How to display HTML tags as plain text using PHP
Last Updated :
28 Nov, 2018
HTML tags begin with the less-than character and end with greater-than character, the text inside the tag formatted and presented according to the tag used. Every tag has special meaning to the browser but there are cases when to show plain HTML code in webpage.
There are various methods in PHP to show the HTML tags as plain text, some of them are discussed below:
Method 1: Using htmlspecialchars() function: The htmlspecialchars() function is an inbuilt function in PHP which is used to convert all predefined characters to HTML entities.
Syntax:
string htmlspecialchars( $string, $flags, $encoding, $double_encode )
- $string: This parameter is used to hold the input string.
- $flags: This parameter is used to hold the flags. It is combination of one or two flags, which tells how to handle quotes.
- $encoding: It is an optional argument which specifies the encoding which is used when characters are converted. If encoding is not given then it is converted according to PHP default version.
- $double_encode: If double_encode is turned off then PHP will not encode existing HTML entities. The default is to convert everything.
Return Values: This function returns the converted string. If there is invalid input string then empty string will returned.
Example:
<?php
echo ( "<b>without using htmlspecialchars() function</b><br>" );
$myVar = htmlspecialchars("<b>using htmlspecialchars()
function </b>", ENT_QUOTES);
echo ( $myVar );
?>
|
Output:
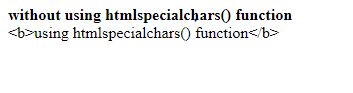
Method 2: Using htmlentities() function: The htmlentities() function is an inbuilt function in PHP which is used to transform all characters which are applicable to HTML entities. This function converts all characters that are applicable to HTML entity.
Syntax:
string htmlentities( $string, $flags, $encoding, $double_encode )
Parameters: This function accepts four parameters as mentioned above and described below:
- $string: This parameter is used to hold the input string.
- $flags: This parameter is used to hold the flags. It is combination of one or two flags, which tells how to handle quotes.
- encoding: It is an optional argument which specifies the encoding which is used when characters are converted. If encoding is not given then it is converted according to PHP default version.
- $double_encode: If double_encode is turned off then PHP will not encode existing HTML entities. The default is to convert everything.
Return Values: This function returns the string which has been encoded.
Example:
<?php
$str = "<b>GeeksforGeeks</b>" ;
echo ( "without using htmlentities() function = " . $str . "<br>" );
$myVar = htmlentities( $str , ENT_QUOTES);
echo ( "with using htmlentities() function = " . $myVar );
?>
|
Output:

Method 3: This method is used to replace the character by set of characters to get the desired output. In this method, < is replaced by < and > is replaced by >.
Example:
<?php
$str = "<b>GeeksforGeeks</b>" ;
echo ( "without using & lt; and & gt; = " . $str . "<br>" );
$myVar = "<b>GeeksforGeeks</b>" ;
echo ( "with using & lt; and & gt; = " . $myVar );
?>
|
Output:
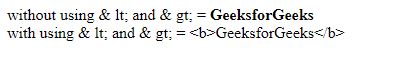
Share your thoughts in the comments
Please Login to comment...