How to do Unit Testing | Detailed Guide with Best Practices
Last Updated :
29 Feb, 2024
Unit testing is the testing process that is usually carried out by developers. It is used to test the piece of code or small components in the source code file by writing different test scenarios. This is the initial level of testing before handing it over to the testing team. It ensures the total code coverages achieved for the particular source code file. Each source code file will have a separate test file to execute all the test cases of that particular file to cover all the scenarios.
Setting Up Your Unit Testing Environment
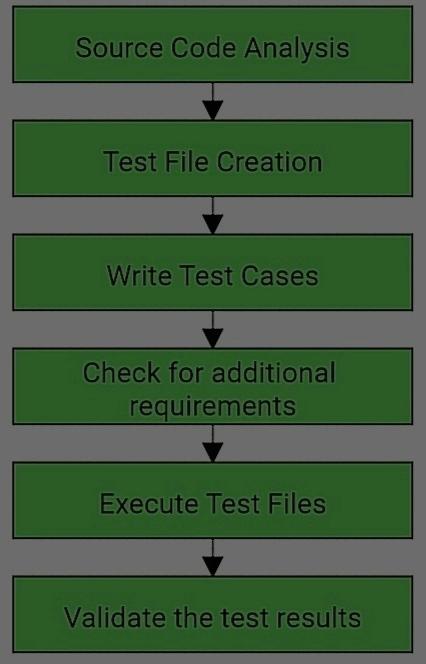
The following are the steps that we need to follow while setting up the test environment.
Step 1: Source Code Analysis
Analyze the source code to confirm how many test scenarios are there to cover and how many test cases are needed for each scenario. Plan for input and output to be specified in the test cases.
Step 2: Test File Creation
Create a separate test file for each source code file and save it with the relevant name under the correct test file location.
Step 3: Write Test Cases
Write the test cases to cover the different test scenarios of the particular source code file in that created test file.
Step 4: Check for Additional Requirements
Check if additional mock data are needed to cover some test cases to match the input with the expected output results.
Step 5: Execute Test Files
Once you are done with your test case, run the whole test file or run each test case as you want to know the coverages.
Step 6: Validate the Test Results
If any of the source codes are uncovered (which can be known after running the test case), try to write test cases for that and run the test cases again. If all test cases pass, then we are good to proceed with deployment. If any of the test cases failed, analyze and re-write the test cases
Writing Unit Tests
To write the test case, we need to know the source code and its functionality. We are going to write test cases for small code snippets. We need to cover different scenarios involved in that code snippet.
- The first step is to analyze, what are the statements we need to cover, and how many scenarios involved in the particular method or function or code snippet.
- Then, what are the inputs we need to pass for the test cases, and do we have to mention the expected output result for the same test case? Do this input and output specifications for different scenarios.
Example:
Let’s take a small piece of code. This method checkAge() is to check whether the person is eligible to vote or not and it has a conditional block for that as well with printing the age of that person. Let’s see how to write test cases for this method.
void checkAge(int age) {
if (age >=18)
print(“Eligible to Vote”)
else
print(“Not Eligible to Vote”)
print(age)
}
The above method contains the method with conditional and unconditional statements. So we have to cover all the statements.
- Test Case 1: Write the test case to call the function and cover the if condition by giving the age value greater than or equal to 18. This covers the if block statements i.e., it covers the conditional statements.
- Test Case 2: Write the test case to call the function and cover the else condition by giving the age less than 18. This covers the else block statements i.e., it covers the conditional statements.
- Test Case 3: Write the test case to print the age. This covers the unconditional statement.
All the above 3 test cases cover all possible scenarios and coverages.
Running Unit Tests
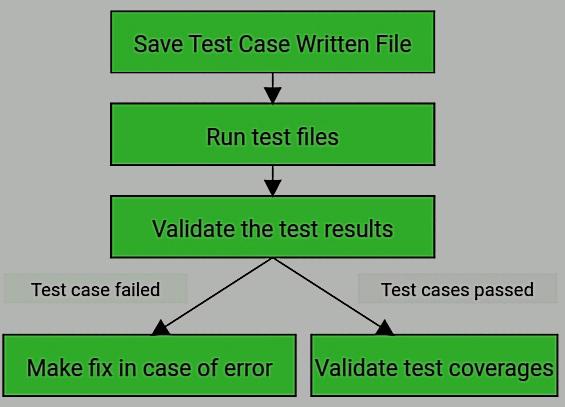
Once you are done with your test cases then now it’s time to execute those test cases. The following steps need to be followed. The test cases can be executed by using any of the test frameworks or you can also run each test case that you want to execute.
Step 1: Save Test Cases Written File
Once test cases are written with different scenarios, save the file to run.
Step 2: Run Test Files
Now, use the command to run all files or particular files or run each test case to see the result.
Step 3: Validate the Test Results
Once the test cases are running successfully, it will display the result with how many test cases are passed and how many test cases are failed. If test cases are not running successfully, then it will show where is the syntax error or other error in that file to correct it. If test cases are passed then it will show the coverage details.
