of style property in JavaScript.
// Select all elements with class test
let temp = document.querySelectorAll(".test");
// Apply CSS property to it
for (let i = 0; i < temp.length; i++) {
temp[i].style.color = "white";
temp[i].style.backgroundColor = "black";
}
Example: Below is the implementation of the above approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Document</ title >
</ head >
< body >
< h2 class = "hello" > Welcome to gfg </ h2 >
< p id = "hi" >Hi it's me.</ p >
< button onclick = "apply()" >
Apply css dynamically
</ button >
< script >
function apply() {
// It overwrites existing classes
let h2 = document.querySelector("h2");
h2.className = "test";
// Add new class to existing classes
let p = document.querySelector("p");
p.classList.add("test");
// Select all elements with class test
let temp = document.querySelectorAll(".test");
// Apply CSS property to it
for (let i = 0; i < temp.length ; i++) {
temp[i] .style.color = "green" ;
temp[i] .style.fontSize = "40px" ;
}
}
</script>
</ body >
</ html >
|
Output:
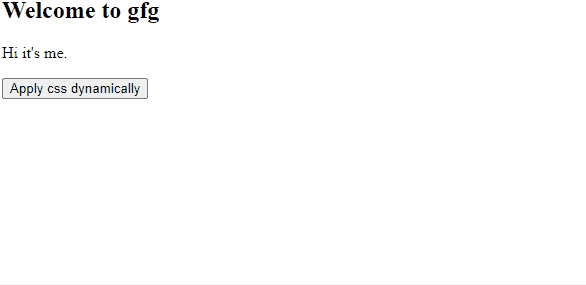
Dynamically create and apply CSS class
Share your thoughts in the comments
Please Login to comment...