Analyzing Test Results
Test result analysis is an important process to carry out. With the help of this analysis, we can increase the efficiency of the unit testing and it helps in increasing the overall test coverage. Let’s see some of the ways to analyze the test results to help with unit testing efficiency.
- Once test cases are running successfully, it will show the result like several test cases passed or failed.
- Analyze the failed test cases and find the solution for that. It will show where and why the test cases are failing.
- If the coverage is in green color of any of the coverage or any other indications then we are good with that.
- It will also show if there is any difference between expected and received results. From this, you can decide what we have to pass in the expected input to get the required output.
- We can see how many uncovered lines are there to cover in the source code file. You can write the test cases for those pending uncovered lines to increase the coverage.
- We can see the % coverages of each coverage (function, branch, block, and condition). With the help of this, we can work to increase the coverage.
- If the coverage is denoted in red color of any of the coverages or any other indications then we need to take action to increase the coverage.
- If the coverage is in the yellow color of any of the coverages or any other indications then additional efforts are required to cover.
Test Coverages and Code Quality
Coverages in Unit Testing
There are different coverages in Unit testing. They are Function coverages, Branch/Decision coverages, Block/Statement coverages, and Condition Coverages. Coverages are calculated in the form of percentages and it ensures how many codes have been covered.
1. Function Coverage: It ensures that how many methods or functions are covered by executing the test cases. The percentage can be calculated by using (Number of functions covered in the source file ) / (Total Number of functions in the source file) * 100.
2. Branch/Decision Coverage: It ensures that how many branches(both conditional and unconditional branches) are covered by executing the test cases. The percentage can be calculated by using (Number of branches covered in the source file ) / (Total Number of branches in the source rce file) * 100
3. Block/Statement Coverages: It ensures how many statements are covered by executing the test cases. The percentage can be calculated by using (Number of statements covered in the source file ) / (Total Number of statements in the source file) * 100
4. Condition Coverages: It ensures how many conditional expressions are covered by executing the test cases. The percentage can be calculated by using (Number of conditions covered in the source file ) / (Total Number of conditions in the source file) * 100.
It is good to have more than 80% of the overall coverage for the application.
Code Quality
The overall test coverage is the combined percentage coverage of how many functions, branches, statements, and conditions are covered.
- If the overall test coverage of all files is more than 70% and less than 80% then it is neutral but additional test cases are needed to cover the source code.
- If the overall test coverage of all files is more than 80% and less than 90% then it is good to proceed for deployment. The code quality seems to be good.
- If the overall test coverage of all files is more than 90% then it is highly efficient to go for deployment and code quality proves its efficiency.
- If the overall test coverage of all files is less than 70% then additional efforts are needed to modify or update the test cases to cover it more than 80%. In this case, we can’t proceed with code deployment to servers.
Best Practices for Unit Testing
Unit testing requires an efficient approach to writing and covering the test cases. Let us see some of the best practices for unit testing.
- Cover All Test Scenarios: The main goal is to cover the test cases for all or most of the scenarios in the source code.
- Less Test Case, More Coverage: It aims to write a minimum number of test cases to cover a maximum number of test scenarios to increase the coverage. For this, we require good source code and test case writing knowledge.Â
- Avoid Additional Data: Try to avoid creating the additional mock data file unnecessarily to cover or increase the test coverages. Because we need to deploy those mock data to the serves as well.
- Test Documentation: Prepare the test plans with proper documentation in advance which will be helpful while writing the test cases.
- Efficient Test Cases: Efficiency in Unit test coding is required. Write efficient test cases which require a minimal amount of time to execute the test results.
- 80% Coverage: More than 80% coverage is good to have for all test files. It is best to have more than 80% in all types of coverage.
- Test Case Readability: Maintain the test case code readability for test case writing which will be helpful for other developers to track the test cases.
- Relevant Test Names: Give relevant test case file names with relevant test information in the test plan documentation. Also, write the test cases with relevant information in the test code as well.
Common Unit Testing Frameworks
Unit Testing Frameworks are needed to run the unit tests. Let’s see some common unit testing frameworks.
- JUnit: It is an open-source framework that is used to provide test runners and execute the accurate results of each test.
- Jest: It is a testing framework that is used for frontend languages to run the test cases. It is used with JavaScript frameworks.
- CyPress: It is a frontend testing framework that is used to provide test results with snapshots. It has many built-in packages which is helpful for testing.
- PyUnit: It is one of the most commonly used test frameworks for Python. Developers use this framework as the default one to test. It consists of in-built Python test libraries to handle the test scenarios.
- XUnit: It is an open-source testing framework that is used for .Net frameworks to write and execute the test cases.
Conclusion
From the above article, we can learn to set up the test environment for unit testing and we can run and analyze the results. You can also follow the best practices mentioned above to run the unit test cases. According to your source code language, you can install the framework tool that you need.
Share your thoughts in the comments
Please Login to comment...